Common Pitfalls When Using the DataStax Java Driver
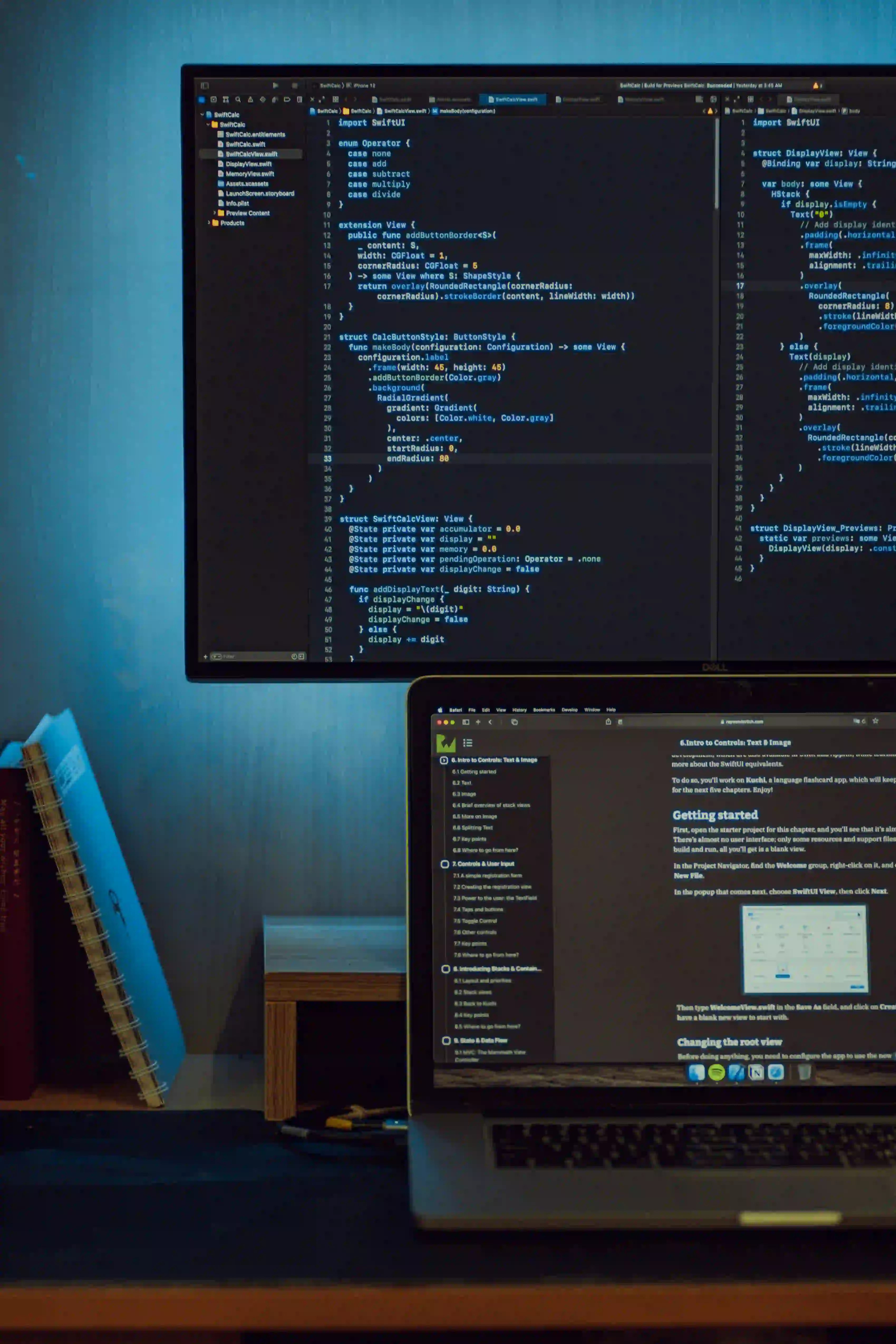
Common Pitfalls When Using the DataStax Java Driver
The DataStax Java Driver is a powerful tool for connecting and interacting with Apache Cassandra databases. Although it offers a robust API for database operations, developers can fall into common pitfalls that affect application performance, reliability, and maintainability. In this blog post, we will explore these pitfalls and provide practical solutions to help you navigate the complexities of using the DataStax Java Driver effectively.
Table of Contents
- Introduction
- Understanding the DataStax Java Driver
- Common Pitfalls
-
- Improper Connection Management
-
- Ignoring Prepared Statements
-
- Failing to Configure Timeouts
-
- Not Utilizing Connection Pooling
-
- Skipping Error Handling
-
- Conclusion
- Additional Resources
Understanding the DataStax Java Driver
The DataStax Java Driver is designed to facilitate interactions with Cassandra in a way that is efficient and easy to use. It provides features such as connection pooling, asynchronous API calls, and comprehensive error handling. However, like any tool, understanding its nuances is crucial to prevent issues down the line.
The following example demonstrates a simple connection using the Java Driver:
import com.datastax.oss.driver.api.core.CqlSession;
public class CassandraConnection {
public static void main(String[] args) {
try (CqlSession session = CqlSession.builder().build()) {
// Connection to Cassandra has been established.
System.out.println("Connected to Cassandra!");
} catch (Exception e) {
System.err.println("Connection failed: " + e.getMessage());
}
}
}
In this code, we create a CqlSession
object, which establishes a connection to Cassandra. But if not managed correctly, this can lead to serious problems.
Common Pitfalls
1. Improper Connection Management
One of the most critical mistakes is not managing connections wisely. Each time you create a new session, you incur overhead. It is essential to avoid frequently opening and closing connections, as this can lead to resource exhaustion.
Solution: Use a single CqlSession
instance throughout your application.
public class CassandraConnection {
private static final CqlSession session;
static {
session = CqlSession.builder().build();
}
public static CqlSession getSession() {
return session;
}
public static void closeConnection() {
session.close();
}
}
This code snippet creates a singleton session that remains open for the application's lifecycle. This design pattern reduces overhead and improves performance.
2. Ignoring Prepared Statements
Another frequent downfall is bypassing prepared statements. When you execute queries directly by concatenating strings, you expose your application to SQL injection risks and may suffer from performance penalties due to query parsing.
Solution: Always use prepared statements when executing queries:
String preparedQuery = "INSERT INTO users (id, name) VALUES (?, ?)";
PreparedStatement preparedStatement = session.prepare(preparedQuery);
BoundStatement boundStatement = preparedStatement.bind(UUID.randomUUID(), "John Doe");
session.execute(boundStatement);
In this example, the prepared statement mitigates the risk of SQL injection and optimizes query execution.
3. Failing to Configure Timeouts
Timeouts can make or break an application. Not configuring timeouts can lead to your application hanging indefinitely if there is a slow network or the database goes down.
Solution: Always specify timeouts in your session configuration:
CqlSession session = CqlSession.builder()
.withLocalDatacenter("datacenter1")
.withRequestTimeout(Duration.ofSeconds(10)) // Set request timeout
.build();
By setting a request timeout, you can ensure that your application only waits for a specified duration before throwing an error, enabling you to handle issues gracefully.
4. Not Utilizing Connection Pooling
The Java Driver has built-in connection pooling that can be easily overlooked. Not taking advantage of this feature can lead to performance bottlenecks, especially under heavy load.
Solution: Leverage connection pooling through configuration:
CqlSession session = CqlSession.builder()
.withPoolingOptions(new PoolingOptions()
.setMaxRequestsPerConnection(HostDistance.LOCAL, 2) // Max requests per connection
.setMaxConnectionsPerHost(HostDistance.LOCAL, 2)) // Max connections to local hosts
.build();
With these settings, you can fine-tune how many requests and connections you maintain, ensuring efficient resource usage.
5. Skipping Error Handling
Lastly, ignoring error handling is like sailing a ship without a compass. Errors are inevitable in distributed systems. Without appropriate handling, your application may crash or produce incorrect results unexpectedly.
Solution: Implement robust error handling everywhere:
try {
session.execute(boundStatement);
} catch (Exception e) {
// Log and handle the error appropriately
System.err.println("Execution failed: " + e.getMessage());
}
By handling exceptions, you ensure your application remains robust and resilient to errors.
My Closing Thoughts on the Matter
Navigating the DataStax Java Driver can be complex, but avoiding these common pitfalls can lead your project to success. By managing connections wisely, utilizing prepared statements, configuring timeouts, leveraging connection pooling, and implementing error handling, you can build a stable and efficient application.
For further information on the DataStax Java Driver, consider checking the DataStax documentation or the Cassandra Best Practices guide for optimization techniques.
By applying these best practices, you’ll be well on your way to creating scalable and maintainable Java applications with DataStax. Happy coding!