Mastering Java Generics: Common Pitfalls and How to Avoid Them
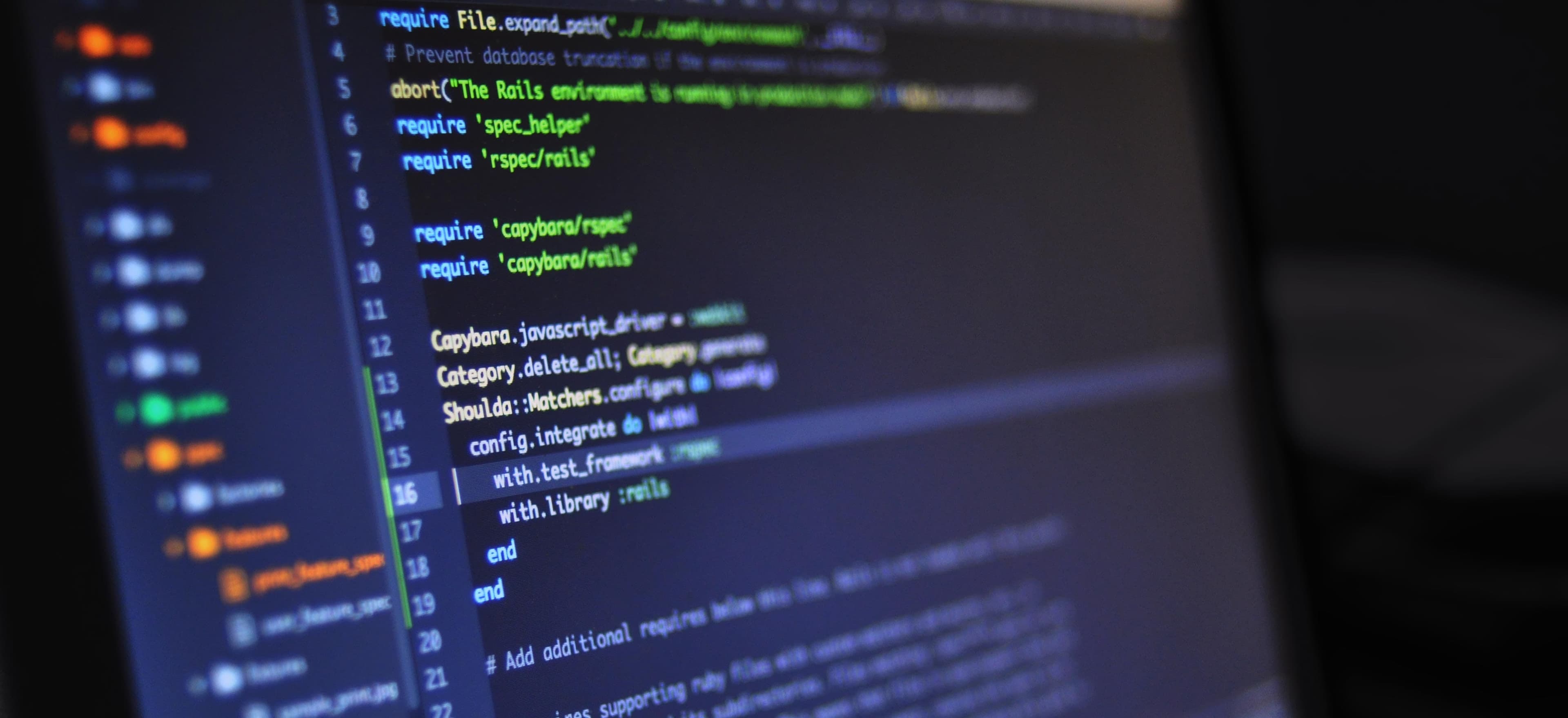
- Published on
Mastering Java Generics: Common Pitfalls and How to Avoid Them
Java Generics is a powerful feature that allows developers to create more flexible, reusable, and type-safe code. Despite its many benefits, developers often face challenges when working with generics. Understanding common pitfalls and learning how to avoid them is crucial for mastering this aspect of Java programming. This blog post will explore these issues, provide code snippets for clarity, and guide you toward better practices in your Java projects.
What Are Java Generics?
Java Generics enable types (classes and interfaces) to be parameters when defining classes, interfaces, and methods. The primary goal is to provide stronger type checks at compile-time, which reduces the risk of ClassCastException
at runtime.
Example of Generics
Consider a simple generic class:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Here, T
is a type parameter that can accept any non-primitive type. This allows for a clean and reusable implementation of the Box
class that can hold various types of objects.
Common Pitfalls in Java Generics
While generics provide significant advantages, they are not without their issues. Let's discuss some common pitfalls, starting with wildcards, followed by bounds, and then type erasure.
1. Wildcard Confusion
Java provides wildcards (represented as ?
) to deal with unknown types. Here's the common mistake:
public void addItem(List<?> list) {
list.add(new String("Hello")); // Compilation Error
}
Why This is a Problem
The wildcard ?
indicates an unknown type. As a result, adding any specific type is not allowed because the compiler cannot ensure type safety.
How to Avoid This
Utilize bounded wildcards when you need to accept or produce a certain type:
public void addItem(List<? super String> list) {
list.add(new String("Hello")); // This works!
}
Here, ? super String
allows you to add a String
to any list that contains String
or its superclasses, such as Object
.
2. Using Raw Types
Raw types refer to generic classes without specifying a type parameter. This can lead to unsafe operations:
List rawList = new ArrayList(); // Raw type
rawList.add("Hello");
rawList.add(10); // Mixing types
Why Avoid Raw Types?
Using raw types removes type safety checks. Accessing elements from rawList
may lead to ClassCastException
:
String item = (String) rawList.get(0); // This is safe
String anotherItem = (String) rawList.get(1); // This will throw an exception
How to Avoid This
Always specify type parameters when working with generics:
List<String> list = new ArrayList<>();
list.add("Hello"); // Now it's safe
3. Inheritance and Generics
Another common pitfall is assuming that type parameters in generics have a natural inheritance relationship. Consider the following scenario:
List<Number> numberList = new ArrayList<Integer>(); // Compilation Error
Why This is a Problem
Generics are invariant. List<Number>
is not a supertype or subtype of List<Integer>
. This means you can't substitute between different parameterized types, even if they are closely related.
How to Avoid This
Use wildcards appropriately:
List<? extends Number> numberList = new ArrayList<Integer>();
This syntax indicates that numberList
can hold a list of any type that extends Number
, preserving the type safety of your collections.
4. Type Erasure
One of the less discussed aspects of generics is type erasure, which removes type information from generics at runtime. This can impact functionality in some cases:
public class Pair<T> {
private T first;
private T second;
public void setFirst(T first) {
this.first = first;
}
public void setSecond(T second) {
this.second = second;
}
}
// Fails at runtime
Pair<String> stringPair = new Pair<>();
Pair<Integer> intPair = new Pair<>();
if (stringPair.getClass() == intPair.getClass()) { // This returns true
// Confusing behavior
}
Why This is a Problem
As all generics are erased into their bounds (or Object
for unbounded types), the type information system cannot distinguish between different instances of the same generic class.
How to Avoid This
Design interfaces and methods such that they don't rely on type information at runtime. Use type tokens (like Class<T>
) to pass type information explicitly when needed.
public class Pair<T> {
private T first;
private T second;
public Pair(Class<T> clazz) { // Use Class as a type token
this.clazz = clazz;
}
}
Wrapping Up
Java Generics can significantly enhance the type safety and reusability of your code. However, as we've discussed, several pitfalls can lead to unsafe operations or extra complexity. By understanding wildcards, avoiding raw types, managing inheritance appropriately, and being aware of type erasure, you can master generics and leverage their power more confidently.
For further reading, consider exploring Oracle's Java Generics documentation and consulting Java Language Specification for a deeper dive into generics and their implementation.
By staying informed of these common pitfalls and aligning your coding practices accordingly, you can write safer and more robust Java code that takes full advantage of generics. Happy coding!
Checkout our other articles