Understanding Java's Access Modifiers: Their Real Impact
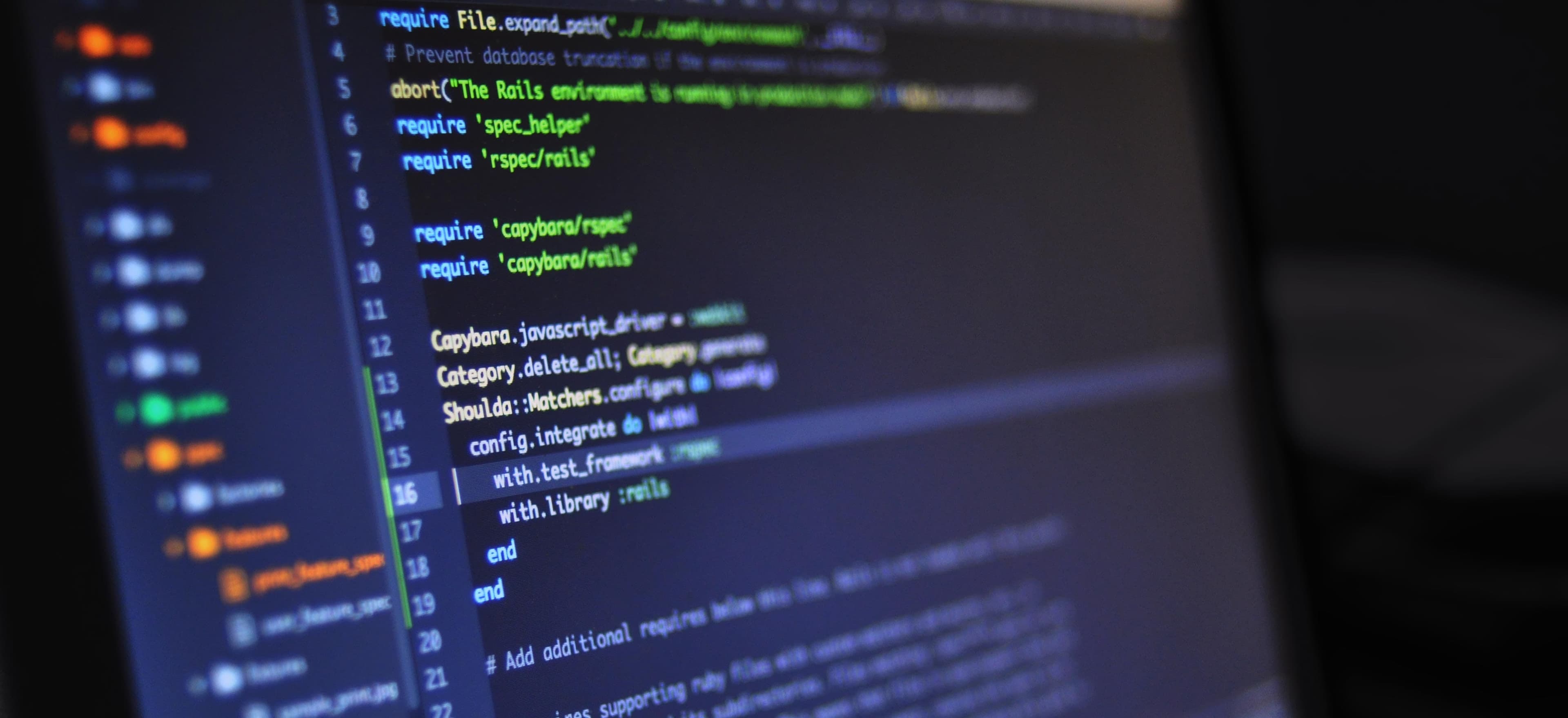
- Published on
Understanding Java's Access Modifiers: Their Real Impact
Java is an object-oriented programming language known for its use of classes and objects to create structured and maintainable code. One key concept in Java that helps us achieve proper encapsulation and data hiding is access modifiers. Access modifiers dictate the visibility of class members (fields, methods, and constructors) and are fundamental to designing robust Java applications.
In this blog post, we will delve into the four primary access modifiers in Java - public
, protected
, default
, and private
. We will explore their nuances with several code examples, explaining why each modifier is used and the real impact they have on the structure of your programs. Let’s get started!
The Four Access Modifiers in Java
1. Public
The public
access modifier is the most permissive. Classes, methods, or fields declared as public
are accessible from any other class in any package.
Why Use Public?
You want to provide access to certain class members across your entire application because they serve as a public API or interface.
public class PublicExample {
public String name;
public PublicExample(String name) {
this.name = name;
}
public void display() {
System.out.println("Name: " + name);
}
}
In the above example:
- The
PublicExample
class and its members are accessible from any other class. - The
display
method can be invoked from outside this class without restriction.
Impact of Public Modifier:
The public
modifier promotes accessibility but be cautious. Overexposure can lead to tight coupling, making your program harder to manage and modify.
2. Private
The private
access modifier is the most restrictive. Members declared as private
can only be accessed within the same class.
Why Use Private?
Encapsulation is key to object-oriented design. You might want to hide certain data or methods to prevent external classes from altering or interacting with them in unsafe ways.
public class PrivateExample {
private String secret;
public PrivateExample(String secret) {
this.secret = secret;
}
private void revealSecret() {
System.out.println("The secret is: " + secret);
}
public void showSecret() {
revealSecret();
}
}
In this code:
- The field
secret
and methodrevealSecret
cannot be accessed from outsidePrivateExample
. showSecret
acts as a controlled interface to access the secret.
Impact of Private Modifier:
Using private
increases security and robustness in your code because it minimizes unintended interference, thus easing debugging and implementing changes down the line.
3. Protected
The protected
access modifier allows members to be accessed within their own package and by subclasses in other packages.
Why Use Protected?
Usually employed in inheritance scenarios, protected
allows for a controlled extension of class members in specialized subclasses.
public class Animal {
protected String species;
protected void speak() {
System.out.println("Generic animal sound");
}
}
public class Dog extends Animal {
public void speak() {
System.out.println("Woof! I am a " + species);
}
}
Here:
species
andspeak
in classAnimal
are accessible to any subclass likeDog
.Dog
can utilize and override these members.
Impact of Protected Modifier:
The protected
modifier strikes a balance between accessibility and data hiding, making it particularly useful in inheritance hierarchies. However, overusing it can still lead to fragile structures.
4. Default (Package-Private)
When no access modifier is specified, Java uses the default access level. Members are only accessible within their own package.
Why Use Default?
Use default access when you want to limit visibility to a specific package while avoiding the noise of declaring access levels.
class PackagePrivateExample {
String name;
PackagePrivateExample(String name) {
this.name = name;
}
void display() {
System.out.println("Name: " + name);
}
}
In this code:
- The class members are only accessible within the same package.
- This is helpful in scenarios where you want to create an internal API that should not be exposed outside its package.
Impact of Default Modifier:
While it fosters package-level encapsulation, it may also limit usability if classes need to interact across packages.
Choosing the Right Access Modifier
Choosing the right access modifier is pivotal in defining class members' visibility and accessibility effectively. Here are a few tips:
- Favor Private: Start with the
private
modifier and open up access only when necessary. - Use Public Judiciously: Avoid making too many members
public
, minimizing connected dependencies. - Embrace Protected Wisely: Use
protected
in inheritance scenarios, but always consider the hierarchy's stability. - Consider Default for Package-Level Design: If you need a clean interface within a package, consider using default access.
Wrapping Up
Understanding Java's access modifiers is essential for writing clean, maintainable, and secure code. Modifiers like public
, protected
, private
, and default collectively form the backbone of encapsulation in object-oriented programming. They not only define visibility but also enforce better design principles.
By thoughtfully applying these modifiers, you can cater to the complexities of scale and changes within your application. Here’s a quick recap of when to use which modifier:
- Use public for APIs and services meant for widespread access.
- Use private for sensitive or internal logic.
- Use protected for class members meant to be utilized in subclasses.
- Use default when you want to restrict usage to specific packages.
Further Reading
By understanding and applying Java's access modifiers effectively, you'll not only enhance your own programming skills, but you'll also architect better software solutions. Happy coding!
Checkout our other articles