Overcoming Challenges in Student Data Persistence with Spring
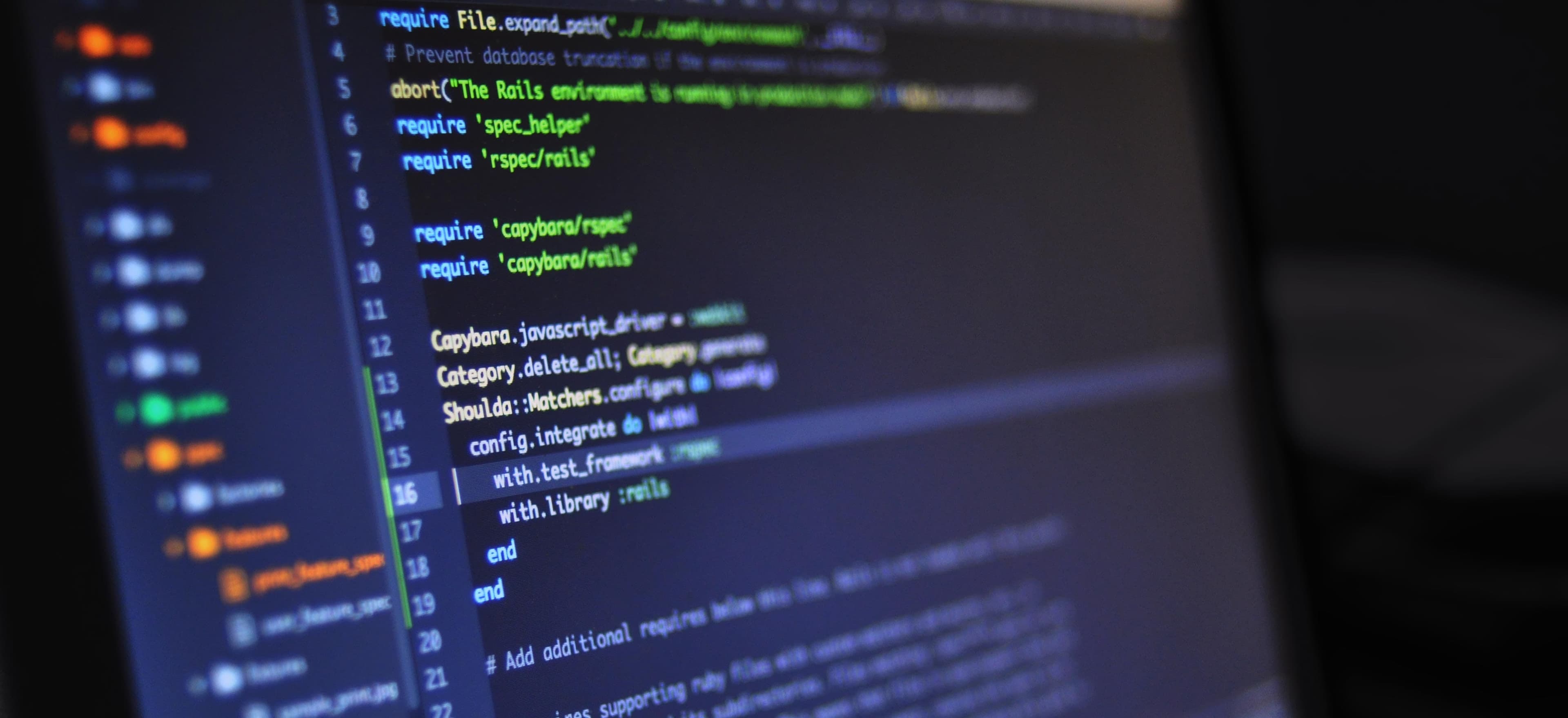
- Published on
Overcoming Challenges in Student Data Persistence with Spring
In today's educational landscape, managing student data efficiently is crucial for institutions. With the exponential growth of data, the challenge of maintaining integrity, persistence, and accessibility of this information can be daunting. Enter Spring Framework – a powerful ally in addressing these challenges. In this blog post, we will explore how Spring can facilitate effective student data persistence.
Understanding Data Persistence
Data persistence refers to the ability of an application to store data in a manner that it can be retrieved even after the application is terminated. In the context of student data, this involves handling everything from student records, grades, attendance, and much more.
However, data persistence is not without challenges. Institutions often struggle with:
- Data Integrity: Ensuring that the data is accurate and consistent.
- Scalability: Ability to handle increasing amounts of data.
- Security: Safeguarding sensitive student information.
- Performance: Fast retrieval and processing of data.
Why Choose Spring for Data Persistence
Spring Framework is widely used due to its comprehensive ecosystem that supports various data operations. Its features like Dependency Injection (DI), Aspect-Oriented Programming (AOP), and strong integration capabilities with databases make it an ideal choice for building robust back-end applications.
Set Up Your Spring Project
To start with, let's set up a Spring Boot project for managing student data. We'll use Spring Data JPA, an abstraction layer over JPA (Java Persistence API), to handle data operations easily.
- Project Dependency Management: Include the required dependencies in your
pom.xml
if you are using Maven:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
Explanation
- Spring Boot Starter Data JPA: This dependency simplifies data management and integrates with various JPA providers.
- Spring Boot Starter Web: It helps build web applications using Spring MVC.
- H2 Database: A lightweight in-memory database perfect for development and testing.
Configuring Application Properties
Next, we need to configure our application properties. Open application.properties
and add the following:
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
spring.h2.console.enabled=true
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
Explanation
- Datasource Configuration: We set up an in-memory H2 database for testing purposes.
- H2 Console: This enables us to access the H2 database console for direct queries.
- Hibernate: Automatic schema generation and SQL logging features help in debugging.
Creating the Student Entity
Create a Student
entity that represents our student data.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
@Entity
public class Student {
@Id
@GeneratedValue
private Long id;
private String name;
private String email;
// Constructors, Getters and Setters
public Student() {}
public Student(String name, String email) {
this.name = name;
this.email = email;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
Explanation
- Entity Annotation: This designates the class as a JPA entity which maps to a database table.
- Id and GeneratedValue: It defines a primary key (id) and an auto-incrementing value which simplifies ID management.
Creating a Repository for Student Data
Now, we need to create a repository interface to handle the data operations relating to the Student
entity.
import org.springframework.data.jpa.repository.JpaRepository;
public interface StudentRepository extends JpaRepository<Student, Long> {
// Additional custom queries can be defined here
}
Explanation
- JpaRepository: It provides built-in CRUD operations for the
Student
entity without implementing any methods. It saves you from writing boilerplate code.
Service Layer for Business Logic
To keep our application scalable and maintainable, we will create a service that handles business logic.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class StudentService {
private final StudentRepository studentRepository;
@Autowired
public StudentService(StudentRepository studentRepository) {
this.studentRepository = studentRepository;
}
public List<Student> getAllStudents() {
return studentRepository.findAll();
}
public Student saveStudent(Student student) {
return studentRepository.save(student);
}
}
Explanation
- Service Annotation: It marks the class as a service provider, making it eligible for Spring’s component scanning.
- Constructor Injection: It promotes immutability and is recommended over field injection for testability.
Building the REST Controller
Finally, we will build a REST controller to expose the student data endpoints.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/students")
public class StudentController {
private final StudentService studentService;
@Autowired
public StudentController(StudentService studentService) {
this.studentService = studentService;
}
@GetMapping
public List<Student> getAllStudents() {
return studentService.getAllStudents();
}
@PostMapping
public ResponseEntity<Student> createStudent(@RequestBody Student student) {
Student savedStudent = studentService.saveStudent(student);
return new ResponseEntity<>(savedStudent, HttpStatus.CREATED);
}
}
Explanation
- RestController: This annotation indicates that the class is a controller and each method will yield JSON responses.
- Request Mapping: Each endpoint is mapped to a specific URL. We use
@GetMapping
for fetching data and@PostMapping
for creating new records.
Testing Your Application
You can run your Spring Boot application with the command:
mvn spring-boot:run
Access the H2 database console at http://localhost:8080/h2-console
using the credentials specified in application.properties
.
To interact with the REST APIs, tools like Postman or Insomnia can be useful. You can fetch students with a GET request at http://localhost:8080/api/students
and create a new student with a POST request to the same endpoint, including a JSON body.
Overcoming Common Challenges
-
Handling Relationships: In a real-world scenario, students may have many-to-many relationships with courses. Utilizing JPA’s annotation aids like
@ManyToMany
can effectively manage these associations. -
Transaction Management: Spring provides declarative transaction management, mainly through the
@Transactional
annotation, to manage data consistency. -
Caching: With the use of Spring Cache, frequently accessed student data can be stored temporarily to improve application performance.
-
Security: Integrating Spring Security can protect sensitive student data and provide user authentication effectively.
To Wrap Things Up
Handling student data persistence with Spring Framework can significantly simplify complex data operations and improve maintainability. By leveraging Spring Data JPA and structuring your applications correctly, you can create efficient, secure, and scalable solutions.
For more in-depth reading, consider exploring the following links:
With Spring, you can focus on building the core application logic while it takes care of the repetitive tasks of data management. Happy coding!
Checkout our other articles