Common Mistakes with Java Operators: Avoid These Pitfalls!
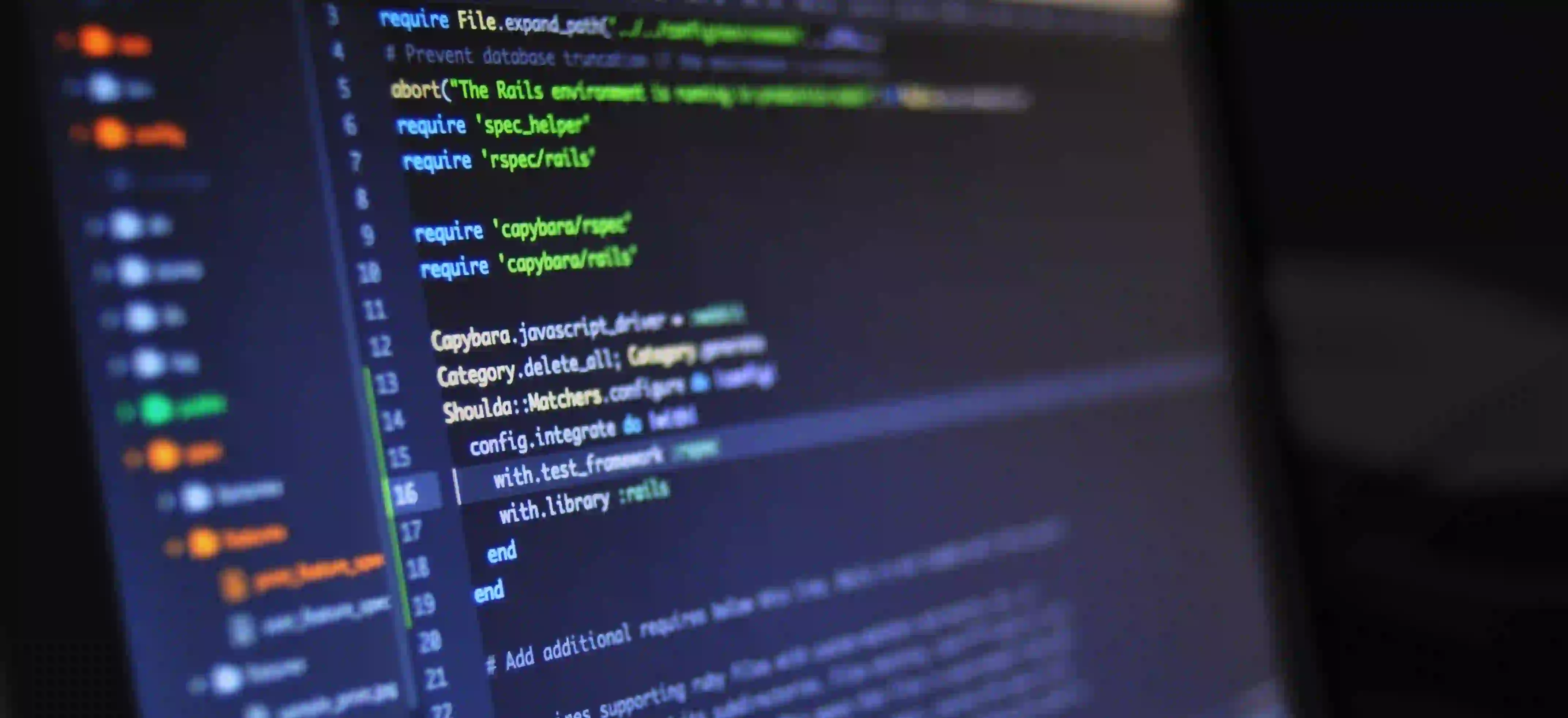
Common Mistakes with Java Operators: Avoid These Pitfalls!
Java is one of the most widely used programming languages in the world. It has a rich set of operators that allow developers to perform a variety of tasks, ranging from arithmetic operations to logical comparisons. However, even experienced developers can make mistakes when using Java operators. In this blog post, we will explore common pitfalls related to Java operators, complete with examples and explanations. By the end of this post, you'll not only understand these mistakes but also be equipped with the knowledge to avoid them.
Understanding Java Operators
Java operators are special symbols that perform operations on variables and values. They can be classified into several categories:
-
Arithmetic Operators: Used for mathematical calculations (e.g.,
+
,-
,*
,/
,%
). -
Relational Operators: Used to compare values (e.g.,
==
,!=
,>
,<
,>=
,<=
). -
Logical Operators: Used to combine boolean expressions (e.g.,
&&
,||
,!
). -
Bitwise Operators: Used for manipulation of bits (e.g.,
&
,|
,^
,~
,<<
,>>
). -
Assignment Operators: Used to assign values to variables (e.g.,
=
,+=
,-=
). -
Ternary Operator: A shorthand for an
if-else
statement (e.g.,condition ? valueIfTrue : valueIfFalse
).
Common Mistakes and How to Avoid Them
1. Confusing =
with ==
One of the most common mistakes in Java is confusing the assignment operator (=
) with the equality operator (==
).
Example:
int a = 5;
if (a = 10) { // Error: trying to assign value to 'a' in a condition
System.out.println("a is 10");
}
Why is this an error? The code should use ==
to compare values, rather than =
which assigns a value.
Correction:
if (a == 10) {
System.out.println("a is 10");
}
2. Using ==
with Objects
In Java, the ==
operator checks for reference equality, rather than value equality when used with objects. This can lead to unexpected results.
Example:
String str1 = new String("Hello");
String str2 = new String("Hello");
if (str1 == str2) {
System.out.println("These strings are equal");
} else {
System.out.println("These strings are not equal");
}
Why is this a common mistake? The output will be "These strings are not equal" because str1
and str2
reference different memory locations.
Correction:
Always use the .equals()
method to compare object values.
if (str1.equals(str2)) {
System.out.println("These strings are equal");
}
3. Misunderstanding Short-Circuit Evaluation
Java uses short-circuit evaluation for its logical operators &&
and ||
. This means that in an expression A && B
, if A is false, Java won't evaluate B, because the overall expression will never be true.
Example:
Boolean value = false;
if (value && expensiveOperation()) {
// expensiveOperation() is not executed
}
Why is this a pitfall? If expensiveOperation()
has side effects or is crucial to the logic, it might not run if the first condition is false.
Correction:
Be aware of short-circuit behavior and ensure that your logical expressions are structured to account for this.
4. Integer Division Pitfalls
In Java, when dividing two integers, the result will also be an integer, which can lead to unexpected truncation of values.
Example:
int a = 5;
int b = 2;
System.out.println(a / b); // Outputs 2, not 2.5
Why is this important? If you're expecting a decimal, this behavior can lead to bugs.
Correction:
To get a decimal result, ensure at least one operand is a double.
double result = (double) a / b;
System.out.println(result); // Outputs 2.5
5. Not Using Parentheses Correctly
Operator precedence can lead to confusion, often resulting in logic errors if parentheses are not used correctly.
Example:
int result = 5 + 10 * 2; // Outputs 25, because * has higher precedence
Why should we worry? This can lead to no intuitive errors where the programmer expects one outcome but gets another due to operator precedence.
Correction:
Use parentheses to make your intentions clear.
int result = 5 + (10 * 2); // Clearly defines the intended operation.
6. Forgetting to Handle Nulls
When using ==
and !=
, null references can lead to NullPointerExceptions. Java does handle nulls with normal conditions, but it’s always good to check for nulls explicitly.
Example:
String str = null;
if (str.equals("Test")) { // Throws NullPointerException
System.out.println("Valid");
}
Why is this critical? Neglecting null checks can cause your application to crash unexpectedly.
Correction:
Add a null check before calling methods on objects.
if (str != null && str.equals("Test")) {
System.out.println("Valid");
}
7. Ignoring Data Types
Different data types can cause unexpected results or compile errors when operators are applied.
Example:
int a = 5;
String b = "5";
if (a == b) { // Compile Error
// This won't compile
}
Why is this a concern? Comparing different data types leads to compile-time errors.
Correction:
Always ensure that types match before making comparisons or calculations.
if (a == Integer.parseInt(b)) {
System.out.println("Equal");
}
Final Considerations
Java operators provide powerful functionalities that can help you create efficient and succinct code. However, making mistakes with these operators can lead to bugs that are often hard to track down. By understanding common pitfalls and knowing how to avoid them, you can write cleaner and more reliable Java code.
For further reading on the intricacies of Java, check out the Oracle Java Documentation and consider joining developer communities like Stack Overflow for deeper discussions and insights.
Call to Action
Have you encountered any other common mistakes with Java operators? Share your experiences in the comments below and help others learn from your insights!