Common Pitfalls of Synchronization in Java Multithreading
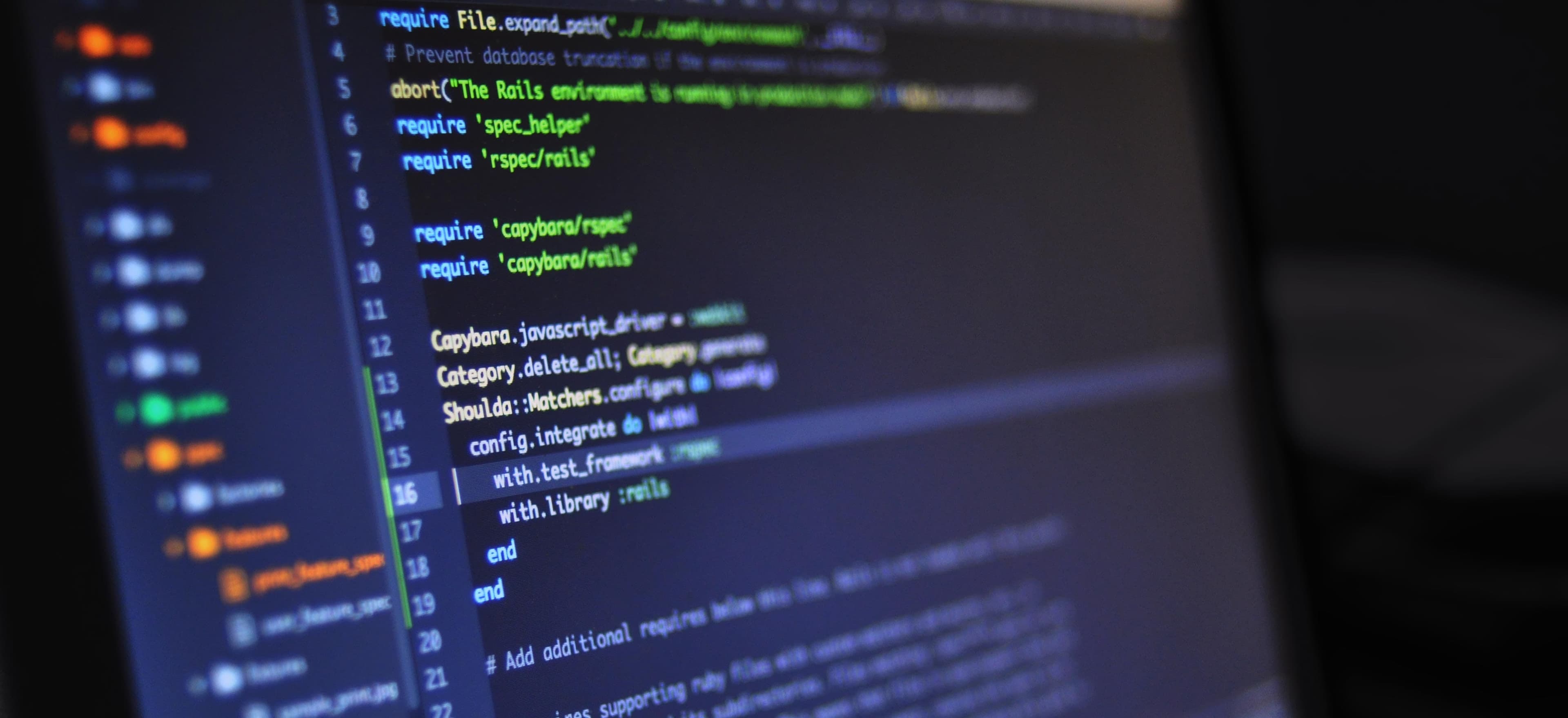
- Published on
Common Pitfalls of Synchronization in Java Multithreading
Multithreading is an essential feature in Java, allowing developers to execute multiple threads simultaneously for improved application performance. However, with this powerful feature comes the responsibility of managing thread synchronization to avoid common pitfalls. In this post, we'll explore some of the most frequent mistakes made when using synchronization in Java, providing code examples along the way.
Understanding Synchronization
Before diving into the pitfalls, it's crucial to understand what synchronization is. In Java, synchronization is used to control access to shared resources by multiple threads. The main goal is to prevent thread interference and memory consistency errors.
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In this simple Counter
class, we have a synchronized increment
method that ensures that only one thread can modify the value of count
at a time. This is our first step toward thread safety.
Pitfall 1: Over-synchronization
One of the most common pitfalls is over-synchronizing, which can lead to contention and reduced performance. When too many blocks of code are synchronized unnecessarily, it can bottleneck application performance. Here’s an incorrect approach:
public class UnsafeCounter {
private int count = 0;
public synchronized void increment() {
// Overhead of synchronization for simple increment
count++;
}
public synchronized int getCount() {
// Overhead of synchronization for reading
return count;
}
public void performComplexOperation() {
// Complex logic here that doesn't require synchronization
// ...
synchronized (this) {
// They could be synchronized here, but aren't necessary for the increment logic
increment();
}
}
}
Best Practice
Only synchronize the code that must be synchronized. In the above example, increment
and getCount
may not need synchronization if the method is part of a larger synchronized block. The performComplexOperation
method might need synchronization, but the actual increment operation does not need to be synchronized if it is already encapsulated properly.
Pitfall 2: Deadlock
Deadlock occurs when two or more threads are waiting for each other to release resources, resulting in a standstill. This can happen if locking order matters. Consider the following example:
public class DeadlockExample {
private final Object resource1 = new Object();
private final Object resource2 = new Object();
public void method1() {
synchronized (resource1) {
synchronized (resource2) {
// Work with both resources
}
}
}
public void method2() {
synchronized (resource2) {
synchronized (resource1) {
// Work with both resources
}
}
}
}
In the above scenario, method1
locks resource1
and waits for resource2
, while method2
locks resource2
and waits for resource1
, leading to deadlock.
Best Practice
To avoid deadlock, ensure that all threads acquire locks in a consistent order and consider using try-lock mechanisms or timed locks using java.util.concurrent.locks.Lock
.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ImprovedDeadlock {
private final Lock lock1 = new ReentrantLock();
private final Lock lock2 = new ReentrantLock();
public void method1() {
lock1.lock();
try {
if (lock2.tryLock()) {
try {
// Work with both resources
} finally {
lock2.unlock();
}
}
} finally {
lock1.unlock();
}
}
public void method2() {
lock2.lock();
try {
if (lock1.tryLock()) {
try {
// Work with both resources
} finally {
lock1.unlock();
}
}
} finally {
lock2.unlock();
}
}
}
Pitfall 3: Lost Wakeup
Lost wakeup occurs when a thread that was waiting on a condition is signaled to wake up, but it misses the wake-up notification due to timing issues. This scenario is most commonly encountered with the wait
and notify
methods.
public class LostWakeupExample {
private final Object lock = new Object();
public void produce() {
synchronized (lock) {
// Producing and notifying
lock.notify();
}
}
public void consume() {
synchronized (lock) {
try {
lock.wait(); // Waiting often misses the signal!
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
// Consuming logic here
}
}
}
If produce
is called after consume
has entered the waiting state, but before it has called wait()
, it can miss the notification.
Best Practice
Ensure that you use a loop to check the condition after waiting. Here’s an updated example:
public class ImprovedLostWakeup {
private final Object lock = new Object();
private boolean available = false;
public void produce() {
synchronized (lock) {
available = true;
lock.notify();
}
}
public void consume() {
synchronized (lock) {
while (!available) {
try {
lock.wait();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
// Consuming logic here
available = false;
}
}
}
Resources for Further Reading
- For a more detailed understanding of multithreading in Java, you can check the Java Concurrency in Practice book.
- The official Java documentation on concurrency is also an excellent resource.
Lessons Learned
When working with synchronization in Java, it’s essential to recognize and avoid common pitfalls like over-synchronization, deadlock, and lost wakeup. By following best practices, developers can ensure thread-safe applications while maintaining performance. Mastering synchronization is a critical step for any developer seeking to work effectively in a multithreaded environment.
In the world of Java, being aware of these pitfalls can save you a tremendous amount of debugging time and enhance your applications' robustness. Remember, while synchronization helps ensure thread safety, it must be applied judiciously.
Take the time to reflect on your code and be aware of these pitfalls as you advance in your Java multithreading journey.
Checkout our other articles