Mastering Java 7 Project Coin: Common Pitfalls Explained
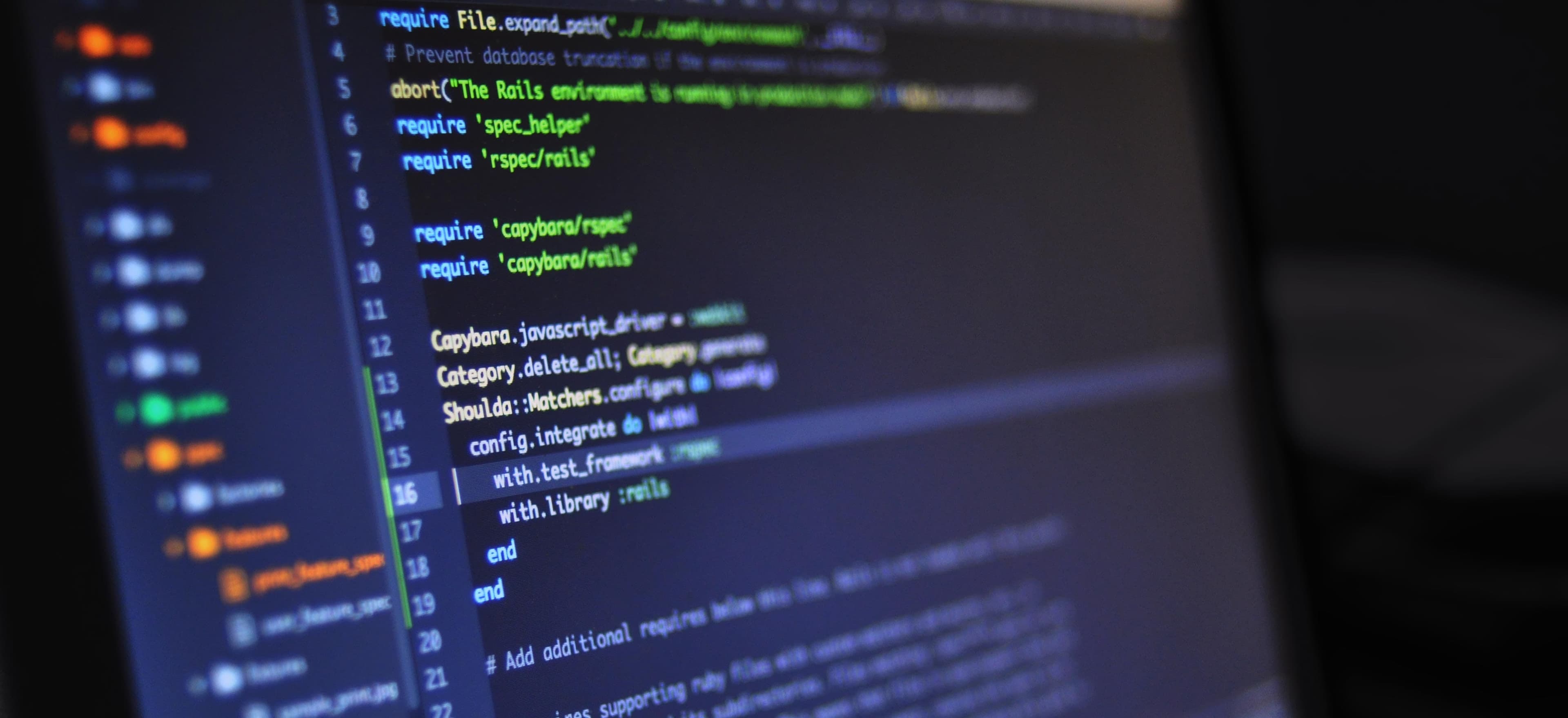
- Published on
Mastering Java 7 Project Coin: Common Pitfalls Explained
The introduction of Java 7 marked a significant enhancement to the Java programming language, thanks to its Project Coin initiative. This project was aimed at promoting smaller language and library changes that improve coding experience while maintaining backward compatibility. Java 7 introduced several features, but developers often fall into pitfalls while implementing them. In this blog post, we will explore some of the most common pitfalls associated with Java 7 Project Coin and provide clear explanations, mitigating strategies, and code snippets to reinforce understanding.
Understanding Project Coin
Project Coin introduced fourteen new language features, the most notable of which include:
- Diamond Operator: Simplifying generic type instantiation.
- String in Switch: Enabling the use of Strings in switch statements.
- Try-With-Resources: Simplifying resource management in try statements.
- Automatic Exception Handling: A cleaner way to handle exceptions.
- Numeric Literals with Underscores: Improving readability of numbers.
Understanding these new features is crucial, but so is recognizing potential pitfalls when using them.
Common Pitfalls
1. Misusing the Diamond Operator
One of the most anticipated features was the diamond operator (<>
). It alleviates the verbosity of declaring types. However, misusing it can lead to compilation errors or unintended behavior.
Code Example:
List<String> list = new ArrayList<>(); // Correct usage
list.add("Java");
Pitfall: Although the diamond operator simplifies code, it can lead to issues if the context is not immediately clear.
Solution:
Always ensure that your generic types are clear in context, especially when creating instances as parameters.
Map<String, List<String>> map = new HashMap<>();
In this case, the type parameters help readers understand that the map will hold Lists identified by Strings.
2. Strings in the Switch Statement
Using Strings in switch statements was a long-awaited feature. However, this can lead to runtime exceptions if not handled properly.
Code Example:
String response = "yes";
switch (response) {
case "yes":
System.out.println("Response is yes");
break;
case "no":
System.out.println("Response is no");
break;
default:
System.out.println("Unknown response");
}
Pitfall: Failing to provide a default case can lead to verbose debugging if unexpected values are encountered.
Solution:
Always include a default case, even if you believe inputs will be controlled.
default:
System.out.println("Unknown response: " + response);
Including the default
ensures that your program handles all possible values gracefully.
3. Try-With-Resources Misuse
Try-with-resources simplifies resource management by automatically closing resources. However, developers often misunderstand its limitations.
Code Example:
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
Pitfall: Developers sometimes think that try-with-resources can manage all types of resources. This is not the case.
Solution:
Only use try-with-resources for classes that implement AutoCloseable
. Ensure you are aware of resource types before applying this feature.
OutputStream os = new FileOutputStream("file.txt");
try (BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(os))) {
// Write Logic
}
This way, you take full advantage of try-with-resources with an understanding of its constraints.
4. Handling Exceptions Improperly
Java 7 emphasizes cleaner exception handling, but many developers end up catching too broad exceptions or miss handling checked exceptions.
Code Example:
try {
// risky code
} catch (Exception e) {
e.printStackTrace(); // Too Broad!
}
Pitfall: Broad exception handling doesn’t provide clarity about error handling and can lead to hiding critical problems.
Solution:
Always catch specific exceptions first, and only use a catch-all (Exception
) if necessary.
try {
// risky code
} catch (IOException e) {
e.printStackTrace(); // Handle IO specifically
} catch (SQLException e) {
e.printStackTrace(); // Handle SQL specifically
} catch (Exception e) {
e.printStackTrace(); // General catch must be last
}
This approach gives you precise control over error management and makes debugging easier.
5. Using Numeric Literals with Underscores
Numeric Literals with Underscores was introduced to improve readability. However, incorrect usage can lead to confusion.
Code Example:
int binary = 0b1010_1011; // Valid Usage
int invalidBinary = 0b10_2; // Invalid Usage
Pitfall: Misplacing underscores can lead to compiler errors without clear messages.
Solution:
Follow the rules for underscore placement:
- Cannot start or end with an underscore.
- Cannot have consecutive underscores.
long creditCardNumber = 1234_5678_9012_3456L; // Correct usage
Utilizing this feature properly can significantly enhance the readability of large numeric values.
To Wrap Things Up
Java 7's Project Coin brought significant improvements, making code more concise and readable. However, as encountered in this post, certain pitfalls can arise if developers do not fully understand these new features or misuse them. By being aware of these common mistakes and knowing how to avoid them, you can take full advantage of Java 7's enhancements while maintaining code quality and stability.
For further reading on Java 7 and its new features, check out the official Oracle documentation. Embrace these enhancements and write cleaner, more efficient code!
In summary, keeping these lessons in mind will help you navigate Java 7's features smoothly. Remember to code mindfully and enjoy the robust functionalities that are now at your disposal!