Common Mistakes New Apache Camel Users Should Avoid
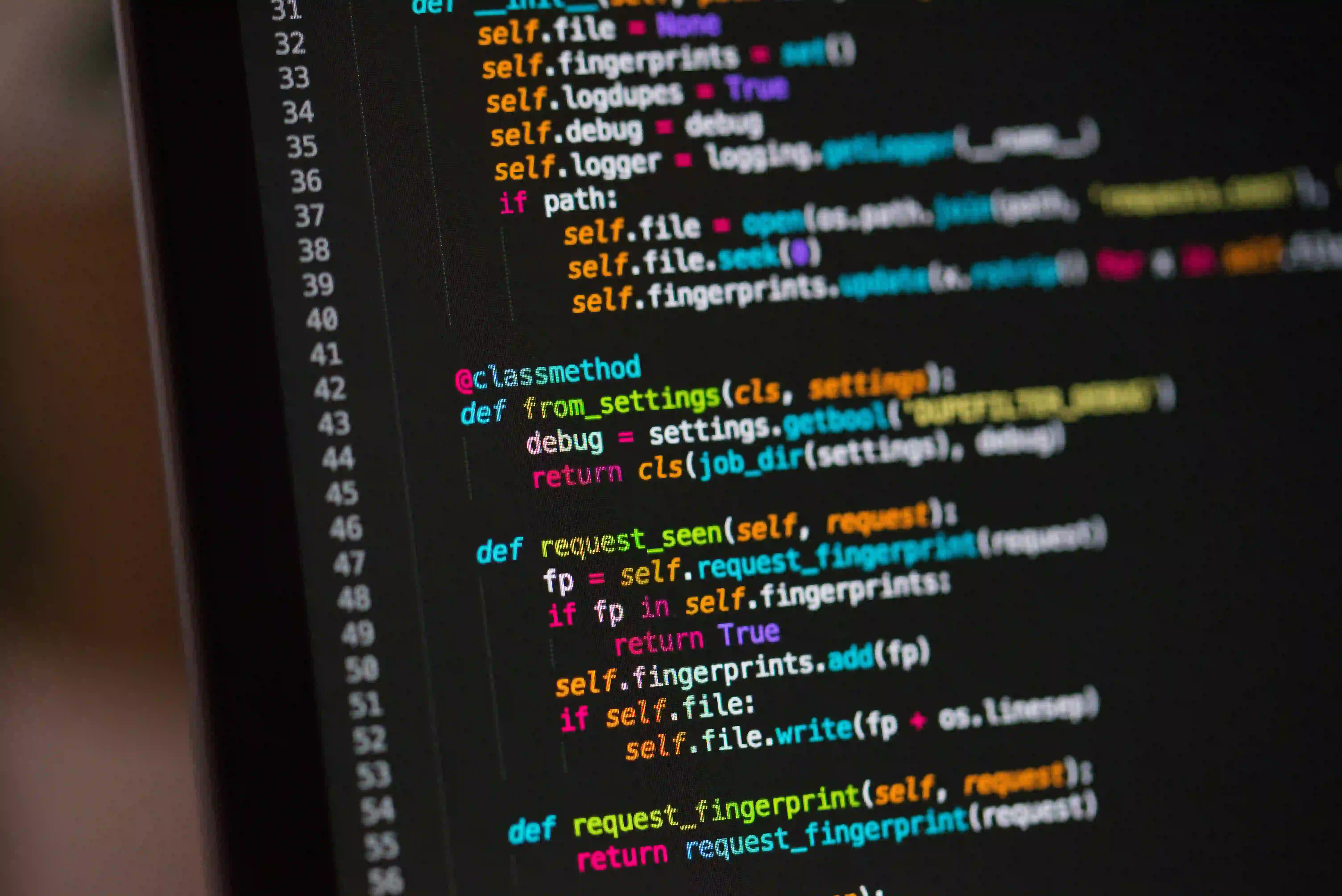
Common Mistakes New Apache Camel Users Should Avoid
Apache Camel is a powerful open-source integration framework that helps developers build robust data integration solutions easily. However, like any complex tool, it's easy for newcomers to fall into common pitfalls. In this blog post, we'll explore some of the frequent mistakes made by new Apache Camel users and how to avoid them.
1. Ignoring the Documentation
One of the most common mistakes is overlooking the rich documentation provided by the Apache Camel community. The official Apache Camel documentation offers crucial insights into the framework’s features, components, and setup.
Why It Matters
Documentation covers various patterns, routes, and best practices tailored for different use cases. Familiarizing yourself with this information can save hours of troubleshooting later.
Tip
Start with the official tutorials and read through the comprehensive user guide. This will help you grasp the fundamentals and avoid confusion as you build complex routes.
2. Overcomplicating Routes
New users often create routes that are overly complex, incorporating excessive components or using convoluted logic. While Apache Camel supports sophisticated routing patterns, simplicity should always be a priority.
Why It Matters
Clear and concise routes are more maintainable, easier to debug, and less prone to errors. Oversized integrations can lead to performance issues and make it difficult to follow the data flow.
from("direct:start")
.to("log:info")
.choice()
.when(header("type").isEqualTo("A"))
.to("mock:resultA")
.when(header("type").isEqualTo("B"))
.to("mock:resultB")
.otherwise()
.to("mock:resultDefault");
Commentary
In the code snippet above, we use a choice()
block for conditional routing. This structure allows for clear paths based on the message's header, ensuring that the routes remain simple and understandable.
3. Not Leveraging Components Properly
Apache Camel comes with a myriad of components for different endpoints (e.g., HTTP, JMS, JDBC). New users may either stick to a single component or fail to use the relevant ones effectively.
Why It Matters
Each component is optimized for specific tasks. By not leveraging them appropriately, you might miss out on performance enhancements and functionalities that could streamline your data flows.
Tip
Always be on the lookout for an appropriate component before choice-making during integration. Check out Camel's components documentation for available options.
4. Failing to Handle Exceptions
Proper exception handling is crucial in any integration solution. New users often neglect to implement error handling on their routes, leaving the applications vulnerable during failure scenarios.
Why It Matters
Without proper error handling, your routes may terminate unexpectedly, leading to data loss or service interruptions.
from("direct:start")
.doTry()
.to("http://external-service")
.doCatch(Exception.class)
.log("Error occurred: ${exception.message}")
.to("mock:errors");
Commentary
This example shows the doTry()
and doCatch()
blocks in action. By wrapping the main logic with these constructs, you can catch exceptions and log them effectively, providing a fallback route to handle errors gracefully.
5. Overlooking Testing
Testing is a crucial part of development. However, many new Apache Camel users do not incorporate unit and integration testing into their workflow.
Why It Matters
Testing ensures that your integration routes work as expected and helps to identify issues early in the development cycle.
Tip
Utilize frameworks like JUnit along with Camel’s testing support to create unit tests for your routes.
public class MyRouteTest extends CamelTestSupport {
@Test
public void testRoute() throws Exception {
// Set up a mock endpoint to verify interactions
getMockEndpoint("mock:result").expectedMessageCount(1);
// Send a message to the start endpoint
template.sendBody("direct:start", "Test Message");
// Verify that the message reached the mock endpoint
assertMockEndpointsSatisfied();
}
}
Commentary
In the testing example, we use Camel's built-in approach for unit testing routes. This method allows you to send test messages and verify if they reach the expected endpoints correctly.
6. Neglecting to Optimize Performance
Performance optimization is often disregarded, especially when developers are focused on getting the integration to work initially. It is crucial to keep performance in mind from the start.
Why It Matters
Suboptimal performance can lead to slow response times and may impact the overall user experience.
Tip
Monitor and analyze your routes using tools like JMX, and always be on the lookout for bottlenecks.
- Batch Processing: Group messages to reduce overhead.
- Load Balancing: Distribute workloads across multiple service instances.
7. Misconfiguring the Camel Context
The Camel Context is the core configuration that holds routes, components, and error handling. New users often misconfigure it, leading to issues in routing behavior or component integration.
Why It Matters
An incorrectly set up Camel Context can impede message processing and cause misrouting.
Tip
Always review your Camel Context configurations, especially if using Spring or Blueprint.
CamelContext context = new DefaultCamelContext();
context.addRoutes(new RouteBuilder() {
public void configure() {
from("timer:foo?repeatCount=1")
.to("log:info");
}
});
Commentary
In this simple example, we configure a Camel Context programmatically. Ensure that your context is set up correctly to initialize routes effectively before running any integration.
My Closing Thoughts on the Matter
While Apache Camel is designed to make integration simpler, new users can easily make critical mistakes that hinder their progress. By avoiding the common pitfalls discussed above, you’ll set yourself up for success with Camel.
Remember to take full advantage of the documentation, optimize your routes, implement error handling, and ensure thorough testing.
For further reading, check out Apache Camel's Best Practices and consider joining the Camel community for insights, tips, and support.
Taking a proactive approach towards learning and using Camel will not only enhance your capabilities as a developer but also lead to creating efficient and effective integration solutions. Happy coding!