Common Pitfalls When Working with Java Variables
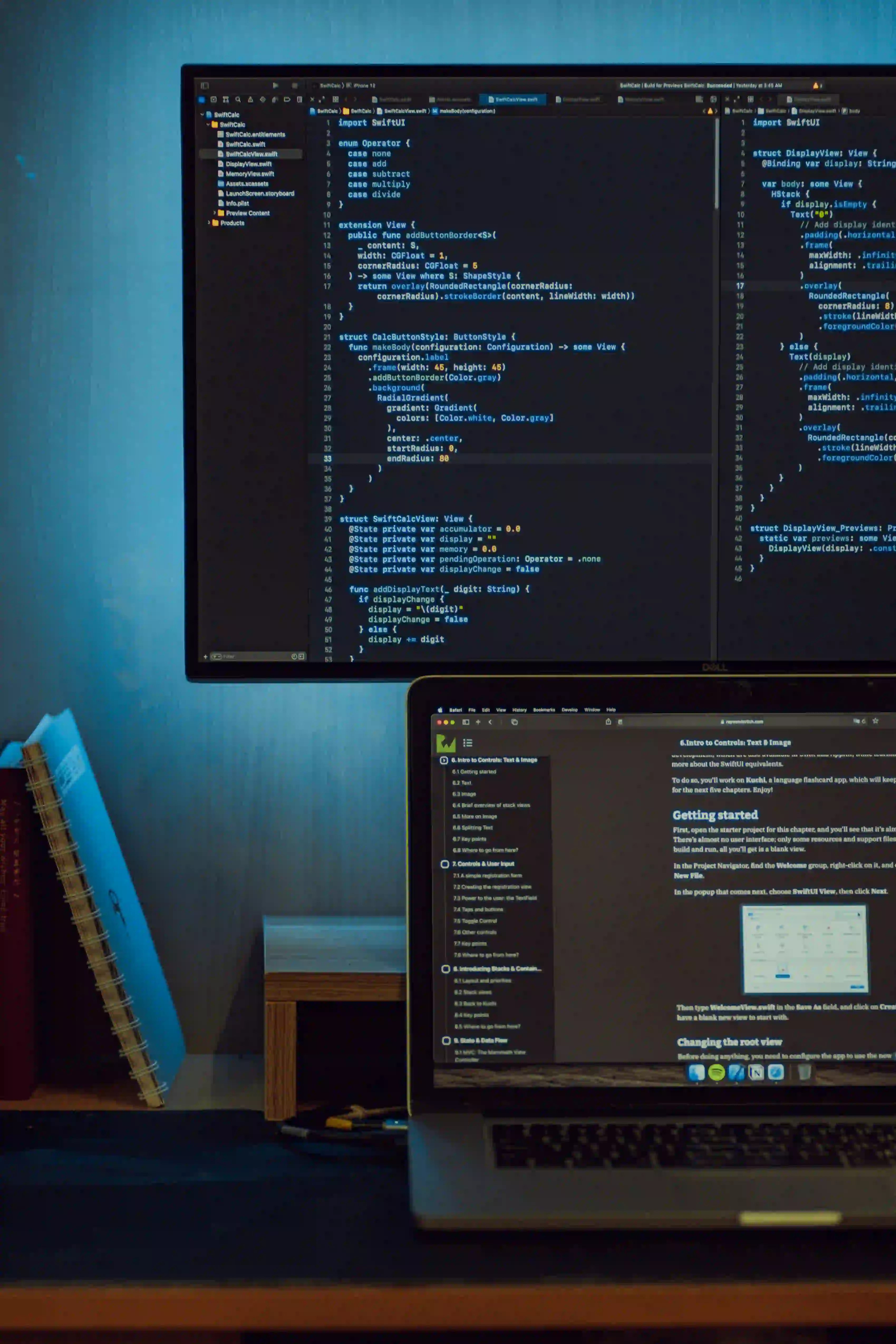
Common Pitfalls When Working with Java Variables
Java is a powerful and widely-used programming language that is designed to be simple and flexible. However, even experienced developers can stumble when dealing with its variable handling. Variables are fundamental building blocks in any programming language, including Java, and understanding how to use them effectively is crucial for writing clean, efficient code. In this blog post, we will delve into common pitfalls when working with Java variables, explore best practices, and provide illustrative code snippets with commentary to help you avoid these mistakes.
1. Understanding Variable Scope
One of the most common issues developers face in Java is variable scope. The scope of a variable determines where it can be accessed in the code.
Code Snippet
public class ScopeExample {
public void methodOne() {
int localVar = 10; // This variable is scoped to methodOne
System.out.println("Local variable: " + localVar);
}
public void methodTwo() {
// System.out.println(localVar); // This will result in a compilation error
}
}
Commentary
In this example, the variable localVar
is declared within methodOne
, making it inaccessible in methodTwo
. This is a fundamental aspect of Java's variable scope rules. Trying to reference localVar
outside its declaration context results in a compilation error.
Why it Matters
Understanding variable scope prevents accidental reference to variables that do not exist in the current context, thus maintaining clean and readable code. For a deeper understanding of variable scope in Java, you may check the Java Tutorials.
2. Naming Conventions
Java has conventions for naming variables that are designed to enhance readability and maintainability. Deviating from these conventions can lead to confusion.
Code Snippet
public class NamingConvention {
private int scoreValue; // Good: follows camelCase
public void calculateScore() {
int scorevalue; // Bad: not following camelCase
scorevalue = 100;
int ScoreValue; // Bad: confusing due to mixed casing
}
}
Commentary
In this snippet, scoreValue
is correctly named in camelCase. However, scorevalue
and ScoreValue
are poorly named, deviating from Java conventions and causing potential confusion.
Why it Matters
Using consistent naming conventions makes your code more understandable and maintainable. It helps other developers (and your future self) to quickly understand the purpose of a variable. For more on naming conventions, refer to the Java Code Conventions.
3. Misunderstanding Primitive vs Reference Types
Java distinguishes between primitive types (such as int
, char
, etc.) and reference types (like Arrays
and Objects
). Misunderstanding this can lead to unexpected behavior.
Code Snippet
public class PrimitiveVsReference {
public static void main(String[] args) {
int primitive1 = 5;
int primitive2 = primitive1; // Value is copied
primitive2++;
System.out.println("Primitive1: " + primitive1); // Outputs 5
System.out.println("Primitive2: " + primitive2); // Outputs 6
int[] reference1 = {1, 2, 3};
int[] reference2 = reference1; // Reference is copied
reference2[0] = 99;
System.out.println("Reference1[0]: " + reference1[0]); // Outputs 99
}
}
Commentary
In this code, we see the difference between primitive and reference types. primitive1
and primitive2
are separate variables, thus changing primitive2
does not affect primitive1
. On the other hand, reference1
and reference2
point to the same array. When we modify reference2
, it affects reference1
as well.
Why it Matters
This distinction is crucial when dealing with data types in Java, especially in large applications. Mixing up these types can lead to bugs that are hard to track down. Knowing when you are working with a copy of a value versus a reference to an object is essential for maintaining data integrity.
4. Forgetting to Initialize Variables
Another common mistake in Java is forgetting to initialize variables before use. Java does not allow the use of uninitialized local variables.
Code Snippet
public class InitializationExample {
public void exampleMethod() {
int number; // Declared but not initialized
// System.out.println(number); // Uncommenting this will result in a compilation error
number = 10; // Initialization
System.out.println("Initialized number: " + number); // Outputs 10
}
}
Commentary
In this example, the variable number
is declared but never initialized before use, which would lead to compilation issues. Java requires that all local variables be initialized before they are accessed.
Why it Matters
Ensuring all variables are initialized helps avoid runtime errors and ensures that your program behaves in a predictable manner. Always take the time to initialize your variables, either at the moment of declaration or just before their first use.
5. Dynamic Typing vs Static Typing
In Java, variable types are statically declared, which means that once a variable is declared, its type cannot change. Attempting to reassign a variable of a different type will lead to a compile-time error.
Code Snippet
public class TypeExample {
public static void main(String[] args) {
int num = 10;
// num = "Hello"; // This will cause a compilation error
System.out.println(num);
}
}
Commentary
In this case, trying to assign a String
to a variable that was declared as an int
would result in a compile-time error. Java's static typing enforces data types at compile time.
Why it Matters
Understanding Java's static typing is crucial for avoiding these types of errors. It helps catch bugs early in the development process and ensures that the code is type-safe.
Closing Remarks
Java provides a robust framework for variable management, but with that comes the responsibility of understanding how to use variables properly. By being mindful of variable scope, naming conventions, the distinction between primitive and reference types, initialization practices, and Java's static typing, you can enhance your Java programming skills significantly.
Avoiding these common pitfalls will lead you to write clearer, more maintainable code. With practice and adherence to these principles, you can become more proficient in handling Java variables in your projects.
For further reading, check out these resources:
- Java Variable Scope
- Java Naming Conventions
Remember, every small detail counts when programming. Avoiding these pitfalls will not only improve your code quality but also enhance your overall development experience. Happy coding!