Mastering Package-Private Error Tracking in Java
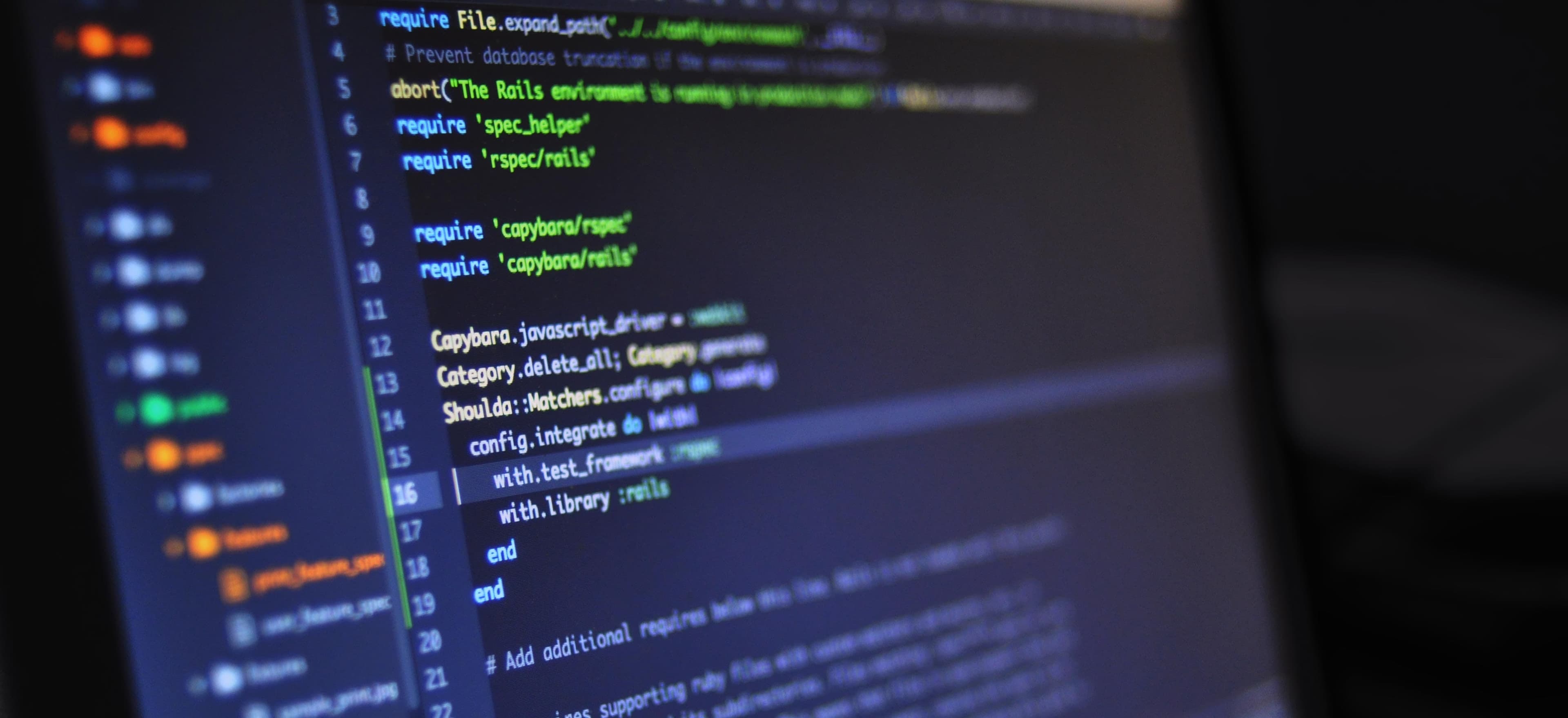
- Published on
Mastering Package-Private Error Tracking in Java
When developing applications in Java, managing errors and exceptions efficiently is crucial for maintaining code quality and enhancing user experience. In this blog post, we will explore a lesser-discussed aspect of error handling: package-private error tracking. This approach offers a blend of encapsulation and transparency that can significantly improve your Java applications.
Understanding Package-Private Access in Java
In Java, access modifiers play an essential role in encapsulating data and enforcing access control. The four access modifiers are:
- Public: Accessible from any other class.
- Protected: Accessible within the same package and from subclasses.
- Private: Accessible only within the defined class.
- Package-private (also known as default): Accessible only within classes that belong to the same package.
By using package-private access, we can share functions and data among classes within the same package while keeping them hidden from the outside world. This type of access comes in handy when developing utility classes or libraries where you want to ensure that certain functionalities are used only within the designated package.
Why Use Package-Private Error Tracking?
Package-private error tracking offers several advantages:
-
Encapsulation: Only classes within the package can access the error tracking mechanism, preventing unwanted interference from external classes.
-
Modularity: By segmenting error handling within packages, you maintain a cleaner structure, which is easier to manage and test.
-
Control: You can define error handling strategies that make sense for a particular module without exposing those strategies globally.
-
Maintainability: It reduces the complexity of managing throwables across the application, especially in large projects.
With this context in mind, let’s delve into how to implement package-private error tracking effectively in Java applications.
Implementing Package-Private Error Tracking
Step 1: Define an Exception Class
Creating a custom exception class offers you the ability to distinguish between different types of exceptions that might be raised within your package.
// src/com/example/errors/PackagePrivateException.java
package com.example.errors;
class PackagePrivateException extends Exception {
public PackagePrivateException(String message) {
super(message);
}
}
Why: By defining a custom exception, you gain the flexibility to handle errors in a way that is meaningful for your particular context.
Step 2: Error Tracking Utility
Now we create a utility class for tracking errors. This class will log errors to the console or any logging framework you might prefer.
// src/com/example/errors/ErrorTracker.java
package com.example.errors;
import java.util.logging.Level;
import java.util.logging.Logger;
class ErrorTracker {
private static final Logger logger = Logger.getLogger(ErrorTracker.class.getName());
static void logError(PackagePrivateException e) {
logger.log(Level.SEVERE, e.getMessage(), e);
}
}
Why:
Using the Logger
class from the java.util.logging
package allows you to control and format your logging output as needed. Since this class is package-private, it ensures that error logging remains encapsulated.
Step 3: Implement Error Tracking in Your Application Logic
Next, integrate the error-tracking utility within your business logic to manage exceptions effectively.
// src/com/example/service/BusinessService.java
package com.example.service;
import com.example.errors.ErrorTracker;
import com.example.errors.PackagePrivateException;
class BusinessService {
public void performOperation() {
try {
// Simulate operations
int result = riskyOperation();
} catch (PackagePrivateException e) {
ErrorTracker.logError(e);
}
}
private int riskyOperation() throws PackagePrivateException {
// Simulating an exception
throw new PackagePrivateException("An error occurred in riskyOperation.");
}
}
Why:
This example encapsulates the error handling logic within the BusinessService
, making it easier to manage errors that arise during operations. Should an exception occur, it is logged through the ErrorTracker
, allowing for easy identification and debugging.
Step 4: Unit Testing Package-Private Error Tracking
When developing, it's critical to test your error-tracking mechanism to ensure that it logs the right information.
// src/com/example/service/BusinessServiceTest.java
package com.example.service;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertThrows;
class BusinessServiceTest {
@Test
void testPerformOperationLogsError() {
BusinessService businessService = new BusinessService();
// Expected to throw an exception
assertThrows(PackagePrivateException.class, () -> businessService.performOperation());
}
}
Why:
This JUnit test ensures that the performOperation
method behaves as expected under erroneous conditions. It verifies that your error tracking is functioning and the errors are being logged.
Moving to the Next Level: Integrating with a Framework
While the above demonstrates a custom approach to error tracking, consider integrating it with a framework like Spring or Jakarta EE for more robust features. For instance, Spring offers an excellent way to handle exceptions using aspects or controllers.
Key Takeaways
Incorporating package-private error tracking into your Java applications is not just about encapsulation—it's about creating a controlled and manageable way of dealing with errors that enhances maintainability and readability. With this approach, you can build a foundation for developing robust Java applications that can handle errors gracefully.
For more information on Java logging, check out the Java Logging Documentation. Additionally, learn how exception handling works in Java with the tutorial available at Java Exception Handling.
By mastering these techniques, you'll be well on your way to crafting elegant and efficient Java applications. Happy coding!
Checkout our other articles