Common Pitfalls in AI Microservice Design and How to Fix Them
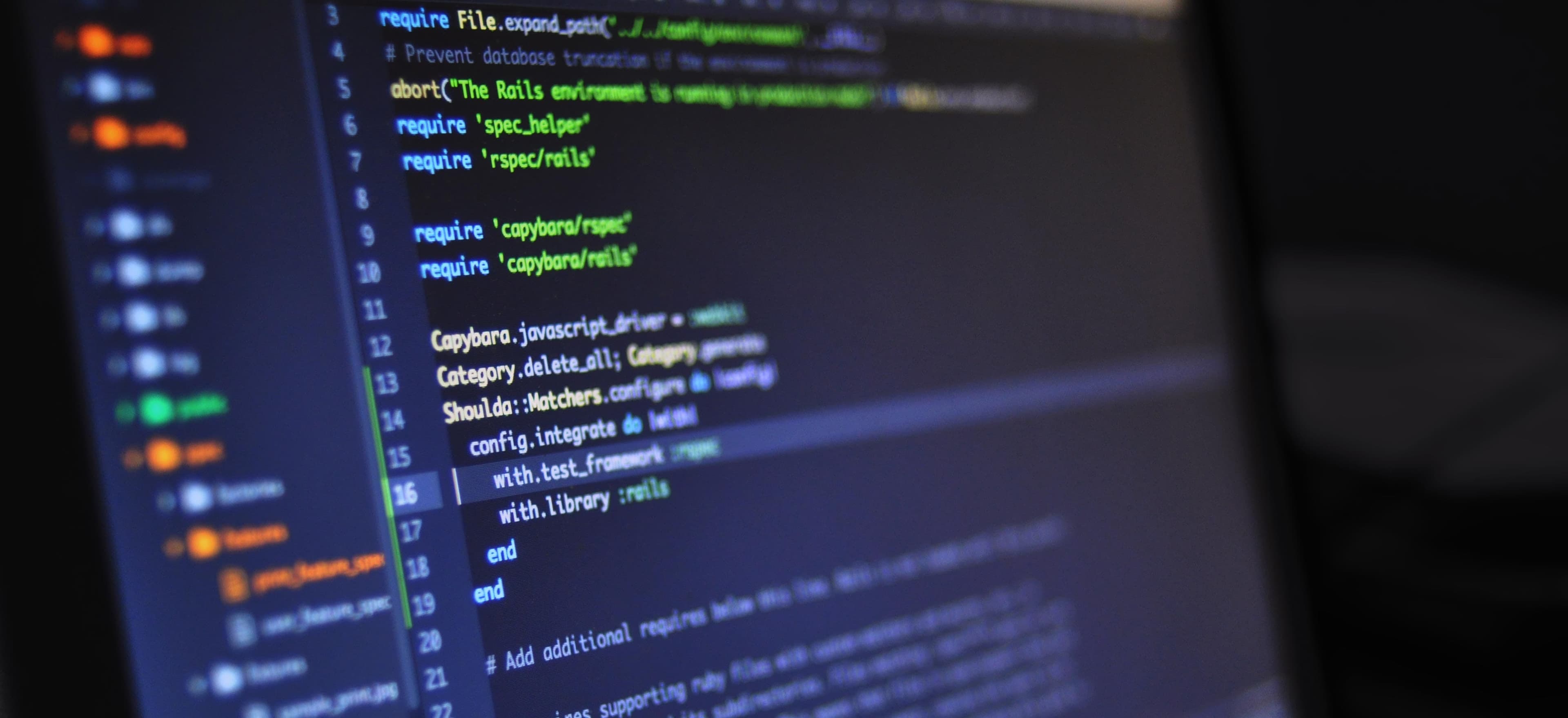
- Published on
Common Pitfalls in AI Microservice Design and How to Fix Them
The adoption of microservices architecture has transformed software design, enabling greater scalability, flexibility, and maintainability. This is particularly crucial in the realm of Artificial Intelligence (AI), where evolving models and deteriorating performance can become significant challenges. This blog post will outline common pitfalls in AI microservice design, along with practical solutions to mitigate these issues.
Understanding AI Microservices
Before diving into pitfalls, it's essential to grasp the concept of AI microservices. These are independent, modular services that encapsulate specific AI functionalities (like model predictions, data processing, or model training) within an application. These services communicate over a network, allowing teams to develop, deploy, and scale them independently.
1. Ignoring Data Management
The Issue
Data is the lifeblood of any AI system. Unfortunately, many AI microservice architectures overlook effective data management strategies. This oversight can lead to issues such as data drift, inconsistencies, and subsequently degraded models.
The Solution
Implement robust data governance and management practices. One way to do this is through data versioning and metadata management. Store different versions of datasets, enabling your microservices to access the relevant training data as models evolve.
Example Code Snippet
public class DataVersioning {
private Map<String, String> dataVersions;
public DataVersioning() {
dataVersions = new HashMap<>();
}
public void uploadData(String versionTag, String dataLocation) {
dataVersions.put(versionTag, dataLocation);
}
public String getDataLocation(String versionTag) {
return dataVersions.get(versionTag);
}
}
Why: This simple DataVersioning
class allows you to maintain different versions of datasets. It provides a straightforward way to reference data versions, which is crucial for training and retraining models.
2. Neglecting Model Deployment and Monitoring
The Issue
Deploying an AI model is only the beginning. Failing to monitor model performance in real-time can lead to catastrophic failures as models age or encounter new data distributions.
The Solution
Adopt continual monitoring practices for your AI models. Consider employing tools like Prometheus for gathering metrics and Grafana for visualization. Integrate monitoring directly into your microservices.
Example Code Snippet
public class ModelMonitor {
private static final double THRESHOLD = 0.8;
public boolean isModelPerforming(double accuracy) {
return accuracy >= THRESHOLD;
}
}
Why: The ModelMonitor
class provides a simple mechanism for assessing model performance based on a predefined accuracy threshold. Integrating performance checks ensures your models are consistently evaluated against real-world data.
3. Lack of API Versioning
The Issue
Microservices often evolve faster than expected; however, introducing breaking changes can disrupt existing consumers. Not having a proper API versioning strategy can lead to major integration issues.
The Solution
Implement versioning in your API endpoints. This ensures backward compatibility while allowing new features or improvements to a microservice without disrupting the older clients.
Example Code Snippet
@RestController
@RequestMapping("/api/v1/models")
public class ModelController {
@GetMapping("/{id}")
public ResponseEntity<Model> getModel(@PathVariable String id) {
// Logic to retrieve model
}
}
Why: By adding versioning to the API path, you allow clients to specify which version of the API they wish to access. This reduces friction during updates and provides a clear separation of concerns.
4. Overcomplicating the Microservices
The Issue
Designing microservices can unintentionally lead to over-engineering. Too many functionalities packed into a single microservice can defeat the purpose of microservices, leading to increased complexity instead of simplicity.
The Solution
Adopt a minimalist approach. When creating a new microservice, apply the Single Responsibility Principle: each service should focus on a single business capability or function.
Example Code Snippet
public class PredictionService {
public Prediction predict(InputData inputData) {
// Logic for making predictions
}
}
Why: The above definition of PredictionService
encapsulates just one responsibility—predicting outcomes based on input data. This maintains clear boundaries between services and minimizes complexity.
5. Inadequate Error Handling and Recovery
The Issue
Microservices, especially in AI, can fail for various reasons—from model predictions leading to unexpected results to service outages. Inadequate error handling can lead to cascading failures across your application.
The Solution
Design your microservices to handle errors gracefully. Use retry mechanisms, fallbacks, and circuit breakers to ensure your system remains robust under failure conditions.
Example Code Snippet
public class PredictionServiceWithRetry {
public Prediction safePredict(InputData inputData) {
for (int attempt = 1; attempt <= 3; attempt++) {
try {
return predict(inputData);
} catch (Exception e) {
if (attempt == 3) {
throw e; // Rethrow after 3 failed attempts
}
}
}
return null; // Fallback
}
}
Why: In this example, the safePredict
method attempts to make a prediction up to three times before giving up. This simple strategy can mitigate temporary issues, keeping the microservice operational during errors.
6. Failure to Establish Clear Interfaces
The Issue
Clear interfaces are essential for microservice communication. Poorly designed APIs can lead to confusion, integration issues, and ultimately a fragile system.
The Solution
Invest time in designing and documenting your APIs. Ensure they are intuitive, consistently named, and that they adhere to standard practices.
Example Code Snippet
public interface ModelService {
Model getModelById(String id);
List<Model> getAllModels();
}
Why: Defining clear interfaces like ModelService
allows other services easy access to model retrieval functionalities. It encapsulates behavior and exposes a limited surface area for interaction.
Wrapping Up
Designing AI microservices poses unique challenges, from managing data effectively to ensuring robust performance monitoring. By recognizing these common pitfalls and implementing best practices, you can overcome them and create a more resilient architecture.
For further reading on microservice design patterns, check out Microservices Patterns by Chris Richardson and Building Microservices for comprehensive insights on scaling your architecture effectively.
By staying mindful of these challenges and focusing on clear, maintainable design principles, your journey into AI microservice architecture can be a rewarding one. Happy coding!