Overcoming Module Conflicts in Java 9's Project Jigsaw
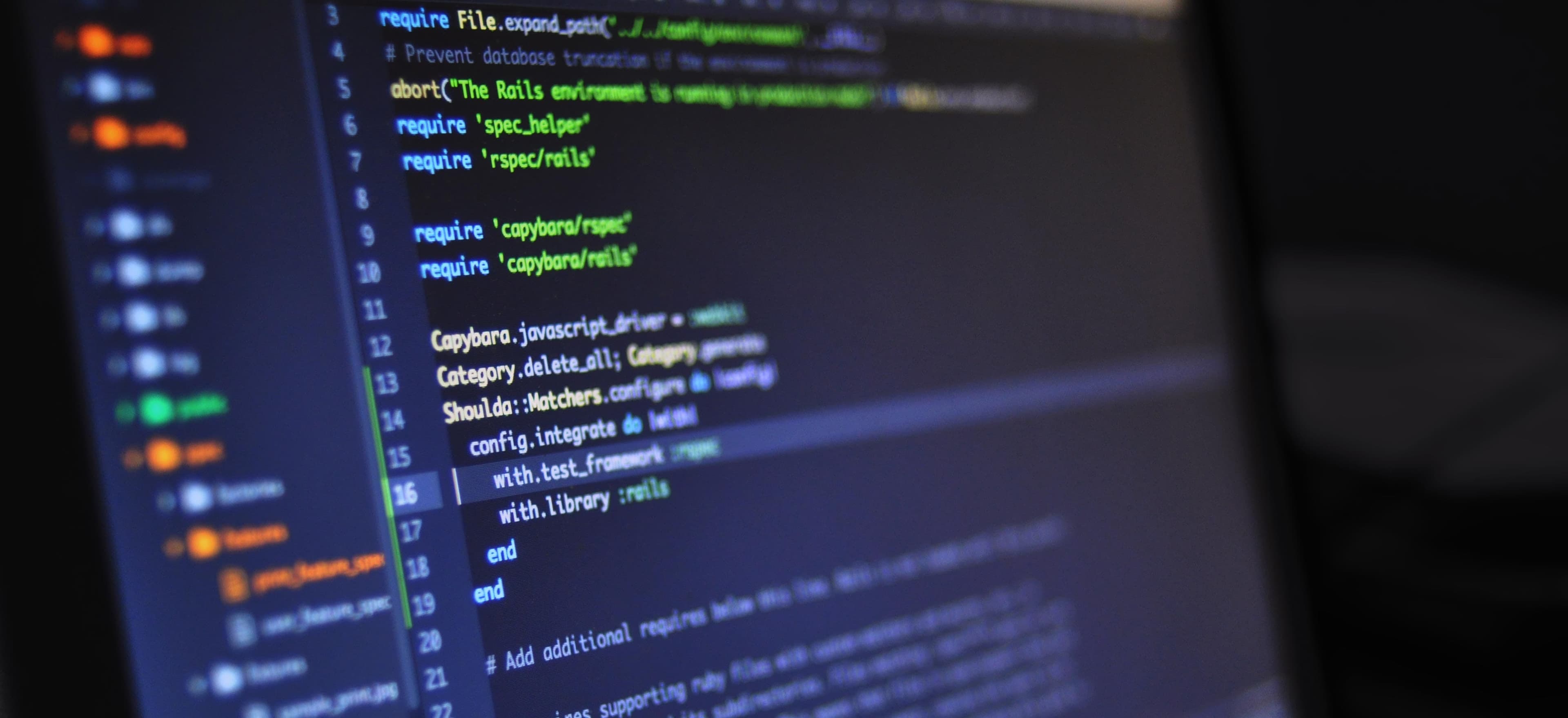
- Published on
Overcoming Module Conflicts in Java 9's Project Jigsaw
Java 9 introduced a revolutionary way to organize code known as Project Jigsaw. This project aimed to modularize the Java platform itself, giving developers a powerful way to create applications that are not only more maintainable but also more performant. However, along with its benefits, it also introduced certain challenges, most notably module conflicts. In this blog post, we'll delve into understanding these conflicts and how to overcome them effectively.
Understanding Project Jigsaw
Before diving into the intricacies of module conflicts, it's essential to grasp what Project Jigsaw is all about. Essentially, Jigsaw allows developers to define modules—collections of packages that encapsulate their public APIs and internal implementation. This initiative aims to improve the scalability of Java applications, making the codebase cleaner and more organized.
Modules are defined in a module-info.java
file, creating a clear contract on what the module exports and what it requires from other modules. Here is a simple example:
module com.example.myapp {
exports com.example.myapp.api;
requires com.example.externalmodule;
}
In this example, the module com.example.myapp
requires another module called com.example.externalmodule
while exposing its API defined in the com.example.myapp.api
package.
The Problem - Module Conflicts
Despite its advantages, one of the significant challenges developers encounter with Project Jigsaw is module conflicts. These conflicts arise when different modules attempt to provide the same package, leading to ambiguity in which implementation should be used.
Common Scenarios Leading to Module Conflicts:
- Duplicate Packages: Two modules exporting the same package.
- Transitive Dependencies: A module depends on another module that itself has dependencies, leading to multiple versions of the same package being loaded.
- Legacy Libraries: Many existing Java libraries were not designed with Jigsaw's module system in mind, thus complicating the migration process.
Example of a Module Conflict
Imagine you have two external libraries, libA
and libB
, both exporting a package called com.example.utils
. If both libraries are included in your module, it will lead to a compilation or runtime error due to duplicate packages. Let's look at a code snippet demonstrating this scenario.
module com.example.app {
requires libA;
requires libB; // Both libA and libB export com.example.utils
}
Running this code will throw an error indicating the package com.example.utils
is already provided by another module.
Strategies to Overcome Module Conflicts
1. Modularize Legacy Code
If you are working with legacy codebases, consider creating a custom module structure. Isolate your legacy code into a separate module and wrap it with proper module definitions. This approach can help reduce direct package conflicts.
module com.example.legacy {
exports com.example.legacy.utils; // Correctly isolates the legacy code
}
2. Use Automatic Modules
Java allows the use of automatic modules, which do not have a module-info.class
but can still be used in a module path. You can define the name of the automatic module via the JAR file name. This is particularly useful for integrating libraries that haven’t been modularized yet.
jar --create --file=libA.jar --main-class=com.example.Main --manifest MANIFEST.MF -C out/ .
The MANIFEST.MF will define the automatic module name as follows:
Automatic-Module-Name: libA
3. Eliminate Transitive Dependencies
If your modules are bringing in conflicting libraries via transitive dependencies, check your dependencies carefully. You can explicitly require only essential libraries in your module-info.java
.
For example, if library C
depends on both A
and B
, and you note that both A
and B
conflict, you can do:
module com.example.app {
requires A;
requires B; // Opt for the necessary library only
}
This way, you gain greater control over what is included in your module, reducing potential conflicts.
4. Use the --patch-module
Option
The --patch-module
option allows you to override certain classes in a module at runtime. This approach can be used to replace a conflicting class with a tailored one, ensuring that the correct implementation is loaded.
java --patch-module com.example.module=overriden/classes com.example.Main
5. Consult Module System Documentation
Refer to the official Java documentation on the module system for insights and in-depth explanations. Understanding the specifications can help you apply best practices when configuring your modules.
Best Practices for Managing Module Conflicts
- Use Unique Package Names: Always ensure that your packages have a unique namespace, especially when developing libraries that might be used by other developers.
- Maintain Dependency Hygiene: Regularly audit your modules and their dependencies to avoid unnecessary complexity.
- Version Control: Manage versions of your dependencies carefully. Use tools like Maven or Gradle to enforce version constraints, thereby reducing the risk of conflicts.
Final Thoughts
Java 9’s Project Jigsaw offers a robust modular system for improved application design, but it’s not without its challenges. Module conflicts can pose significant issues in application development, especially when dealing with legacy libraries or complex dependencies. By employing the strategies and best practices outlined here, you can effectively manage and overcome these conflicts, leading to cleaner, more maintainable code.
For further reading on Java's module system and to deepen your understanding, visit the official documentation at Java Platform Module System.
Happy coding!
Checkout our other articles