Common Pitfalls of Getters and Setters in Programming
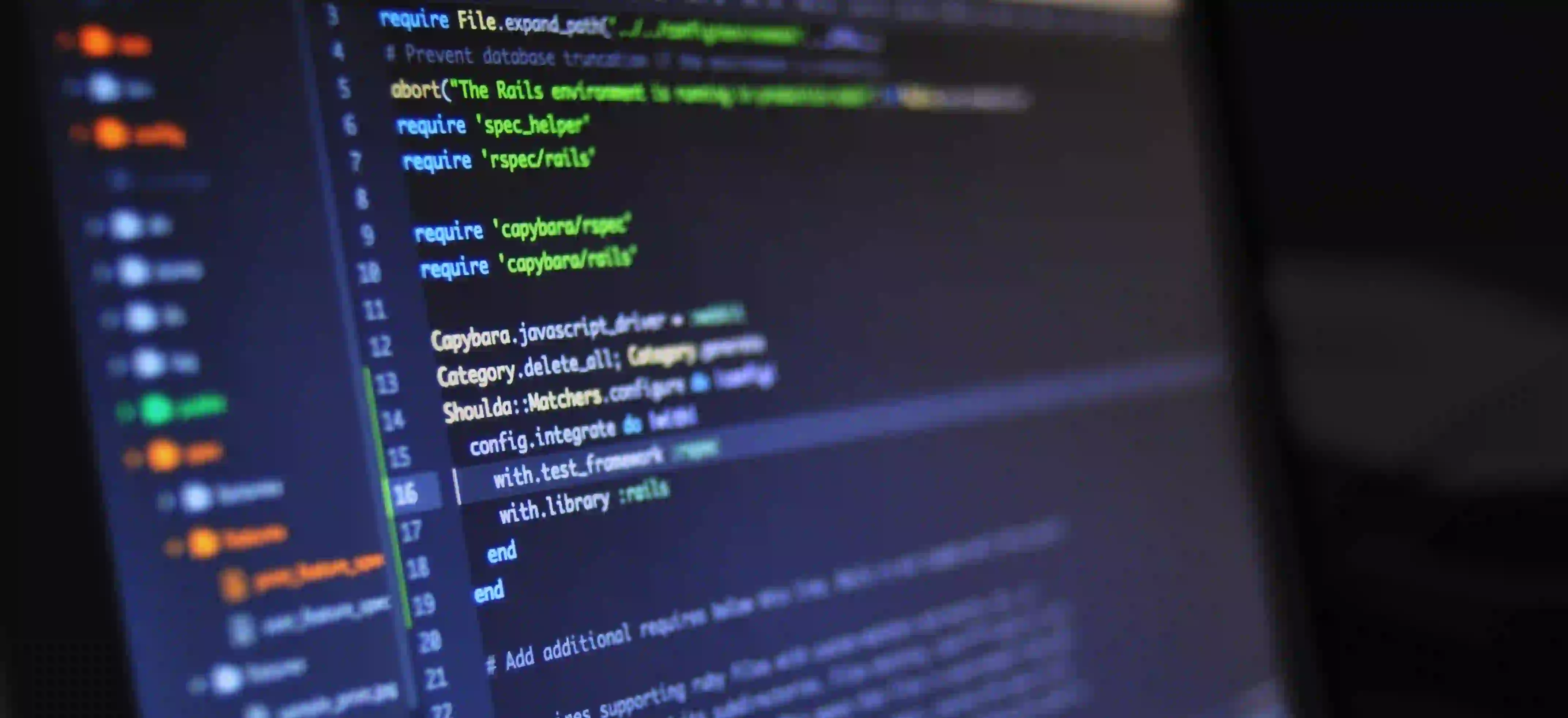
Common Pitfalls of Getters and Setters in Programming
Getters and setters—those ubiquitous methods in object-oriented programming (OOP)—are often touted as best practices for accessing and modifying private instance variables. While they provide a layer of abstraction, there is considerable debate surrounding their use. This blog post will help you better understand the common pitfalls of getters and setters in programming, particularly in Java.
By the end of this article, you will glean insights into how to approach encapsulation effectively, thus promoting code that is not only functional but also maintainable and readable.
What Are Getters and Setters?
Getters and setters are methods employed to retrieve (get) and modify (set) the values of private attributes of a class. These methods usually follow a common naming convention: the getter method is prefixed with 'get' followed by the attribute name, and the setter method is prefixed with 'set'.
Here's a simple Java example:
public class Person {
private String name;
private int age;
// Getter for name
public String getName() {
return name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
// Getter for age
public int getAge() {
return age;
}
// Setter for age
public void setAge(int age) {
if (age >= 0) { // Validation
this.age = age;
} else {
throw new IllegalArgumentException("Age cannot be negative.");
}
}
}
While this implementation seems neat and organized, it's essential to consider the broader implications.
Common Pitfalls
1. Overusing Getters and Setters
Getters and setters can lead to overexposure of class fields, diluting the benefits of encapsulation. Consider the Person
class above. By providing public access to all fields, you're essentially exposing the internal state of the object directly.
Instead of using verbose getters and setters, you might want to create more meaningful behaviors. For instance:
public class Person {
private String name;
private int age;
public void celebrateBirthday() {
age++;
}
}
This way, the internal state is modified through a controlled mechanism, which is what encapsulation is all about.
2. Setters Without Validation
Many developers neglect validation logic in their setters. This is particularly problematic in maintainable code.
Consider our earlier age setter:
public void setAge(int age) {
this.age = age; // No validation
}
This opens the door to illogical states. Make sure to always validate inputs within setters:
public void setAge(int age) {
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative.");
}
this.age = age;
}
3. Making Setters Too Complex
Setters should generally be simple. Adding complex logic in these methods can lead to unexpected behaviors. For example:
public void setName(String name) {
// Complex logic here, such as logging, notifying others, etc.
// Not good practice
}
Such complexity defeats the purpose of a simple setter. Keep logic separate and succinct. If you find yourself adding multiple lines of code, consider using a designated method.
4. Lack of Immutability
In Java, immutability is a powerful abstraction principle. By using getters and setters, you may inadvertently convey that certain objects can be modified when they shouldn't be.
For example, if you have an Address
class, consider making it immutable:
public final class Address {
private final String street;
private final String city;
public Address(String street, String city) {
this.street = street;
this.city = city;
}
public String getStreet() {
return street;
}
public String getCity() {
return city;
}
}
With this design, you protect the integrity of the Address
object, ensuring its state cannot be altered after creation.
5. Exposing Internal Representation
Getters and setters often expose the internal representation of a class. This can introduce tight coupling between classes, making it harder to refactor or change the internals later.
For instance:
public class Product {
private List<String> tags;
public List<String> getTags() {
return tags;
}
}
This allows client code to manipulate the tags list directly, which might lead to inconsistent states. Instead, consider providing methods to add or remove tags:
public void addTag(String tag) {
tags.add(tag);
}
public void removeTag(String tag) {
tags.remove(tag);
}
6. Assume Object Variety
Assuming all attributes need both getters and setters can lead to unnecessary complexity. Always ask yourself: “Is this attribute likely to change?”
For example, in a DateOfBirth
class, exposing a setter may not be appropriate:
public final class DateOfBirth {
private final LocalDate date;
public DateOfBirth(LocalDate date) {
this.date = date;
}
public LocalDate getDate() {
return date;
}
}
This reinforces the concept of representing an important aspect of the domain in a robust manner, ensuring values won’t inadvertently change.
7. Ignoring Thread Safety
In a multithreaded environment, the absence of synchronization in getters and setters can lead to unpredictable behavior.
You can use synchronized methods:
public synchronized void setAge(int age) {
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative.");
}
this.age = age;
}
Or you can explore other concurrency control techniques. This is particularly crucial when you expect multiple threads to interact with the object.
Key Takeaways
Getters and setters can help in encapsulating the data of a class, but their misuse can lead to many programming pitfalls. By being aware of these issues, you'll improve the maintainability and clarity of your code.
It's always important to remember that while these methods can be useful commodities in your coding toolkit, they should not become the crutch you lean on without question. Thoughtful consideration of your class design will yield better, more robust code.
Additional Resources
For a deeper dive into object-oriented programming principles, check out Effective Java by Joshua Bloch and Java Concurrency in Practice by Brian Goetz.
Your journey in Java will be markedly fruitful if you embrace these best practices, turning potential pitfalls into rich opportunities for creating a mature and scalable codebase. Happy coding!