Mastering Default and Defender Methods in Java 8
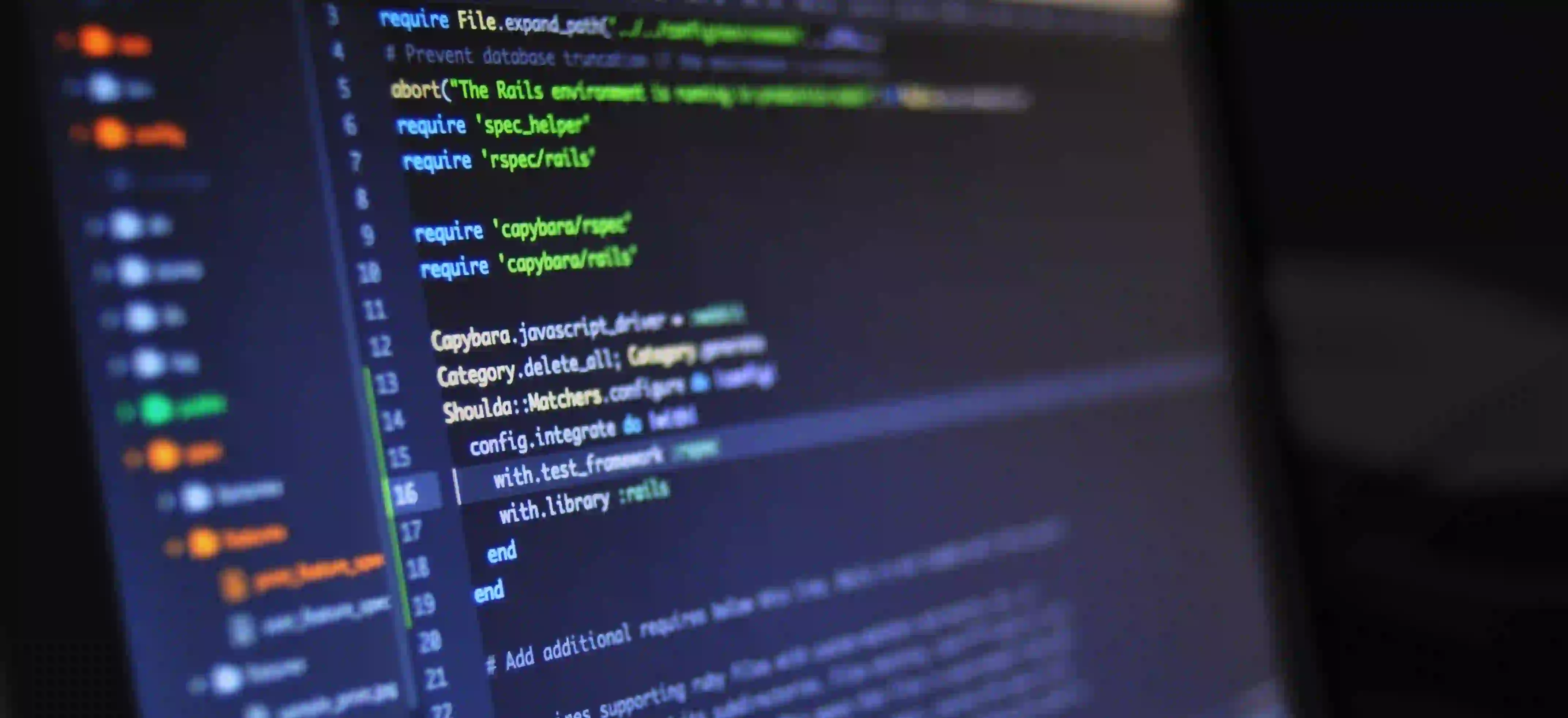
Mastering Default and Defender Methods in Java 8
Java 8 brought a number of significant enhancements to the language, one of the most notable being the introduction of default and static methods in interfaces. These new features transformed the way developers approach interfaces, allowing them to evolve without breaking existing implementations. In this blog post, we will delve deep into default and static methods, exploring their purpose, functionality, and practical applications in modern Java programming.
Understanding Default Methods
Before Java 8, interfaces could only declare methods, which meant that any implementing class had to provide actual definitions for all methods. This limitation often led to cumbersome class hierarchies and made it difficult to evolve APIs without breaking existing code. Default methods address this issue by allowing developers to add new methods to interfaces with a default implementation.
Syntax of Default Methods
The syntax for defining a default method is straightforward. Here’s a simple example:
public interface Vehicle {
void start();
default void stop() {
System.out.println("Stopping the vehicle.");
}
}
In the code above, the Vehicle
interface declares a method start
that must be implemented, while the stop
method comes with a default implementation. Any class implementing Vehicle
must provide an implementation for start
, but stop
can be inherited directly.
Why Use Default Methods?
-
Backward Compatibility: Default methods allow existing interfaces to add new functionality without breaking older implementations. This is essential for maintaining libraries and APIs.
-
Code Reusability: You can provide common functionalities in the default method, reducing duplicate code across implementing classes.
-
Enhanced Readability: By adding concrete methods directly in interfaces, the code can become more readable and intuitive.
Implementing Default Methods
Let’s see how default methods can be implemented in a real-world scenario.
public class Car implements Vehicle {
@Override
public void start() {
System.out.println("Starting the car.");
}
// Optional: Override default method
@Override
public void stop() {
System.out.println("Stopping the car.");
}
}
public class Bike implements Vehicle {
@Override
public void start() {
System.out.println("Starting the bike.");
}
// Utilizes default stop implementation without overriding
}
In this example, we have two classes: Car
and Bike
, both implementing the Vehicle
interface. The Car
class overrides the default stop
method, while Bike
uses the default method directly.
Key Takeaways
- Flexibility: Classes can choose to override default methods or rely on the provided implementations.
- Multiple Inheritance: Java's interfaces can utilize multiple default methods from different interfaces. However, if a class implements two interfaces with the same default method, it must provide an implementation to resolve the conflict.
Static Methods in Interfaces
Alongside default methods, Java 8 introduced static methods in interfaces. A static method in an interface serves as a utility function and is not tied to any particular instance.
Syntax of Static Methods
Defining a static method in an interface is similar to how you would do it in a class:
public interface MathUtils {
static int add(int a, int b) {
return a + b;
}
static int subtract(int a, int b) {
return a - b;
}
}
Here, the MathUtils
interface contains two static methods for addition and subtraction. You can invoke these methods directly without creating an instance of any implementing class.
Why Use Static Methods?
-
Utility Functions: Static methods can be used for utility functions related to the interface but do not require any instance. This aids in better organization of code.
-
Encapsulation of Constants: Static methods can also be used to encapsulate constant values or operations related to those constants.
-
Code Organization: Allowing static methods in interfaces helps keep related functionality grouped together logically.
Utilizing Static Methods
Here’s how to use the static methods we defined earlier:
public class Main {
public static void main(String[] args) {
int sum = MathUtils.add(5, 3);
int difference = MathUtils.subtract(10, 6);
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
}
}
In this code snippet, we use the static methods from the MathUtils
interface directly. There’s no need to create an object of an implementation class, leading to concise and readable code.
Potential Use Cases of Default and Static Methods
-
Functional Interfaces: Default methods are particularly useful in functional interfaces. They can add additional behaviors while keeping the interface focused on its primary function.
-
Framework Development: When developing frameworks, default methods can help provide default behavior to classes while allowing users the flexibility to override it when necessary.
-
APIs: API designers can safely introduce new methods in existing interfaces, providing enhanced functionality without breaking existing circuit.
Bringing It All Together
The introduction of default and static methods in Java 8 has significantly changed how developers approach interfaces. These methods allow for greater flexibility, backward compatibility, and clean code organization. By mastering these features, developers can create more robust, maintainable, and scalable applications.
For further reading on the new features introduced in Java 8, check out Oracle's Java Documentation and Java 8 in Action by Raoul-Gabriel Urma.
Remember, with every new feature in Java, it is crucial to understand when and how to apply them effectively in your programming practices. Happy coding!