Troubleshooting Common Netty Encoder/Decoder Issues
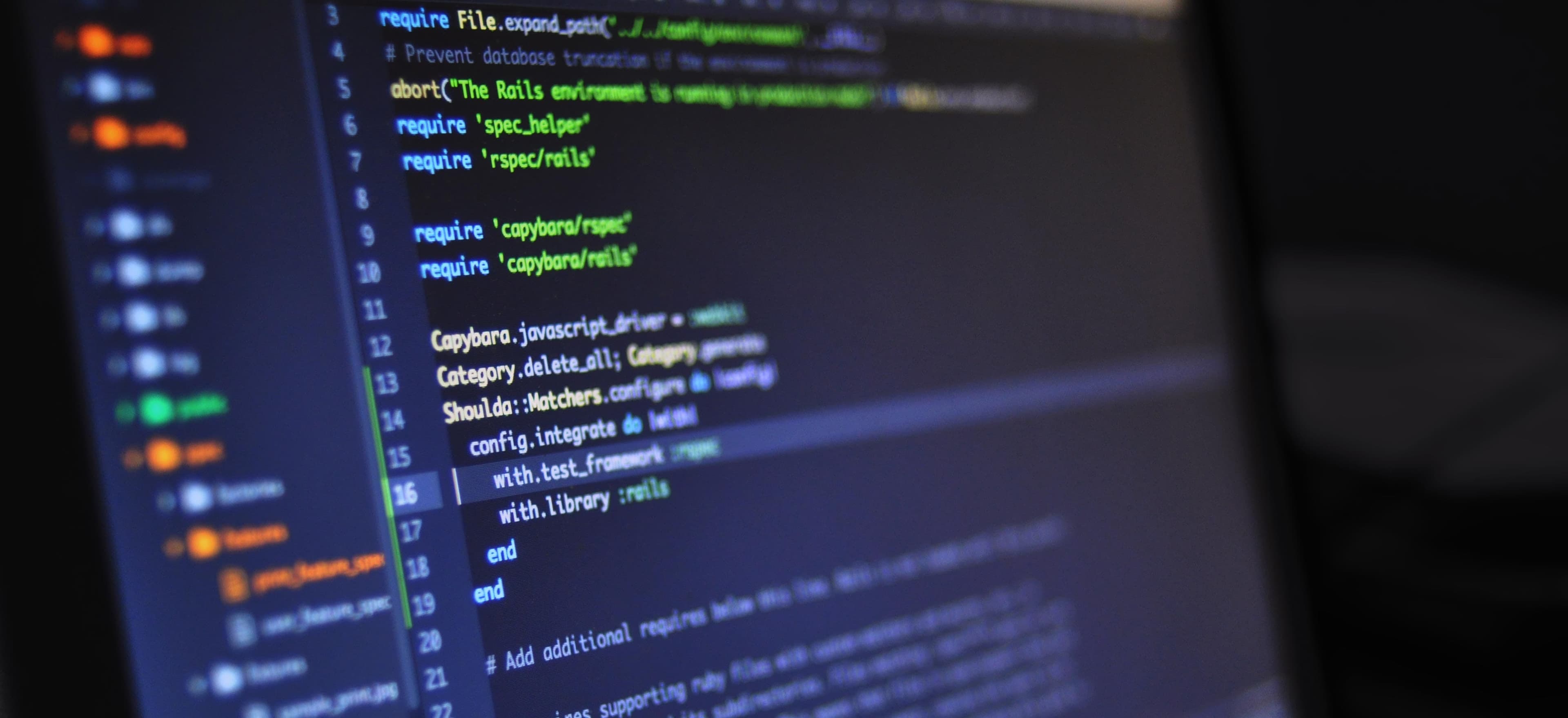
- Published on
Troubleshooting Common Netty Encoder/Decoder Issues
In the realm of network programming, Netty has emerged as a powerhouse framework for developing asynchronous event-driven network applications. Whether you're building web servers, chat applications, or any form of client-server communication, understanding how to troubleshoot the common issues with encoders and decoders in Netty is essential.
In this post, we will delve into common encoder/decoder issues you might encounter while working with Netty. We will provide clear examples and explanations to help you resolve these issues effectively, all while adhering to best practices.
Understanding Netty's Encoders and Decoders
Before diving into troubleshooting, let's clarify what encoders and decoders do in Netty. The main purpose of encoders is to transform outgoing messages into a format suitable for transmission, while decoders convert incoming bytes back into the original messages.
This duality is crucial for ensuring that data can be sent over the network efficiently and correctly, adapting to different protocols as needed.
Common Issues with Encoders and Decoders
1. Data Format Mismatch
One of the most common issues arises when the data format expected by the receiver does not match the format produced by the sender. This might occur due to changes in message structures or misunderstanding of protocols.
Example
Consider the scenario where you're sending JSON data. If the encoder outputs JSON strings while the decoder expects Java objects, the communication will inevitably break.
Encoder Code:
public class JsonEncoder extends MessageToByteEncoder<MyMessage> {
@Override
protected void encode(ChannelHandlerContext ctx, MyMessage msg, ByteBuf out) throws Exception {
String json = new ObjectMapper().writeValueAsString(msg);
byte[] data = json.getBytes(StandardCharsets.UTF_8);
out.writeBytes(data);
}
}
Decoder Code:
public class JsonDecoder extends ByteToMessageDecoder {
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List<Object> out) throws Exception {
byte[] data = new byte[in.readableBytes()];
in.readBytes(data);
String json = new String(data, StandardCharsets.UTF_8);
MyMessage msg = new ObjectMapper().readValue(json, MyMessage.class);
out.add(msg);
}
}
Commentary:
- The
JsonEncoder
convertsMyMessage
objects into a JSON string before sending them through the channel. - The
JsonDecoder
reads incoming bytes and converts them back intoMyMessage
objects. - Why it's important: If the JSON structure or field names change, deserialization will fail.
2. Buffer Overflows
Buffer overflows occur when the amount of data received exceeds the buffer's capacity. This often leads to IndexOutOfBoundsException
errors.
How to Avoid
To prevent buffer overflows, ensure that your decoder handles data efficiently. You can check if the buffer has enough readable bytes before attempting to read from it.
Revised Decoder Code:
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List<Object> out) throws Exception {
if (in.readableBytes() < expectedSize) {
return; // Not enough bytes to read
}
// Read your data - process as needed
}
Commentary:
- Always check the size of the inbound ByteBuf before trying to read from it.
- Why it's important: This prevents runtime exceptions and increases application stability.
3. Inconsistent State Management
When using multiple encoders and decoders, maintain consistent state across handlers. Failing to do so can lead to dropped messages or missing data.
Example
If you have an encoder that maintains an internal state to create a message but the existing state is not updated or is modified elsewhere, this can lead to corrupted data.
Stateful Encoder Example:
public class StatefulEncoder extends MessageToByteEncoder<MyMessage> {
private int messageCount = 0;
@Override
protected void encode(ChannelHandlerContext ctx, MyMessage msg, ByteBuf out) throws Exception {
messageCount++;
// Encode using messageCount for tracking
}
}
Commentary:
- If
messageCount
is modified elsewhere, it can produce unpredictable behavior in message encoding. - Why it's important: Maintain encapsulation of your encoder's state to prevent state corruption.
4. Incorrect Message Flow
Issues can arise when messages sent through the pipeline aren’t handled in the correct order or never reach the intended destination.
Prevention Techniques
- Pipeline Configuration: Ensure that handlers in your channel pipeline are configured in the correct sequence.
- Logging: Implement logging on both encoding and decoding paths to identify failures or bottlenecks.
Logging Example:
public class LoggingEncoder extends MessageToByteEncoder<MyMessage> {
@Override
protected void encode(ChannelHandlerContext ctx, MyMessage msg, ByteBuf out) throws Exception {
logger.info("Encoding message: " + msg);
// Add encoding logic
}
}
Commentary:
- Incorporate logging to have visibility into message flows, which can help uncover where messages are lost.
- Why it's important: Monitoring message flow can reveal hidden issues that disrupt communication.
5. Testing with Different Payload Sizes
Sometimes, your application might work flawlessly for small messages but fail for larger ones due to increased complexity in serialization/deserialization processes.
Recommendations
- Unit Tests: Always perform unit tests using various payload sizes to identify critical failures.
- Load Testing: Implement load tests to see how the system behaves under stress.
@Test
public void testLargeMessageEncoding() {
MyMessage largeMsg = new MyMessage(...); // Populate with large data
ByteBuf encoded = encoder.encode(...);
assertNotNull(encoded); // Ensure encoding is successful
}
Commentary:
- It's essential to look beyond typical use cases during testing.
- Why it's important: Prevent surprises in production where situations can differ significantly from testing environments.
The Bottom Line
Understanding how to troubleshoot common encoder and decoder issues in Netty is paramount for developers working on network applications. This guide outlined key problems, provided sample code, and emphasized best practices to ensure stable communication between client and server.
By maintaining proper etiquette in data formats, carefully managing buffer sizes, maintaining state, tracking message flow, and performing rigorous testing, you can significantly reduce downtime and enhance application performance.
For further reading, you may find Netty's Official Documentation useful, as it provides detailed insights into managing various Netty components. Additionally, check out the JSON Handling with Jackson tool to improve your skills with JSON data formats.
Implement these practices today and transform your Netty applications into robust, fault-tolerant systems!