Unlocking the Power of Reflection for Java Annotations
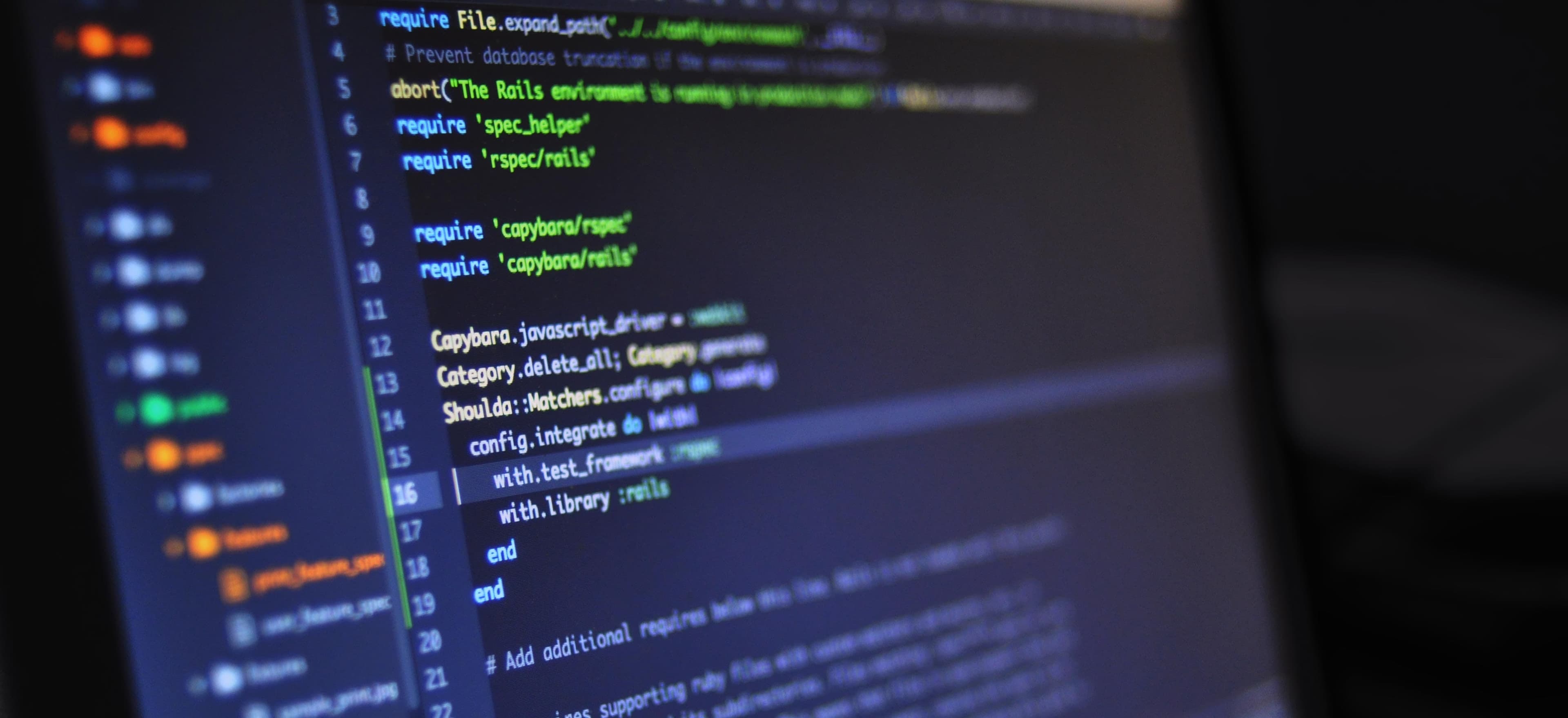
- Published on
Unlocking the Power of Reflection for Java Annotations
Java annotations are a powerful feature that provides metadata about the code without modifying it. When combined with reflection, these annotations gain a whole new level of usability, enabling developers to write more dynamic, maintainable, and reusable code. In this blog post, we will explore how to effectively utilize reflection to manage annotations, enhancing your Java applications' capabilities.
What are Annotations?
Annotations in Java are defined using the @interface
keyword. They allow developers to embed descriptive information within their code. For instance, you can use annotations to define configurations, document code behavior, or specify parameters for frameworks and libraries.
Here is a simple example of creating and using a custom annotation:
@interface MyCustomAnnotation {
String value();
int number();
}
@MyCustomAnnotation(value = "Hello, Annotations!", number = 42)
public class AnnotatedClass {
}
In the code above, MyCustomAnnotation
is defined with two elements, value
and number
. The AnnotatedClass
uses this annotation, allowing us to attach metadata directly to the class.
Establishing the Context to Reflection
Reflection is a feature in Java that allows you to inspect classes, interfaces, fields, and methods at runtime, regardless of their visibility. It provides the ability to manipulate objects and invoke methods dynamically.
The power of reflection becomes even more apparent when paired with annotations. By utilizing reflection, you can extract annotations and their values programmatically, allowing your application to adapt its behavior based on the presence of certain metadata.
Accessing Annotations via Reflection
To access annotations using reflection, you can follow these steps:
- Obtain the
Class
object of the annotated class. - Check if the annotation is present using the
isAnnotationPresent
method. - Retrieve the annotation using
getAnnotation
.
Here’s how you can implement this:
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.RUNTIME)
@interface MyCustomAnnotation {
String value();
int number();
}
@MyCustomAnnotation(value = "Hello, Annotations!", number = 42)
public class AnnotatedClass {
}
public class AnnotationProcessor {
public static void main(String[] args) {
Class<AnnotatedClass> obj = AnnotatedClass.class;
if (obj.isAnnotationPresent(MyCustomAnnotation.class)) {
MyCustomAnnotation annotation = obj.getAnnotation(MyCustomAnnotation.class);
System.out.println("Value: " + annotation.value());
System.out.println("Number: " + annotation.number());
}
}
}
Why Use Reflection with Annotations?
-
Dynamic Behavior: Reflection allows you to create more dynamic applications. Instead of hardcoding configurations, you can specify behavior using annotations and read them at runtime.
-
Separation of Concerns: By employing annotations, you can separate the configuration/behavior from the actual code logic. This approach makes it easier to maintain and understand the code.
-
Integration with Frameworks: Many Java frameworks use annotations extensively. For example, Spring and JPA leverage annotations to manage application configurations and ORM mappings, respectively. Understanding reflection can help developers utilize these frameworks more effectively.
Practical Use Case: Custom Logger
Let’s elaborate a practical example where we develop a simple logging mechanism powered by annotations and reflection.
Step 1: Creating a Custom Annotation
First, we create a logging annotation:
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
@interface LogExecutionTime {
}
This annotation can be applied to methods, and it will provide metadata indicating that we want to log the method execution time.
Step 2: Implementing Aspect Logic
Now, we’ll create an aspect to handle this annotation using reflection:
import java.lang.reflect.Method;
public class Logger {
public static void logExecutionTime(Object obj) {
Method[] methods = obj.getClass().getDeclaredMethods();
for (Method method : methods) {
if (method.isAnnotationPresent(LogExecutionTime.class)) {
long startTime = System.currentTimeMillis();
try {
method.invoke(obj);
} catch (Exception e) {
e.printStackTrace();
}
long endTime = System.currentTimeMillis();
System.out.println("Execution time of " + method.getName() + ": " + (endTime - startTime) + " ms");
}
}
}
}
Step 3: Using the Logger
Now, let’s create a class that utilizes our logging feature:
public class MyService {
@LogExecutionTime
public void serve() {
// Simulating some processing time
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
@LogExecutionTime
public void serveAnother() {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class Main {
public static void main(String[] args) {
MyService service = new MyService();
Logger.logExecutionTime(service);
service.serve();
service.serveAnother();
}
}
Explanation of the Code
In the Logger
class, we check each method of the provided object. If a method is marked with @LogExecutionTime
, we record the current system time before and after invoking the method to calculate its execution time.
This simple logging mechanism enhances our service class by providing insights into method performance without cluttering the method definitions themselves.
In Conclusion, Here is What Matters
In conclusion, the combination of reflection and annotations opens the gateway to versatile programming techniques in Java. By leveraging these tools, you can improve code readability, maintainability, and allow for dynamic behavior, ultimately enhancing the quality of your applications.
For further reading, check out the Oracle Java Documentation on Reflection and Java Annotations Tutorial for deeper insights into these topics.
By strategically using reflection and annotations in your own Java applications, you're not just following a trend; you're enhancing your programming toolkit. Happy coding!
Checkout our other articles