Optimizing RabbitMQ for Real-Time Messaging Performance
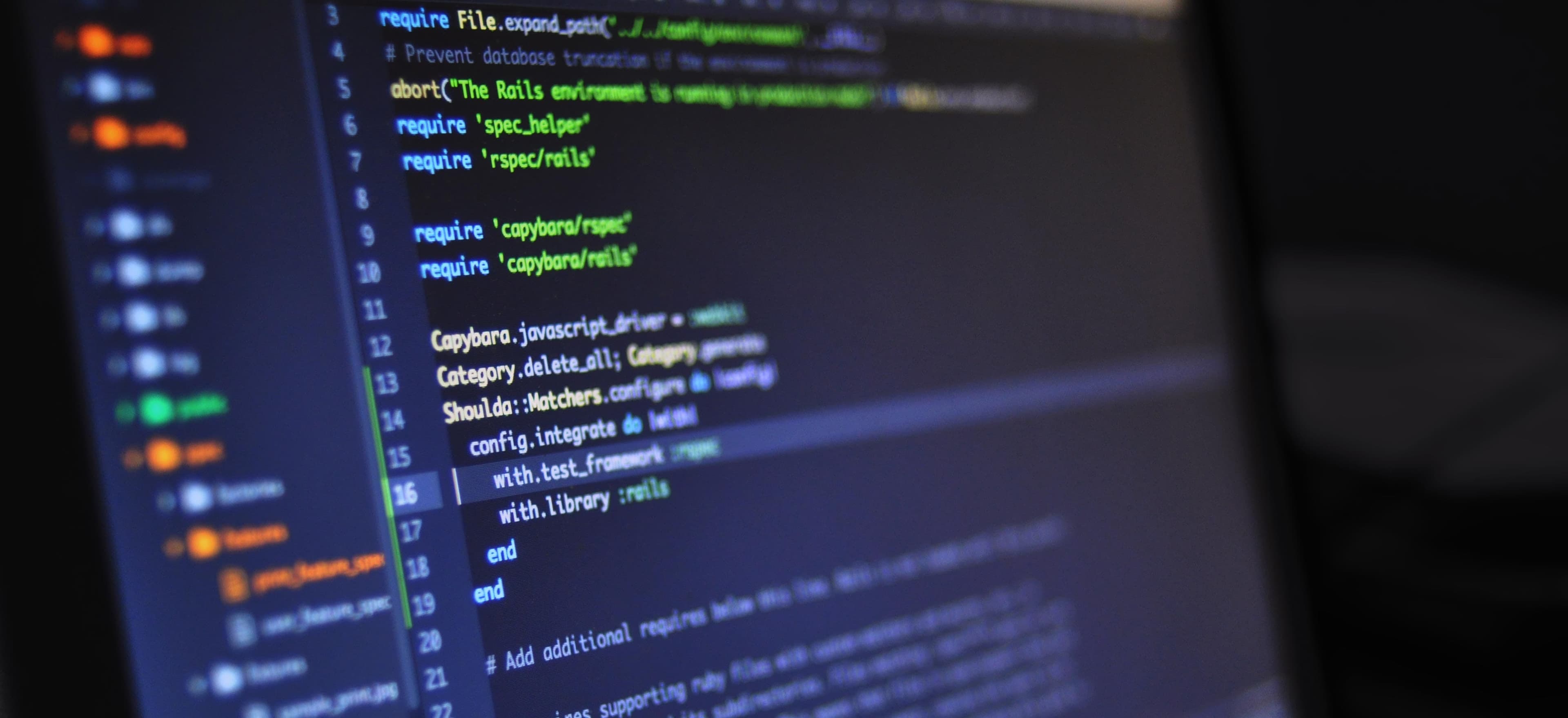
- Published on
Optimizing RabbitMQ for Real-Time Messaging Performance
RabbitMQ is one of the most popular open-source message brokers that supports multiple messaging protocols, ensuring seamless communication in distributed systems. However, achieving real-time performance is crucial for applications such as chat systems, stock price notifications, and live data feeds. In this blog post, we will delve into strategies for optimizing RabbitMQ to meet real-time messaging demands, presenting code snippets and configurations for practical understanding.
Understanding RabbitMQ and Real-Time Messaging
RabbitMQ leverages a producer-consumer model, allowing applications to send and receive messages via message queues. For real-time messaging, you should focus on low latency, high throughput, and fault tolerance.
Real-time messaging generally requires:
- Low Latency: The time taken from sending a message to its consumption.
- High Throughput: The ability to process a large number of messages per second.
- Reliability: Ensuring messages are not lost during transmission or processing.
Let's get into the performance optimizations you can implement to enhance RabbitMQ functionality.
1. Choosing the Right Message Acknowledgments
By default, RabbitMQ performs message acknowledgment on the consumer side, ensuring that messages are only removed from the queue once they’re processed successfully. However, for optimal real-time performance, consider the following acknowledgment strategies:
Manual Acknowledgments
Using manual acknowledgments allows control over when a message is acknowledged.
import com.rabbitmq.client.*;
public class ManualAckConsumer {
private final static String QUEUE_NAME = "test_queue";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
channel.basicConsume(QUEUE_NAME, false, (consumerTag, message) -> {
String msg = new String(message.getBody(), "UTF-8");
System.out.println("Received: " + msg);
// Process the message here
// After processing:
channel.basicAck(message.getEnvelope().getDeliveryTag(), false);
}, consumerTag -> { });
}
}
}
Why Use Manual Acknowledgments?
This strategy allows you to delay message acknowledgment until the message is fully processed, preventing the loss of messages if an error occurs. However, ensure that you manage message acknowledgments carefully to avoid unnecessary delays.
2. Prefetch Count Configuration
The prefetch count determines how many messages can be sent to a consumer before it has to acknowledge messages. A low prefetch count increases latency, while a high count can overwhelm a consumer.
channel.basicQos(10); // Set prefetch count to 10
Why Set a Suitable Prefetch Count?
Adjusting the prefetch count allows you to control the flow of messages. Setting it too low can limit throughput, while too high demands more resources from the consumer. Finding the right balance is essential for real-time performance.
3. Message Persistence and Delivery Guarantees
RabbitMQ offers multiple delivery modes for messages: transient and persistent. Persistent messages are written to disk, while transient messages are stored in RAM.
Although persistent messages guarantee that messages are not lost, depending on your use case, this can add latency. For real-time messaging, you might want to consider transient messages for minimal delay.
Code Snippet for Sending Persistent Messages
String message = "Hello, World!";
AMQP.BasicProperties props = new AMQP.BasicProperties.Builder()
.deliveryMode(2) // persistent
.build();
channel.basicPublish("", QUEUE_NAME, props, message.getBytes());
Why Choose the Right Message Type?
Evaluate the trade-off between reliability and performance. If loss of a few messages is acceptable in your system, using transient messages can significantly enhance performance due to reduced overhead.
4. Connection and Channel Management
Creating connections and channels can be resource-intensive operations. Efficient management is fundamental in a real-time messaging system.
Using Connection Pooling
Instead of creating channels on-the-fly, consider implementing connection pooling to reuse existing connections.
import org.apache.commons.pool2.ObjectPool;
import org.apache.commons.pool2.impl.GenericObjectPool;
public class RabbitMqConnectionPool {
private static ObjectPool<Channel> pool = new GenericObjectPool<>(new RabbitMqChannelFactory());
public static Channel getChannel() throws Exception {
return pool.borrowObject();
}
}
Why Use Connection Pooling?
Connection pooling reduces latency due to overhead in creating channels, thus facilitating better resource management and improved message processing rates.
5. Clustering and High Availability
Leveraging RabbitMQ clusters increases throughput and ensures high availability. Clusters can distribute the message load across several nodes, thus enhancing performance.
Configuring a RabbitMQ Cluster
- Install RabbitMQ on multiple nodes.
- Configure each node to be aware of the others.
- Establish mirrored queues to ensure messages are replicated across nodes.
Why Utilize Clustering?
A RabbitMQ cluster not only improves throughput but also provides failover capabilities, ensuring uninterrupted service in real-time applications.
For further reading on RabbitMQ clustering, you can refer to the official RabbitMQ clustering guide.
6. Monitoring and Metrics
Monitoring is crucial for real-time performance management. Use RabbitMQ's management plugin to visualize message flows, consumer statuses, and queue lengths.
Setting Up the Management Plugin
Enable the management plugin with the following command:
rabbitmq-plugins enable rabbitmq_management
After enabling, access the management interface at http://localhost:15672/
to gather insights.
Why Monitor Your RabbitMQ Instances?
Continuous monitoring helps identify bottlenecks, excessive latency, and other performance issues, allowing for timely intervention.
Final Thoughts
Optimizing RabbitMQ for real-time messaging involves a multifaceted approach that includes managing acknowledgments, configuring prefetch counts, making judicious choices about message persistence, ensuring efficient connection handling, and leveraging clustering for increased throughput. Additionally, reliable monitoring will provide insights that aid in maintaining performance and quick troubleshooting.
By implementing these optimizations, you can ensure your application responds swiftly to events, meets user expectations, and operates smoothly even under heavy loads. Real-time messaging is not just about speed but also about reliability, and a well-optimized RabbitMQ setup can help you achieve both.
For additional insights into improving messaging performance, explore more in-depth articles about RabbitMQ performance best practices and practical implementations.
Feel free to reach out with your thoughts or questions! Happy coding!