Common Pitfalls When Using Java 8 Lambda Expressions
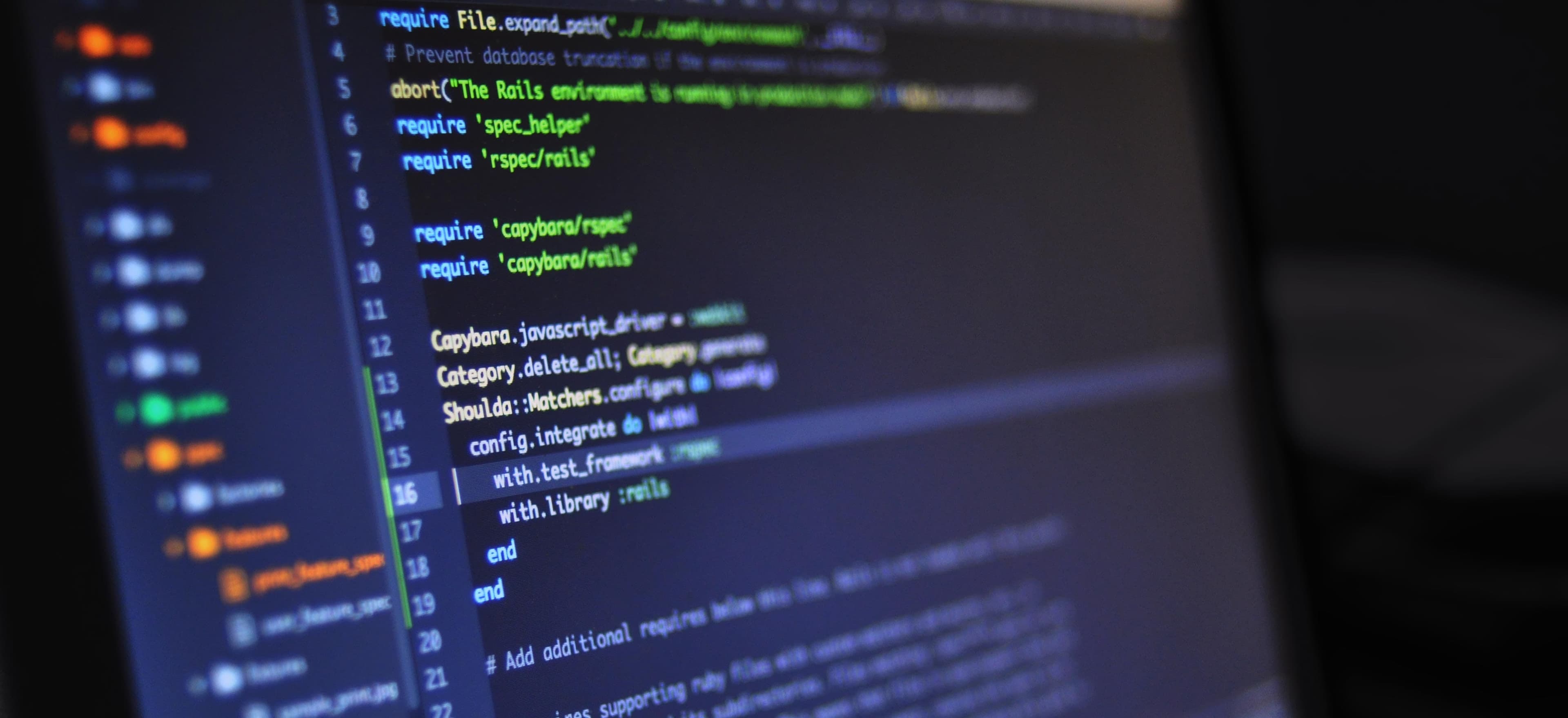
- Published on
Common Pitfalls When Using Java 8 Lambda Expressions
Java 8 has introduced a significant evolution to the language through the addition of Lambda expressions. These concise blocks of code enhance the expressiveness and readability of your Java programs. However, mastering Lambdas is not without its challenges. This post will cover some common pitfalls developers may encounter when using Java 8 Lambda expressions, providing illustrative code snippets to help clarify the discussion.
Understanding Lambda Expressions
At their core, Lambda expressions provide a way to implement functional interfaces, which are interfaces that contain a single abstract method. Here is a simple example of a Lambda expression in action:
Runnable runnable = () -> System.out.println("Hello, Java 8!");
In this example, the Runnable
interface is implemented using a Lambda expression, reducing the amount of boilerplate code needed compared to using an anonymous class.
Why Use Lambda Expressions?
Using Lambda expressions enhances code clarity and succinctness. It also aligns with the functional programming paradigm, allowing for cleaner, more modular code. However, as developers begin to implement Lambdas, they may encounter several pitfalls.
1. Variable Scope Issues
One common pitfall is misunderstanding variable scope. In Java, local variables used in Lambda expressions must be effectively final.
Example of Variable Scope Issue
Consider the following code:
public class VariableScopeExample {
public static void main(String[] args) {
int outerVariable = 10;
Runnable runnable = () -> {
// outerVariable++; // This will cause a compilation error
System.out.println(outerVariable);
};
runnable.run();
}
}
Commentary
In this code, attempting to modify outerVariable
within the Lambda results in a compilation error. The Java compiler allows only effectively final variables in Lambda expressions, ensuring predictable behavior. This restriction is primarily designed to prevent inconsistencies in multi-threaded scenarios.
2. Overusing Lambda Expressions
It’s tempting to convert all your code to use Lambda expressions, but this can lead to over-engineered solutions. Sometimes, traditional loops or regular methods can be clearer and easier to understand.
Example of Overuse
import java.util.Arrays;
import java.util.List;
public class OverusingLambdaExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Unnecessarily complex usage of lambdas
numbers.forEach(n -> {
if (n % 2 == 0) {
System.out.println(n);
}
});
// More concise option
numbers.stream()
.filter(n -> n % 2 == 0)
.forEach(System.out::println);
}
}
Commentary
In the above example, using Lambdas led to an overly complex solution for a simple task. While the use of Streams (a more complex use of Lambdas) is powerful, if the method is only making small alterations to simple loops, consider using simple loops for the sake of readability.
3. Exception Handling
Handling exceptions in Lambda expressions is another area where developers often stumble. Unlike traditional methods, you cannot use checked exceptions in Lambda expressions easily.
Example of Exception Handling
import java.util.Arrays;
import java.util.List;
public class ExceptionHandlingExample {
public static void main(String[] args) {
List<String> strings = Arrays.asList("1", "2", "three", "4");
strings.forEach(s -> {
try {
int number = Integer.parseInt(s);
System.out.println(number);
} catch (NumberFormatException e) {
System.err.println("Invalid number: " + s);
}
});
}
}
Commentary
In this example, the Lambda expression includes a try-catch block to handle exceptions. Omitting such error handling can lead to issues if unexpected values are encountered, undermining the robustness of your application.
4. Performance Implications
While Lambdas can make your code cleaner, they can also introduce performance implications, especially in environments where instance creation is costly. Every time a Lambda is invoked, a new instance of the encapsulating class is created.
Example of Performance Impact
import java.util.stream.IntStream;
public class PerformanceExample {
public static void main(String[] args) {
int sum = IntStream.range(1, 1000000)
.map(i -> {
// Inefficient use of Lambda
return i * 2;
})
.sum();
System.out.println("Sum: " + sum);
}
}
Commentary
In the above code, the individual mapping for every integer could have been optimized using method references, reducing the overhead associated with Lambda expression invocations.
5. Infinite Recursion in Lambdas
Another less common but critical pitfall is infinite recursion when using Lambdas. This can occur if a Lambda expression inadvertently references itself.
Example of Infinite Recursion
import java.util.function.Supplier;
public class InfiniteRecursionExample {
public static void main(String[] args) {
Supplier<String> infiniteSupplier = () -> infiniteSupplier.get(); // Recursion without termination
// This will lead to a StackOverflowError
System.out.println(infiniteSupplier.get());
}
}
Commentary
In this scenario, the Lambda expression continues to call itself indefinitely, resulting in a StackOverflowError
. Always ensure that Lambda expressions have clear exit conditions.
Closing the Chapter
Java 8 Lambda expressions offer a powerful addition to the Java programming language, unleashing a new level of code flexibility and expressiveness. However, it's crucial to be mindful of the pitfalls mentioned in this post.
Understanding variable scope, avoiding excessive use of Lambdas, managing exceptions effectively, recognizing performance implications, and preventing infinite recursive calls are vital to leveraging Lambdas effectively.
Additionally, consider the Java 8 documentation for further reading on Lambda expressions.
By adhering to best practices and being aware of these common pitfalls, Java developers can harness the full potential of Lambda expressions, making their code not only concise and elegant but also robust and maintainable.
If you want to dive deeper into Java 8 streams and their power, explore this tutorial on Java Streams for more examples and insights.
Happy coding!