Understanding JVM Memory Leaks: Common Causes and Fixes
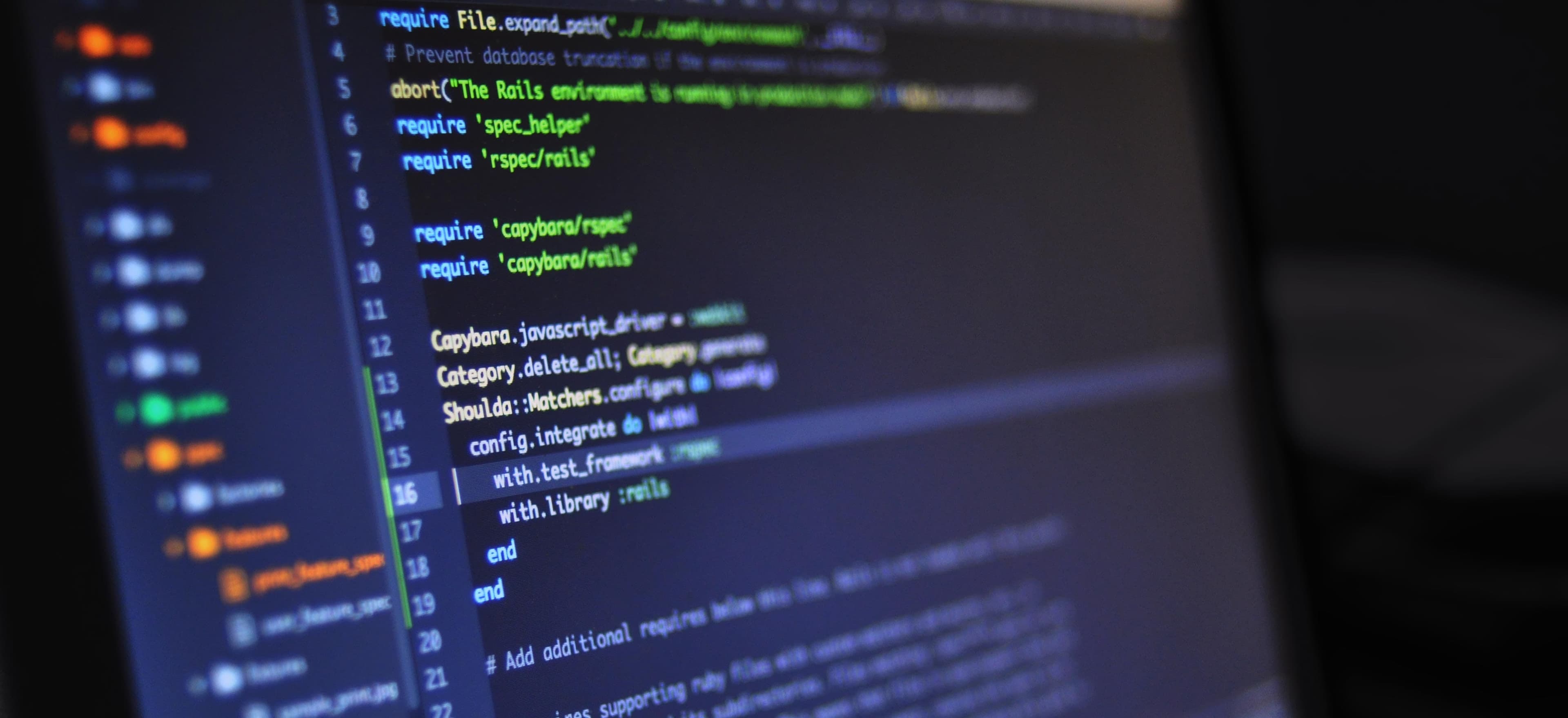
- Published on
Understanding JVM Memory Leaks: Common Causes and Fixes
Java Virtual Machine (JVM) is the backbone of Java applications, enabling us to run code on various platforms. However, one of the significant challenges faced by developers is memory leaks. Memory leaks can severely impact application performance, leading to system slowdowns, crashes, or excessive garbage collection pauses. In this blog post, we'll explore common causes of memory leaks in the JVM, how to detect them, and effective strategies to fix them.
What is a Memory Leak?
A memory leak occurs in a program when it allocates memory but fails to release it back to the operating system. In the context of Java and the JVM, this often means that objects are referenced but no longer needed, preventing the garbage collector from reclaiming their memory. Consequently, the application continues to use more memory over time, leading to eventual performance issues.
Common Causes of Memory Leaks
-
Static Collections
Static collections (like HashMaps or ArrayLists) retain references to their elements indefinitely. If objects are added to these collections and are never removed, they cannot be garbage collected.
public class MemoryLeakExample { private static final List<String> staticCollection = new ArrayList<>(); public static void addToCollection(String value) { staticCollection.add(value); } }
Why: The
staticCollection
retains references to all added values, preventing garbage collection. Since it is static, it persists until the class is unloaded. -
Inner Classes
Non-static inner classes hold an implicit reference to their outer class. If the inner class is long-lived (like an event listener), it creates an unintentional memory leak if the outer class is no longer needed.
public class Outer { private String name = "Outer Class"; class Inner { public void displayName() { System.out.println(name); } } }
Why: The
Inner
class maintains a reference toOuter
, and ifOuter
has a longer lifecycle thanInner
, it can lead to memory leaks. -
Event Listeners
Adding listeners to UI components without removing them can lead to memory leaks. If the source component is destroyed, the listener often remains referenced.
public class MemoryLeakEvent { public MemoryLeakEvent(Button button) { button.addActionListener(e -> System.out.println("Button clicked!")); } }
Why: The listener holds a reference to its enclosing instance, which keeps the instance from being garbage collected after the button is no longer used.
-
Thread Local Variables
Using
ThreadLocal
can create memory leaks if not handled correctly. If the variable is never removed, it can accumulate memory within the thread it was created in.public class ThreadLocalExample { private static final ThreadLocal<String> threadLocalValue = ThreadLocal.withInitial(() -> "Initial Value"); public String getThreadLocalValue() { return threadLocalValue.get(); } }
Why: If a thread that uses
ThreadLocal
is not terminated or cleaned up, it holds references that can accumulate and lead to leaks.
Detecting Memory Leaks
Detecting memory leaks requires careful analysis and monitoring. Here are a few approaches:
1. Profiling Tools
Tools like VisualVM and Eclipse Memory Analyzer can visualize memory usage within your application. These tools provide heap dumps that you can analyze to identify memory leaks by looking for objects that are retained longer than expected.
2. Garbage Collection Logging
Enabling garbage collection (GC) logging provides insights into the behavior of the garbage collector. You can enable GC logging with the following JVM options:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -Xloggc:gc.log
Why: This allows you to see how frequently garbage collections occur and the memory consumption over time, helping to correlate performance issues with potential leaks.
3. Automated Leak Detection Libraries
Libraries such as LeakCanary can automatically detect memory leaks in Android applications and provide detailed stack traces for analysis.
Fixing Memory Leaks
Once identified, fixing memory leaks can vary depending on the underlying cause. Here are some effective strategies:
1. Use Weak References
For collections intended to cache objects without preventing their garbage collection, consider using WeakReference
.
public class Cache {
private final Map<String, WeakReference<Object>> cache = new HashMap<>();
public void add(String key, Object value) {
cache.put(key, new WeakReference<>(value));
}
public Object get(String key) {
WeakReference<Object> ref = cache.get(key);
return ref != null ? ref.get() : null;
}
}
Why: This ensures that if a strong reference to the object does not exist elsewhere, it can be garbage collected.
2. Remove Unused Listeners
Always ensure you unsubscribe event listeners when they are no longer needed. For instance, if you're using anonymous inner classes or lambda expressions, consider explicitly removing them.
public class EventSource {
private final List<ActionListener> listeners = new ArrayList<>();
public void addActionListener(ActionListener listener) {
listeners.add(listener);
}
public void removeActionListener(ActionListener listener) {
listeners.remove(listener);
}
}
Why: This allows the garbage collector to reclaim memory for the enclosing objects, thus mitigating leaks.
3. Manage Inner Class References
Consider using static inner classes or moving the inner class to a top-level class to avoid implicit references to the outer class.
public class Outer {
private static class StaticInner {
public void doSomething() {
// No reference to Outer class
}
}
}
Why: This prevents the inner class from unintentionally retaining the outer class's reference, facilitating garbage collection.
4. Analyze Thread Local Usage
Be disciplined with ThreadLocal
by clearing values when they are no longer needed.
public class ThreadLocalExample {
private static final ThreadLocal<String> threadLocalValue = new ThreadLocal<>();
public void setValue(String value) {
threadLocalValue.set(value);
}
public void clearValue() {
threadLocalValue.remove();
}
}
Why: This reduces the risk of memory leaks by making sure the thread-local data does not persist beyond its useful life.
Lessons Learned
Memory leaks can be detrimental to Java applications, impacting performance and reliability. By understanding common causes—such as static collections, inner classes, event listeners, and improper use of ThreadLocal
—you can adopt strategies to detect and prevent leaks.
Regularly profiling your application, managing references carefully, and utilizing tools available for monitoring can save time and effort in the long run. With proper understanding and careful programming practices, you can maintain robust and efficient Java applications free of memory leaks.
For further reading, consider checking out JVM Memory Management for a deeper understanding of how the JVM manages memory.
Happy coding!