Mastering Inheritance: Common Pitfalls in Java Polymorphism
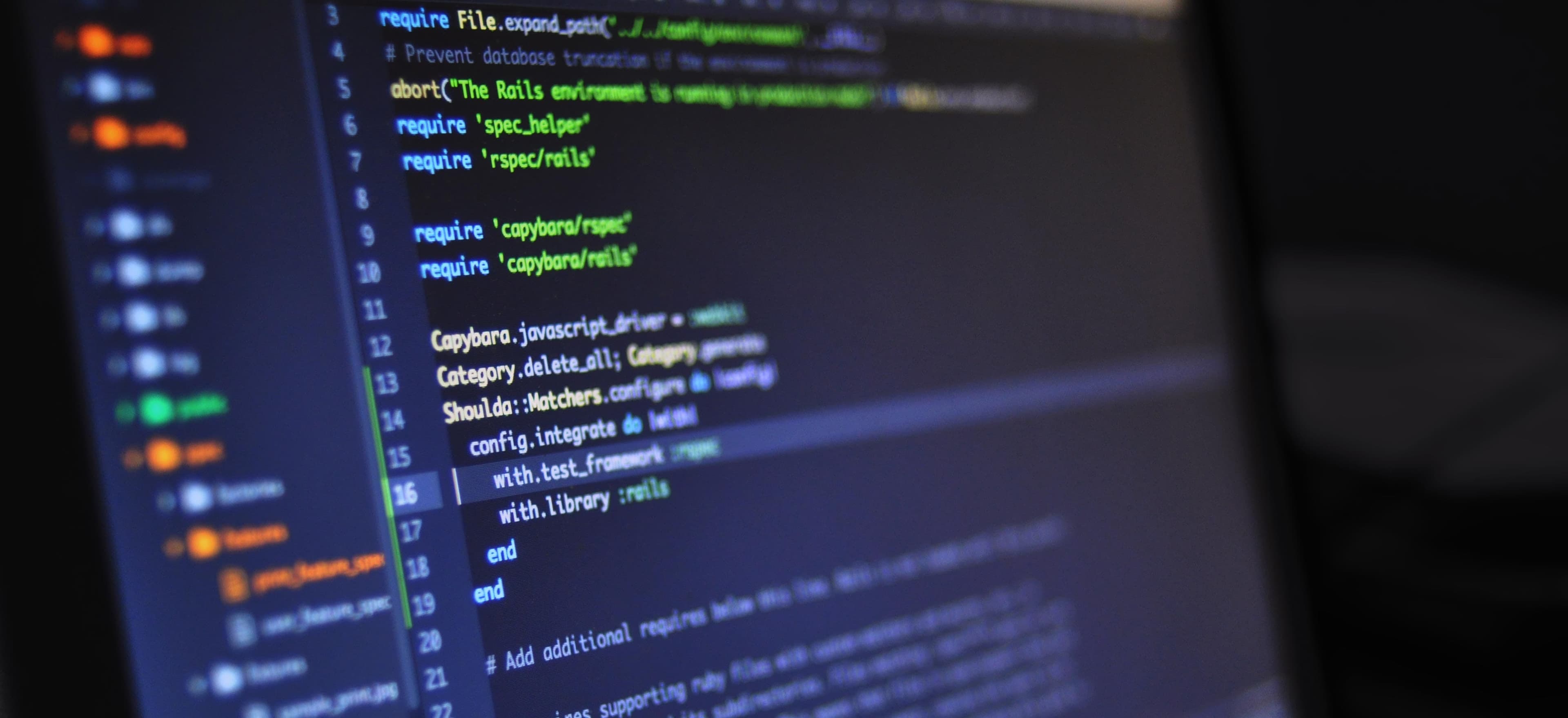
- Published on
Mastering Inheritance: Common Pitfalls in Java Polymorphism
Polymorphism is a cornerstone concept of object-oriented programming, allowing objects to be treated as instances of their parent class rather than their actual class. In Java, polymorphism can be achieved through method overriding and method overloading. However, understanding its subtleties is crucial for writing effective and bug-free code. In this post, we’ll explore common pitfalls in Java polymorphism, highlighting best practices through code examples and providing contextual insights.
Understanding Polymorphism
In Java, there are two types of polymorphism:
- Compile-Time Polymorphism (Method Overloading): This occurs when multiple methods with the same name are present in a class with different parameter lists.
- Run-Time Polymorphism (Method Overriding): This occurs when a subclass provides a specific implementation of a method that is already defined in its superclass.
Code Example of Method Overloading
class MathOperations {
// Method to add two integers
public int add(int a, int b) {
return a + b;
}
// Overloaded method to add three integers
public int add(int a, int b, int c) {
return a + b + c;
}
}
public class Main {
public static void main(String[] args) {
MathOperations mathOps = new MathOperations();
System.out.println("Sum of two integers: " + mathOps.add(5, 10)); // 15
System.out.println("Sum of three integers: " + mathOps.add(5, 10, 15)); // 30
}
}
Commentary on the Code
In this simple example, the MathOperations
class demonstrates method overloading. The add
method is defined twice, allowing flexibility in how many integers you want to sum up. This technique illustrates compile-time polymorphism, enhancing code readability without cluttering it.
Common Pitfalls in Method Overriding
While overriding methods can enhance the functionality of inherited classes, there are several pitfalls to watch out for:
1. Failing to Use @Override
Annotation
The @Override
annotation is used to indicate that a method is intended to override a method in a superclass. While it’s not mandatory, it helps catch errors at compile-time.
class Parent {
void show() {
System.out.println("Parent class show() method");
}
}
class Child extends Parent {
@Override
void show() {
System.out.println("Child class show() method");
}
}
Why Use @Override
?
By incorporating the @Override
annotation, if a method signature in the child class does not match any method in the parent class, the compiler will throw an error. This can save time and reduce bugs in large codebases.
2. Incorrect Access Modifiers
When overriding a method, ensure that the access modifier in the subclass is compatible with the superclass. You cannot reduce the visibility of an inherited method.
class Parent {
protected void display() {
System.out.println("Parent display");
}
}
class Child extends Parent {
// This will cause a compile-time error
private void display() {
System.out.println("Child display");
}
}
Commentary on Access Modifiers
In this example, attempting to change the access modifier from protected
in the parent class to private
in the child class results in an error. Proper understanding of access modifiers ensures the integrity of the method inheritance structure.
3. Overriding Static Methods
Static methods belong to the class rather than the object, and thus, they cannot be overridden. Instead, when you define a static method in a subclass with the same name, you create a method hiding.
class Parent {
static void staticMethod() {
System.out.println("Static method in Parent");
}
}
class Child extends Parent {
static void staticMethod() {
System.out.println("Static method in Child");
}
}
public static void main(String[] args) {
Parent.staticMethod(); // Output: Static method in Parent
Child.staticMethod(); // Output: Static method in Child
}
Why Understand Static Methods?
Understanding the difference between method overriding and method hiding is essential for managing code flows effectively. Static methods don't follow polymorphic behavior, which may lead to unexpected issues if developers assume otherwise.
4. Returning a Subtype in Overridden Methods
One significant advantage of method overriding is that you can return a subtype of the method's return type in the overriding version. However, if you attempt to return a supertype instead, you lose polymorphic behavior.
class Vehicle {
Vehicle getType() {
return this;
}
}
class Car extends Vehicle {
@Override
Car getType() {
return this; // Returning subtype
}
}
Commentary on Return Types
In this case, getType
in the subclass correctly returns a Car
, maintaining the integrity of polymorphic behavior. Returning the correct type allows you to leverage the specific features of the subclass without losing the benefit of the method's general signature from the base class.
Diagnosing Common Runtime Issues
Even with all best practices followed, runtime issues can occur. Here are some common sources:
1. ClassCastException
This can happen when you attempt to downcast a superclass reference to a subclass that it does not refer to.
Vehicle vehicle = new Vehicle();
Car car = (Car) vehicle; // This will throw ClassCastException
How to Prevent ClassCastException?
Utilize the instanceof
operator to check the type before performing the cast:
if (vehicle instanceof Car) {
Car car = (Car) vehicle;
} else {
System.out.println("Vehicle is not an instance of Car");
}
2. Inconsistent Method Functionality
Maintaining consistent behavior across overridden methods is essential. If you inadvertently change the logic in a subclass, you may produce bugs within existing code that relies on the base class implementation.
Key Takeaways
Mastering inheritance and polymorphism in Java requires an understanding of both the foundational principles and the common pitfalls that developers face. Through careful design, the use of annotations, and attention to method signatures and return types, you can craft robust and flexible applications.
For more on OOP concepts, explore resources such as the Oracle Java Tutorials or Java Design Patterns.
By recognizing and overcoming common pitfalls in polymorphism, you’ll not only enhance your Java skills but also build scalable solutions that stand the test of time. Happy coding!