Common Serialization Pitfalls in Java You Should Avoid
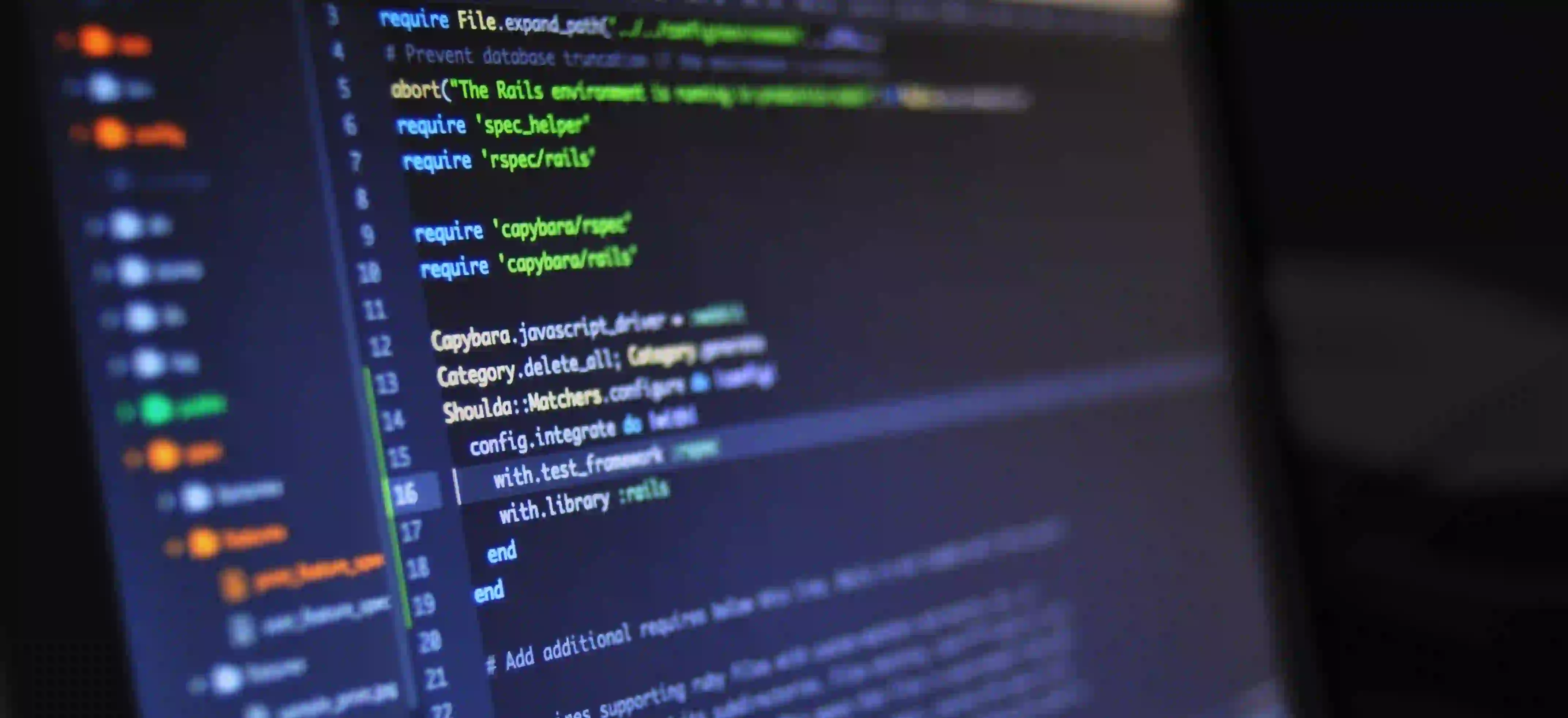
Common Serialization Pitfalls in Java You Should Avoid
Java serialization is a vital feature in the Java programming language that allows an object to be converted into a byte stream, enabling it to be easily saved to a file or transferred over a network. However, there are several pitfalls in serialization that can lead to significant issues if not handled properly. In this post, we will delve into common pitfalls associated with serialization in Java, examining the nuances, pitfalls, and best practices to avoid them.
Understanding Java Serialization
Before diving into the pitfalls, it's essential to grasp what Java serialization actually entails. Serialization in Java is accomplished through the Serializable
interface. This interface does not require any methods to be implemented, which makes it deceptively straightforward to use. You simply mark the class with the Serializable
marker interface, and Java takes care of the rest.
import java.io.Serializable;
public class User implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
// Constructors, getters, setters omitted for brevity
public User(String name, int age) {
this.name = name;
this.age = age;
}
}
In the snippet above, we created a simple User
class and declared it as Serializable
. The serialVersionUID
serves as a version control mechanism.
The Importance of serialVersionUID
Pitfall 1: Ignoring serialVersionUID
One of the most common mistakes made by developers is neglecting the serialVersionUID
. This uniquely identifies the version of a serialized class. If a class evolves (for instance, through the addition of fields), and the serialVersionUID
is not updated, it can lead to InvalidClassException
.
Best Practice:
Always declare the serialVersionUID
explicitly. This prevents unintended issues during deserialization when the class structure evolves.
private static final long serialVersionUID = 123456789L;
By providing a serialVersionUID
, you explicitly control the versioning of your class. This is particularly important for classes that are likely to change in future releases.
Reference Link:
Official Java Documentation on serialVersionUID
.
Lack of Control Over Serializing Fields
Pitfall 2: Serialization of Non-Serializable Fields
When a class has fields that are either not serializable or can lead to serialization issues, you’ll encounter problems. Java tries to serialize the entire object graph, and if it encounters a non-serializable field, it throws a NotSerializableException
.
public class Account implements Serializable {
private String accountNumber;
// Non-Serializable field
private transient DatabaseConnection dbConnection; // Use transient keyword
// Constructors, getters, setters omitted for brevity
}
In this example, dbConnection
is not serializable. By marking it as transient
, you instruct Java to ignore this field during serialization, avoiding potential exceptions.
Best Practice:
Always remember to mark non-serializable fields with transient
. This gives you control over what gets serialized.
Handling Security Risks
Pitfall 3: Security Vulnerabilities
Serialization can open doors to various security risks. For example, attackers can manipulate serialized objects to perform attacks like bypassing authentication checks or injecting malicious payloads.
Best Practices:
- Avoid Serialization of Trusted Objects: Don’t serialize sensitive or trusted objects that can impact application security.
- Implement Custom ReadObject Method: Use a custom
readObject
method to ensure object integrity upon deserialization.
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
ois.defaultReadObject();
// Additional validation logic can be implemented here.
}
This will allow you to validate the object once it’s deserialized, ensuring that its state is always consistent with expected integrity constraints.
Compatibility Issues
Pitfall 4: Changes in Class Structure
As your application evolves, you might need to add or remove fields from your classes. If you don't handle these changes properly when the class is serialized, it can lead to compatibility problems.
Best Practice:
- Use the transient Keyword: Whenever possible, add new fields as transient. This will allow you to maintain backward compatibility.
- Customize Read and Write object methods.
Here's a knowledgeable example of these methods:
private void writeObject(ObjectOutputStream oos) throws IOException {
oos.defaultWriteObject();
// Put additional logic, like handling new fields.
}
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
ois.defaultReadObject();
// Handle old and new fields here
}
Performance Concerns
Pitfall 5: Inefficient Serialization
Serialization can be inefficient, particularly if large objects or object graphs are serialized. This can lead to performance issues in your application.
Best Practices:
- Use appropriate Serialization Frameworks: Consider using alternative serialization libraries like Kryo or Protocol Buffers for better performance.
- Custom Serialization Logic: Optimize the serialization process by serializing only the required fields and avoiding unnecessary object graphs.
The Last Word
Avoiding these common serialization pitfalls in Java can significantly enhance robustness, performance, and security in object-oriented applications. While Java serialization is straightforward, it requires careful consideration of aspects like version control, handling of non-serializable fields, security risks, compatibility, and performance concerns.
By following the best practices discussed, including the effective use of serialVersionUID
and the transient keyword, you can mitigate potential issues in serialization while maintaining efficient and secure applications. Happy coding!