Boosting CI/CD Speed: Overcoming Pipeline Bottlenecks
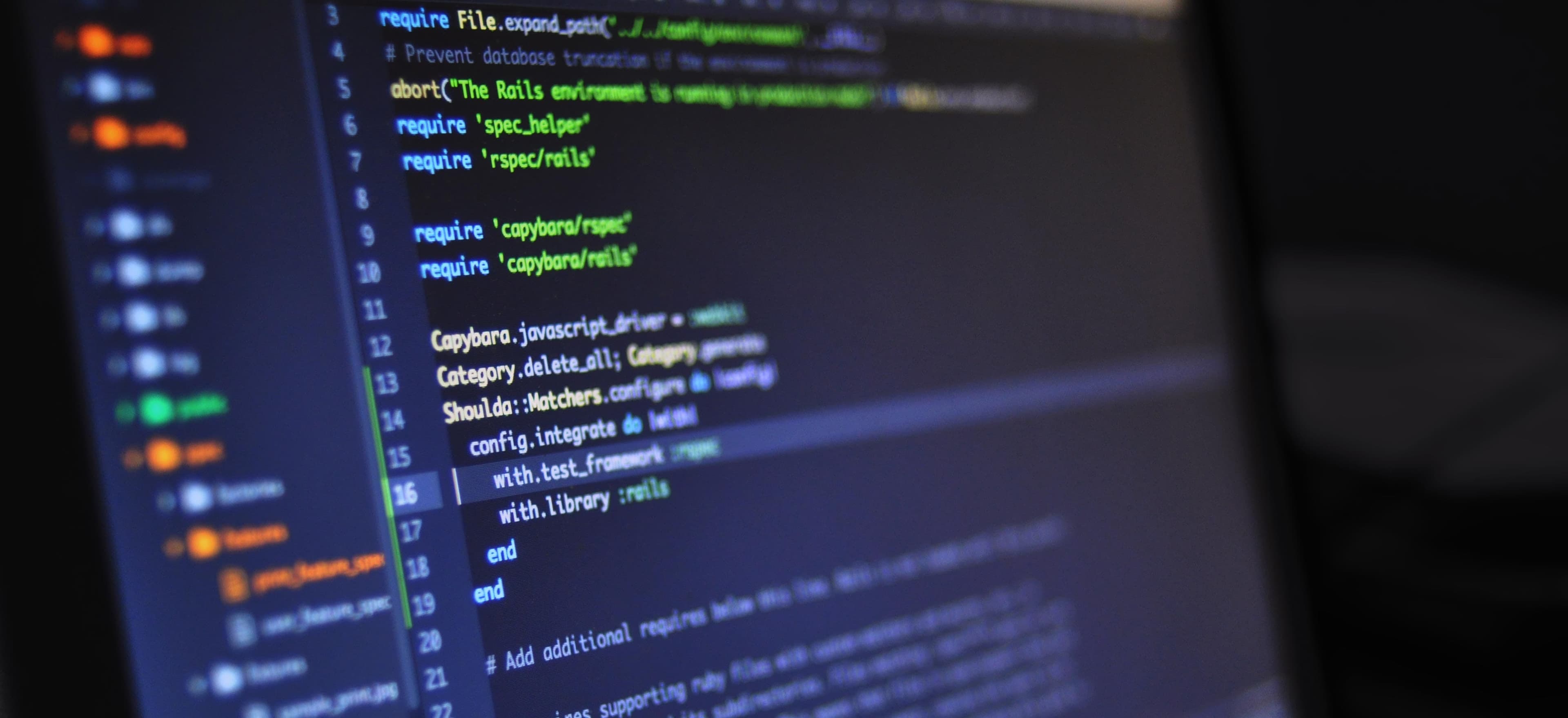
- Published on
Boosting CI/CD Speed: Overcoming Pipeline Bottlenecks
Continuous Integration (CI) and Continuous Deployment (CD) are powerhouses in modern software development. They help teams ensure consistent integration of code changes, optimize testing, and speed up deployment processes. However, these processes can come to a grinding halt when bottlenecks occur in the pipeline. In this blog post, we will explore common bottlenecks in CI/CD pipelines and provide effective strategies to mitigate them. Let’s dive in!
Understanding CI/CD Pipelines
Before we tackle bottlenecks, it's important to understand what a CI/CD pipeline entails. At its core, a CI/CD pipeline automates the process of code changes from a developer’s machine to production. This typically includes:
- Source Code Management: Code is stored in repositories (like Git).
- Continuous Integration: Code changes are automatically built and tested.
- Continuous Deployment: Once tested, code is deployed to production environments automatically.
Once you follow these steps, you can ensure fast iteration and shorter release cycles.
Identifying Common Bottlenecks
A pipeline bottleneck can occur at various stages due to a range of issues. Here’s a closer look at some recurring bottlenecks:
1. Testing Overhead
Automated tests are crucial for catching errors early. However, if your test suite is slow or inefficient, it can significantly delay the CI/CD process.
2. Resource Constraints
CI/CD processes require computational resources—like CPUs, memory, and storage. If these resources are limited or suboptimal, they can pose significant speed challenges.
3. Networking Issues
Slow connections can affect code pulling from repositories or downloading dependencies.
4. Infrequent Integration
When team members manually integrate code changes, delays can stack and lead to large, complex merges that break the build.
Strategies to Overcome Pipeline Bottlenecks
Now that we’ve identified common bottlenecks in CI/CD pipelines, let’s discuss strategies to boost efficiency and eliminate delays.
1. Optimize Your Tests
Focusing on the Right Tests
While it's essential to have a comprehensive test suite, not all tests are created equal. Focus on:
- Unit Tests: They run quickly and are essential for validating the individual components.
Using a testing framework like JUnit in Java can help streamline your unit tests. Here is a simple example:
import static org.junit.Assert.*;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3)); // Ensures add() method works as expected
}
}
The @Test
annotation signals JUnit to execute the following method as a test.
Parallel Testing
To reduce the time spent on testing, consider running tests in parallel. Many CI/CD tools support running tests in parallel, which can drastically decrease test execution time.
2. Scale Your Infrastructure
Use Cloud Resources
Consider utilizing cloud providers like AWS or Azure for scalable compute resources. This flexibility allows you to adjust resource allocations based on current pipeline demand.
CI/CD Tools with Better Performance
Choose a CI/CD system that can scale with your needs. Look into tools like Jenkins, CircleCI, or GitHub Actions, which can efficiently handle workloads with greater speed.
3. Improve Networking Efficiency
Caching Dependencies
Use caching mechanisms to keep the frequently used dependencies readily available in your environment, reducing unnecessary downloads.
For example, in a Java Maven project, you can cache the .m2
directory:
cache:
paths:
- ~/.m2/repository
By caching your repositories, the CI server won’t need to re-download libraries that have not changed.
Optimize Your Repository
Split large repositories into smaller, more manageable ones if possible. Large repositories typically slow down cloning and synchronization processes.
4. Automate Integration
Utilize branch policies and pull request workflows to enforce smoother code integrations. For example, integrating code into the main branch more frequently (like on a daily basis) can prevent complex merges.
5. Monitor Pipeline Performance
Implement Metrics and Alerts
Use monitoring tools to track pipeline performance. This will help you quickly identify when bottlenecks occur. Tools like Grafana or DataDog can integrate with your CI/CD tools to provide insightful dashboards.
Regular Reviews
Conduct regular reviews of the CI/CD pipeline. This ensures that everyone is on the same page and encourages continuous improvement. Hold team retrospectives to discuss what is working and what isn’t.
Closing Remarks
By proactively identifying and tackling bottlenecks within your CI/CD pipeline, you can significantly enhance development speed and efficiency. Implementing these strategies not only improves turnaround times but also boosts team morale and productivity. Start small by optimizing tests, consider the scalability of your infrastructure, and always explore new technologies that can enhance your deployment process.
In today's fast-paced software development landscape, efficiency is key to staying competitive. For more information about CI/CD best practices, check out the official guidelines from Atlassian.
Whether you are a seasoned developer or just venturing into CI/CD, optimizing your pipelines is a continuous journey that pays off in improved software delivery and product quality. What challenges have you faced in your CI/CD journey, and how have you worked to overcome them? Share your experiences below!