Common InfluxDB Connection Issues in Spring Boot Apps
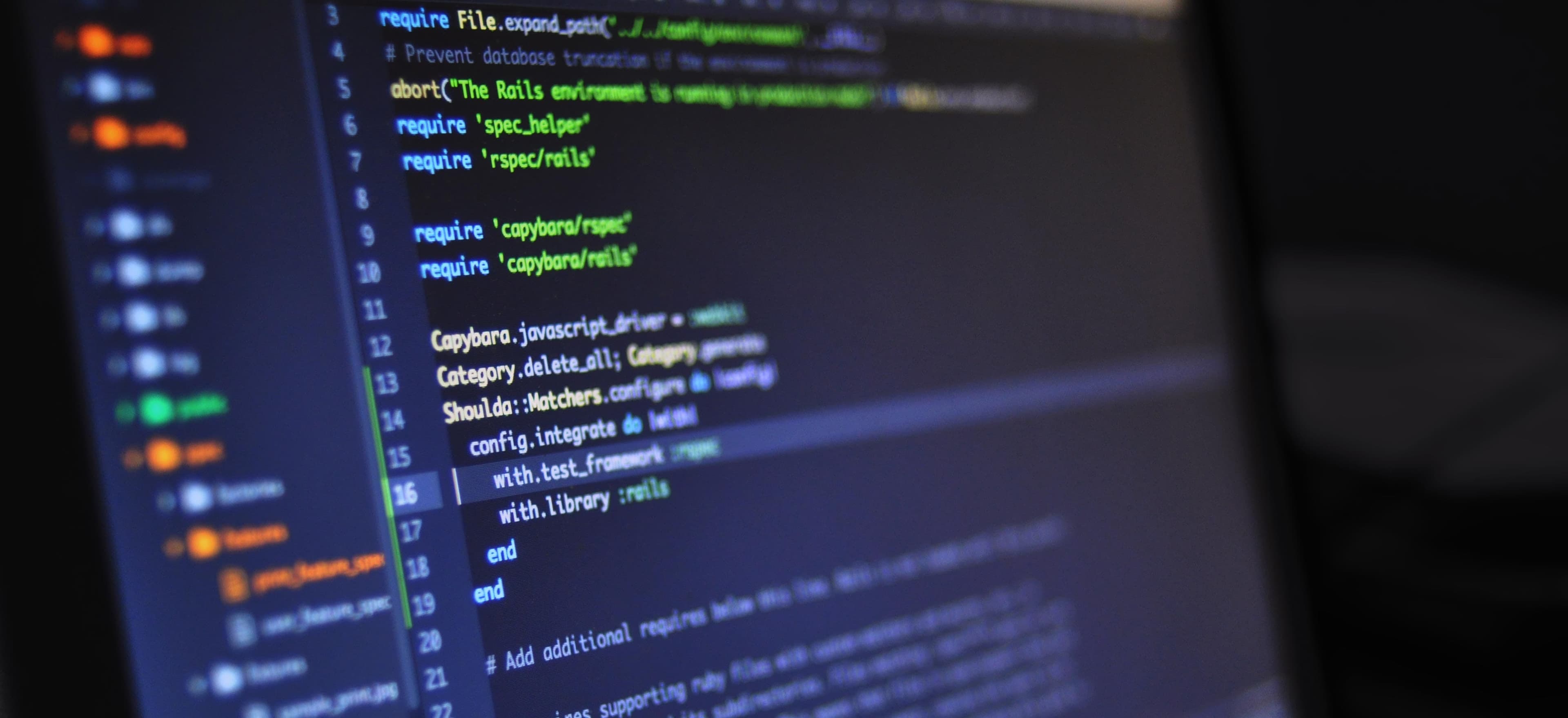
- Published on
Common InfluxDB Connection Issues in Spring Boot Apps
InfluxDB is a powerful time series database widely used for monitoring and analytics. Integrating InfluxDB with your Spring Boot applications can provide enhanced capabilities to manage and analyze time-stamped data efficiently. However, setting up the connection might not always be a straightforward process. This post covers the common InfluxDB connection issues faced by Spring Boot developers and how to troubleshoot them effectively.
Table of Contents
- Understanding InfluxDB
- Common Connection Issues
- A. Incorrect Configuration
- B. Network Issues
- C. Authentication Problems
- Best Practices for Connection Management
- Conclusion
- Additional Resources
Understanding InfluxDB
InfluxDB is designed to handle high write and query loads, making it an excellent choice for applications that require quick retrieval of time-based data, such as IoT applications, monitoring systems, and analytics. While Spring Boot makes it easy to create and configure microservices, integrating with InfluxDB requires a little more attention.
Setting Up InfluxDB with Spring Boot
Before diving into the common connection issues, let’s quickly set up InfluxDB in a Spring Boot application. You will need to add a dependency to your pom.xml
file for the InfluxDB client.
<dependency>
<groupId>org.influxdb</groupId>
<artifactId>influxdb-java</artifactId>
<version>2.22</version>
</dependency>
After that, you can configure the InfluxDB
bean in your Spring Boot application:
import org.influxdb.InfluxDB;
import org.influxdb.InfluxDBFactory;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class InfluxConfig {
@Bean
public InfluxDB influxDB() {
// Connection parameters for InfluxDB
String url = "http://localhost:8086"; // Update this according to your setup
String user = "username"; // Your InfluxDB username
String password = "password"; // Your InfluxDB password
// Establishing Connection
InfluxDB influxDB = InfluxDBFactory.connect(url, user, password);
return influxDB;
}
}
The above configuration creates a reusable InfluxDB
bean, making it easy to inject where needed. Now, let’s explore some common connection issues you might encounter during integration.
Common Connection Issues
Despite our best efforts, several issues may arise that prevent the application from connecting to InfluxDB. Below are some of the most common ones.
A. Incorrect Configuration
One of the most prevalent issues is an incorrectly configured connection parameter.
Debugging Tips:
- Check URL and Port: Make sure the URL and port you are using are correct. The default port for InfluxDB is
8086
. - Validate Credentials: Ensure the username and password are set correctly and that the specified user has access to the database.
- Database Existence: Verify that the database you are trying to write to or query exists.
B. Network Issues
Network problems can often lead to connection failures. If your InfluxDB instance is not running on the localhost, ensure the network route is accessible.
Common Checks:
- Ping the Server: Use ping commands to check connectivity.
- Firewalls: Ensure that firewalls are not blocking the port. Update firewall rules if necessary.
- Docker Configuration: If you are running InfluxDB in a Docker container, ensure that the network is configured to allow communication between the Spring Boot app and the InfluxDB instance.
C. Authentication Problems
Authentication issues can also be tricky, especially if you are using InfluxDB 2.x, where RBAC (Role-Based Access Control) is mandated.
Suggestions for Resolution:
- Use Token-Based Authentication: Starting from version 2.0, InfluxDB uses token-based authentication. Make sure you are passing the correct token instead of the username and password.
- Verify User Permissions: Check whether the user has adequate permissions to access the relevant database or perform the operations you are initiating.
Best Practices for Connection Management
To avoid common connection issues and improve the performance of your Spring Boot application, consider implementing the following best practices:
-
Use Connection Pooling: Leverage a connection pool to manage multiple connections efficiently. This minimizes the overhead of creating new connections for every request and helps with resource management.
import org.influxdb.InfluxDB; import org.influxdb.InfluxDBFactory; import org.influxdb.impl.InfluxDBImpl; import org.influxdb.exceptions.InfluxDBIOException; public class InfluxDBPooling { private InfluxDB influxDB; public InfluxDBPooling(String url, String user, String password) { InfluxDBFactory.connect(url, user, password); } public InfluxDB getConnection() { return influxDB; // Consider implementing a connection pool here } }
-
Monitor Connection Health: Regularly check the health of your connection and implement appropriate fallback mechanisms.
-
Handle Exceptions Gracefully: Implement robust error handling around connection attempts to enable your application to remain stable under unfavorable conditions.
-
Read the InfluxDB Documentation: For any advanced features and connection details, always refer to the official InfluxDB Documentation to stay updated with the best practices.
-
Use Logging: Implement logging to capture connection attempts and failures, aiding in troubleshooting when things go awry.
Wrapping Up
Integrating InfluxDB into your Spring Boot applications can significantly boost your application's capabilities to handle time-series data. However, it is essential to be mindful of potential connection issues and adopt best practices for smooth operation. A solid understanding of your setup's requirements and thorough configuration can save you time in the long run.
For more insight into time-series databases and their applications, check out this comprehensive InfluxDB guide.
By following the steps and tips provided in this post, you can configure, troubleshoot, and optimize your InfluxDB connection in a Spring Boot application effectively. Happy coding!
Checkout our other articles