Builder Pattern: Overcoming Object Creation Complexity
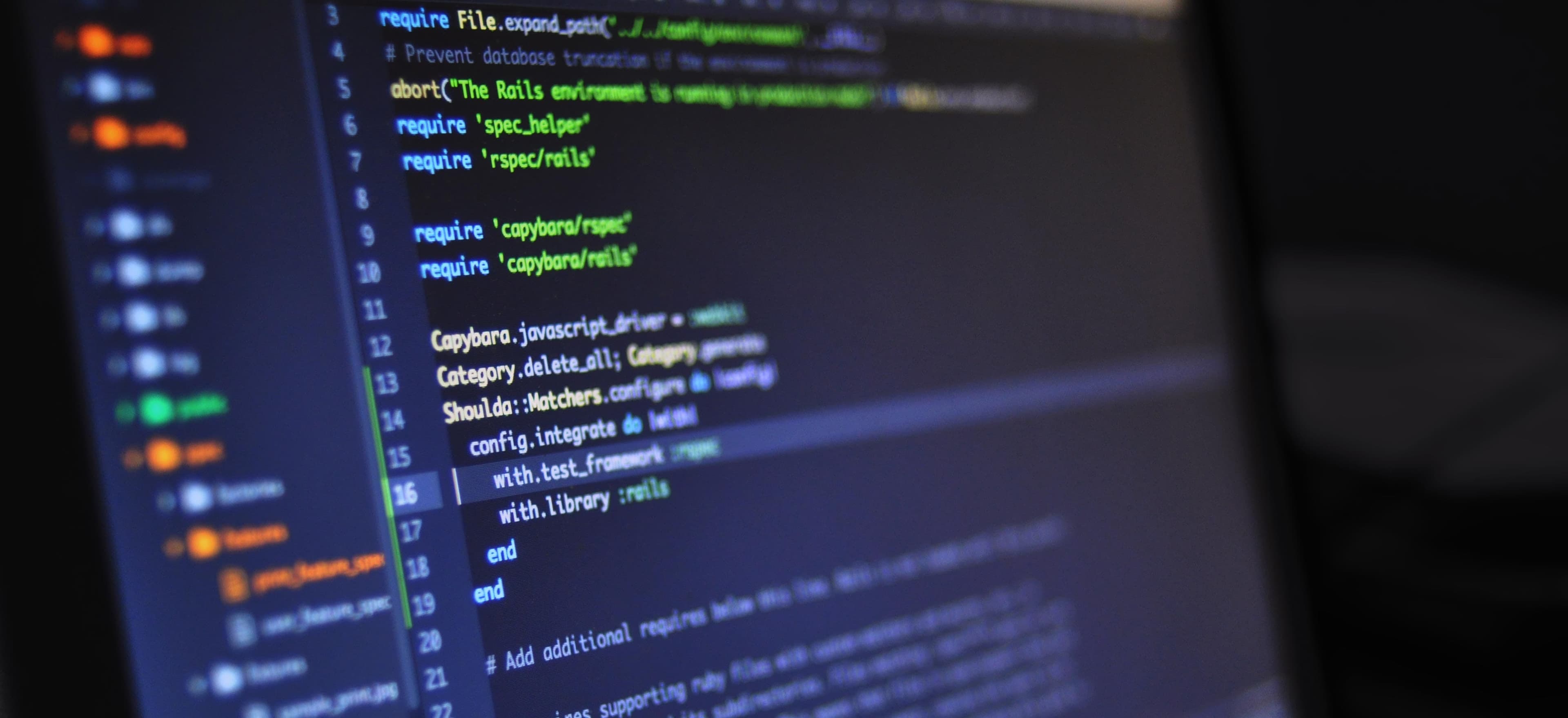
- Published on
Builder Pattern: Overcoming Object Creation Complexity
In the world of software development, complexity is an unavoidable yet manageable aspect. As a developer, you might find yourself in scenarios where object creation becomes cumbersome. This is especially true when an object requires a substantial number of properties or configurations. In such situations, design patterns come to our rescue. One such pattern is the Builder Pattern.
Understanding the Builder Pattern
The Builder Pattern is a creational design pattern that allows for the step-by-step construction of complex objects. It lets you create different representations of an object using the same construction code. By separating the construction process from the representation, it enhances code readability and reusability.
Key Benefits
- Simplifies Object Creation: Streamlines the process of creating complex objects by breaking it down into manageable parts.
- Immutable Objects: Facilitates the creation of immutable objects which are not supposed to change once they are built.
- Enhances Readability: Makes your code easier to understand, as it provides a clear path for creating an object.
Before implementing the Builder Pattern in Java, let’s explore its structure.
Structure of the Builder Pattern
The Builder Pattern generally consists of the following components:
- Builder: An interface that declares the methods for building parts of the Product.
- ConcreteBuilder: A class that implements the Builder interface. It constructs and assembles parts of the product.
- Director: A class that constructs an object using the Builder interface.
- Product: The complex object that is being built.
Visual Representation
You can visualize the Builder Pattern as follows:
Director ---> Builder ---> ConcreteBuilder
^
|
Product
Implementing the Builder Pattern in Java
Let's implement a practical example to elucidate the Builder Pattern. Suppose we want to create a House
object that has multiple properties such as size, color, and number of rooms.
Step 1: Define the Product Class
This is the class that will represent the object we are constructing.
public class House {
private final int size;
private final String color;
private final int numRooms;
private House(HouseBuilder builder) {
this.size = builder.size;
this.color = builder.color;
this.numRooms = builder.numRooms;
}
public static class HouseBuilder {
private final int size; // required
private String color; // optional
private int numRooms; // optional
public HouseBuilder(int size) {
this.size = size;
}
public HouseBuilder color(String color) {
this.color = color;
return this;
}
public HouseBuilder numRooms(int numRooms) {
if (numRooms < 1) {
throw new IllegalArgumentException("Number of rooms must be at least 1");
}
this.numRooms = numRooms;
return this;
}
public House build() {
return new House(this);
}
}
@Override
public String toString() {
return "House{" +
"size=" + size +
", color='" + color + '\'' +
", numRooms=" + numRooms +
'}';
}
}
Commentary on the Code
- Encapsulation: The constructor is private, keeping
House
immutable after creation. Hence, once created, you cannot change the properties. - Required and Optional Parameters: The
HouseBuilder
constructor takes a mandatory parameter (size). Optional parameters (color, numRooms) are provided using method chaining. - Method Chaining: This technique enhances the readability of the code. Each setter returns the builder object itself, allowing calls to be chained together fluidly.
Step 2: Building the House
Now that we have defined our product and builder class, let’s see how to build a House
object.
public class BuilderPatternExample {
public static void main(String[] args) {
House house = new House.HouseBuilder(300)
.color("Blue")
.numRooms(4)
.build();
System.out.println(house);
}
}
Explanation
In this example, we create a House
of size 300 with the color blue and four rooms. The builder pattern allows us to create the object step by step, leading to cleaner and clearer code. The build()
method is called at the end to finalize the object.
Scenarios For Using the Builder Pattern
The Builder Pattern shines in various scenarios:
- Complex Object Creation: When an object requires many configurations or attributes.
- Immutable Objects: When you want to create an object that should be immutable after creation.
- Fluent Interfaces: When you prefer a fluent interface for building objects.
Wrapping Up
The Builder Pattern is a powerful design concept that simplifies object creation in complex applications. By clearly separating the object from the construction process, we attain cleaner, more maintainable code. Not only does it facilitate easier creation of complex objects, but it also enhances readability and reduces the likelihood of errors.
Incorporating this pattern into your codebase can greatly enhance the ease of managing object creation. If you want to further explore design patterns, this link offers additional insights.
Are you ready to tackle object creation complexity in your Java projects with the Builder Pattern? Happy coding!