The Key Differences Between Java Interfaces and Abstract Classes
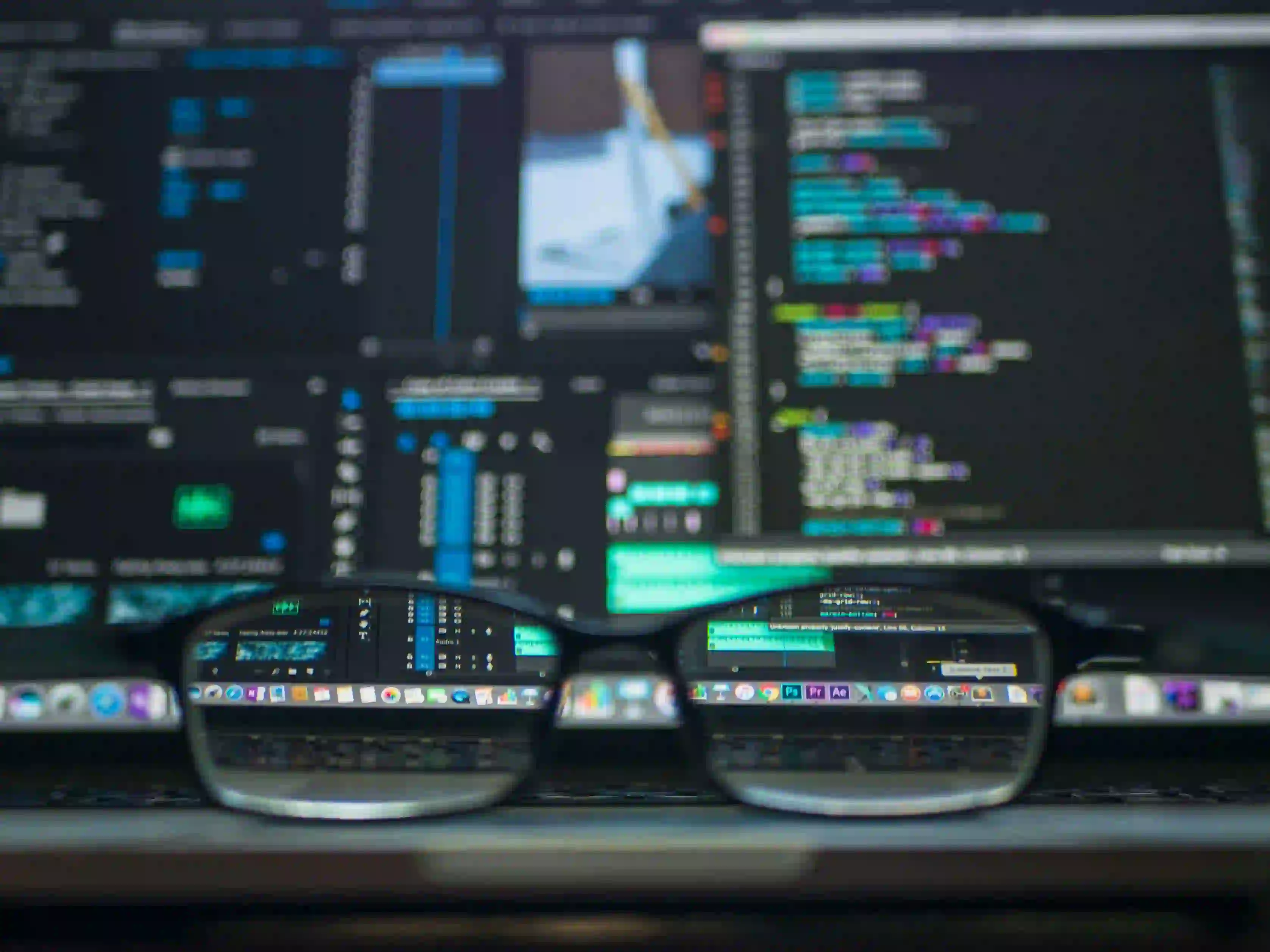
The Key Differences Between Java Interfaces and Abstract Classes
When developing applications in Java, you often need to design classes that share common behavior while still allowing for flexibility and extensibility. Two essential features of Java that help achieve this are interfaces and abstract classes. However, understanding when to use one over the other can be a daunting task for developers, especially those new to Java. In this blog post, we'll explore the key differences between Java interfaces and abstract classes, their use cases, when to use each, and provide illustrative code examples.
What is an Interface?
In Java, an interface is a reference type similar to a class that can only contain constants, method signatures, default methods, static methods, and nested types. Interfaces cannot contain instance fields or constructors. They are a way to achieve abstraction and multiple inheritance, allowing a class to inherit behavior from multiple sources.
Example of an Interface
public interface Animal {
void makeSound();
void eat();
}
In this example, the Animal
interface defines two methods: makeSound
and eat
. Any class implementing this interface will need to provide implementations for these methods.
What is an Abstract Class?
An abstract class, on the other hand, is a class that cannot be instantiated and may contain both abstract methods (without an implementation) and concrete methods (with an implementation). Abstract classes are primarily used when you want to provide a common base with shared functionality while leaving some methods to be implemented by subclasses.
Example of an Abstract Class
public abstract class Animal {
abstract void makeSound(); // abstract method
void eat() { // concrete method
System.out.println("This animal is eating.");
}
}
In this example, the Animal
abstract class provides a concrete method, eat
, while the abstract method makeSound
must be implemented by any concrete subclass.
Key Differences Between Interfaces and Abstract Classes
1. Purpose and Use Case
-
Interface: Primarily focused on defining a contract for behavior. Use it when you want to define capabilities that can be shared across multiple classes.
-
Abstract Class: Used to share code among related classes while allowing some methods to remain abstract. Use it when you want to provide default behavior or state, while also requiring subclasses to implement specific methods.
2. Implementation
-
Interface: All methods in an interface are implicitly public and abstract, unless specified as static or default. No method body is allowed unless the method is static or has a default implementation.
-
Abstract Class: Can contain both abstract methods (which do not have a body) and concrete methods (which do). This allows abstract classes to provide shared functionalities across subclasses.
3. Multiple Inheritance
-
Interface: Java supports multiple inheritance for interfaces, meaning a class can implement multiple interfaces.
-
Abstract Class: A class can extend only one abstract class, limiting the inheritance hierarchy.
4. Fields and Properties
-
Interface: Can only have static final fields (constants). Interfaces cannot hold state in the same way a class can.
-
Abstract Class: Can have instance fields that can maintain state. Abstract classes can include properties that subclasses can inherit.
5. Constructor
-
Interface: Cannot have constructors since they cannot be instantiated.
-
Abstract Class: Can have constructors that can be called from subclasses.
Examples of When to Use Each
When to Use Interfaces
If you're developing a system where various disparate classes need to share a common behavior or capability, interfaces are the way to go. For example, consider a payment processing system. You could define an interface for various payment types.
public interface Payment {
void processPayment(double amount);
}
public class PayPal implements Payment {
public void processPayment(double amount) {
System.out.println("Processing payment of " + amount + " through PayPal.");
}
}
public class CreditCard implements Payment {
public void processPayment(double amount) {
System.out.println("Processing payment of " + amount + " through Credit Card.");
}
}
When to Use Abstract Classes
If you have a base class that implements some default behavior but you want to define some specific behavior for subclasses, opt for an abstract class. For example:
public abstract class Shape {
abstract void draw();
void describe() {
System.out.println("This is a shape.");
}
}
public class Circle extends Shape {
void draw() {
System.out.println("Drawing a circle.");
}
}
public class Rectangle extends Shape {
void draw() {
System.out.println("Drawing a rectangle.");
}
}
In this case, Shape
provides a describe
implementation that all shapes can inherit, while each shape must implement its drawing logic.
Mixing Interfaces and Abstract Classes
In practice, you often use both interfaces and abstract classes in a Java application. For instance, you might have an interface for a repository pattern that specifies the contract for data access, while using an abstract class to share common database access logic.
public interface Repository<T> {
void add(T item);
void remove(T item);
T find(int id);
}
public abstract class AbstractRepository<T> implements Repository<T> {
// Common database operations and properties
@Override
public void remove(T item) {
System.out.println("Removing item from database.");
// Implementation to remove item
}
}
public class BookRepository extends AbstractRepository<Book> {
@Override
public void add(Book book) {
System.out.println("Adding book to database.");
// Implementation to add book
}
@Override
public Book find(int id) {
// Implementation to find book
return new Book();
}
}
In this design, AbstractRepository
provides common functionality for data access, while the Repository
interface defines essential data operations.
Lessons Learned
Both Java interfaces and abstract classes have their strengths and weaknesses. The choice between them really depends on the specific use case and design requirements of your application. In summary:
- Use interfaces when you need to define a contract for behavior that could be implemented by different classes in an unrelated hierarchy.
- Use abstract classes when you want to provide common functionality for closely related classes and still enforce a structure.
By strategically leveraging both features, your Java applications can become more modular, maintainable, and focused on code reusability. For a deeper dive into Java object-oriented programming concepts, check out the official Java documentation.
By understanding the key differences between interfaces and abstract classes, you're now equipped to make informed decisions in your Java development projects. If you have any questions or comments, feel free to leave them below!