Mastering Spring Cloud: Common Setup Pitfalls to Avoid
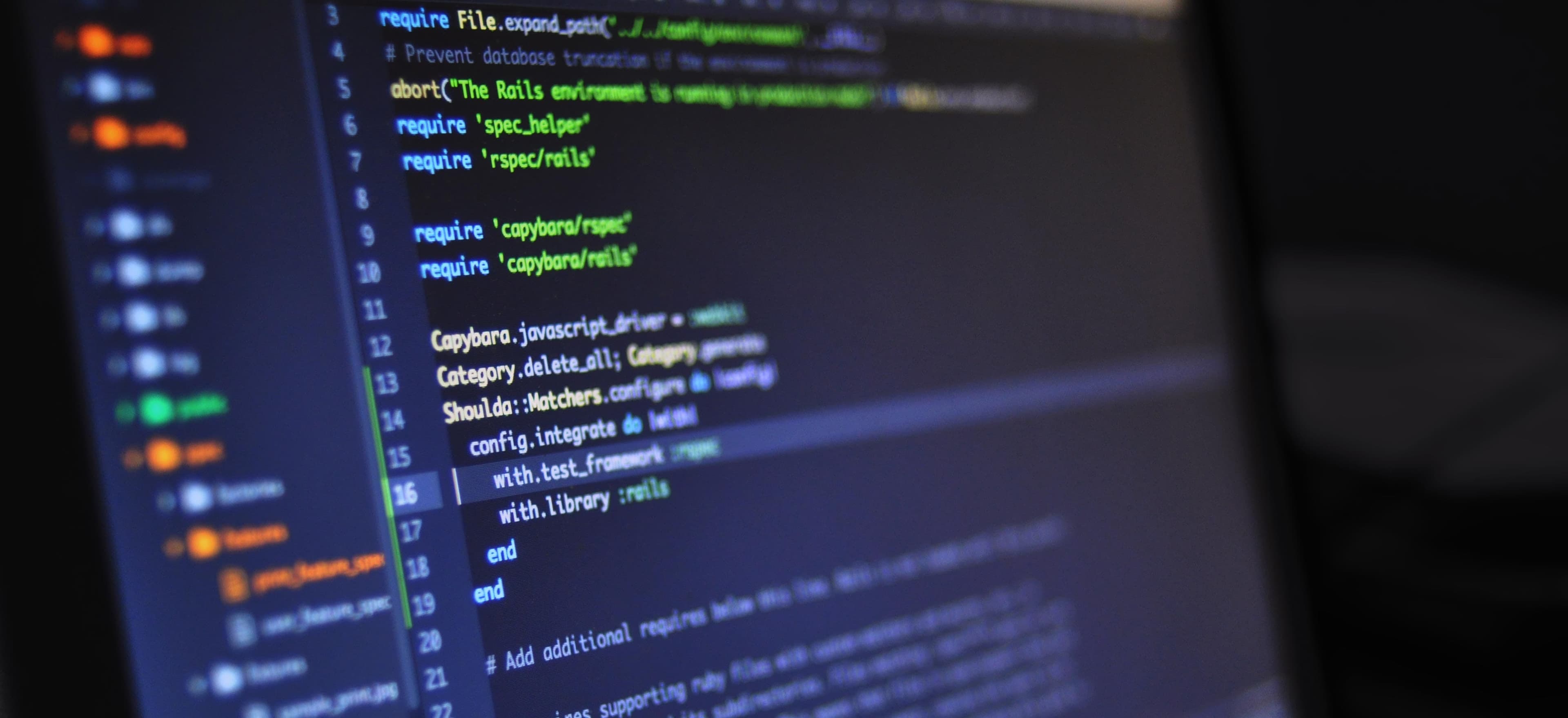
- Published on
Mastering Spring Cloud: Common Setup Pitfalls to Avoid
Spring Cloud provides a suite of tools that enables developers to build robust microservices architectures. However, improper setup and configuration can lead to frustrating challenges, particularly for those new to the ecosystem. In this blog post, we will explore common pitfalls in setting up Spring Cloud and how to avoid them.
Understanding Spring Cloud
Before diving into the setup pitfalls, let's briefly discuss what Spring Cloud is and why it's important. Spring Cloud is an extension of the Spring framework, specifically designed to make it easier to develop distributed systems. It offers tools for service discovery, configuration management, circuit breakers, and more.
For more information on Spring Cloud, visit the official Spring Cloud documentation.
Common Setup Pitfalls
1. Not Understanding the Role of Service Discovery
Pitfall: Misconfiguring service discovery is one of the most common errors made during Spring Cloud setup. Many developers overlook the importance of service discovery tools like Netflix Eureka or Consul, treating them merely as optional extras.
Solution: Understand that service discovery is crucial for microservices. It facilitates communication between services without hard-coding their locations.
Here’s a simple configuration for a Eureka server in your Spring Boot application:
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Why: The @EnableEurekaServer
annotation sets up an Eureka server that allows other microservices to register themselves and discover each other. Proper setup ensures that your microservices can communicate seamlessly, reducing the risk of failures.
2. Hardcoding Configuration Values
Pitfall: Hardcoding configuration values leads to unmanageable code and makes your application less flexible and harder to maintain.
Solution: Leverage Spring Cloud Config to externalize your configuration.
# application.yml
spring:
application:
name: my-service
cloud:
config:
uri: http://localhost:8888
Why: By using Spring Cloud Config, you separate your configuration from the codebase. This approach makes it easier to manage different environments (development, testing, production) while maintaining a single codebase.
3. Ignoring API Gateway Patterns
Pitfall: Without an API gateway, your microservices can become cluttered with routing logic, making it hard to manage requests efficiently.
Solution: Use Spring Cloud Gateway to facilitate routing.
@Configuration
public class GatewayConfig {
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("service1", r -> r.path("/service1/**")
.uri("lb://SERVICE1"))
.build();
}
}
Why: The RouteLocator
configuration allows you to define routes that point to specific microservices effectively. It centralizes request routing logic, enabling a cleaner architecture while improving security and performance.
4. Skipping Tests on the Configuration Server
Pitfall: Many developers implement Spring Cloud Config but neglect testing their configurations. This oversight can lead to runtime errors that are difficult to trace.
Solution: Establish a testing strategy for your configuration server.
@RunWith(SpringRunner.class)
@SpringBootTest(webEnvironment = SpringBootTest WebEnvironment.RANDOM_PORT)
public class ConfigServerTest {
@MockBean
private Environment environment;
@Test
public void testConfigRetrieval() {
// Simulate environment properties retrieval
when(environment.getProperty("my.property")).thenReturn("myValue");
// Add assertions as required
}
}
Why: Unit and integration tests ensure that your configuration server behaves as expected. Incorporating tests into your development process helps identify misconfigurations early, saving time and effort.
5. Brushing Aside Security Components
Pitfall: Failing to secure your microservices can expose them to vulnerabilities. Developers often assume Spring Cloud's security is "enabled by default."
Solution: Properly configure security features to protect your services.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.oauth2Login();
}
}
Why: The configuration above secures your services by requiring authentication for endpoints. Security should be a priority, especially when dealing with sensitive data.
6. Forgetting About Circuit Breakers
Pitfall: Underestimating the importance of resilience in microservices can lead to cascading failures if one service goes down.
Solution: Utilize circuit breakers, such as those provided by Netflix Hystrix.
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String callService() {
// Call another microservice
}
public String fallbackMethod() {
return "Service temporarily unavailable";
}
Why: Circuit breakers help prevent network or service failures from affecting the entire system. By implementing fallback methods, you can manage failure gracefully and provide seamless user experiences.
7. Missing Documentation and Comments
Pitfall: Poorly documented code can become the bane of any team's development efforts. Everyone assumes they understand the setup until it's time to change or troubleshoot it.
Solution: Ensure your code is thoroughly documented, with comments explaining the setup and logic.
// This method sets up a custom route for the API gateway
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes() // Start the route configuration
.route("service1", r -> r.path("/service1/**")
.uri("lb://SERVICE1")) // Load balancer URI
.build(); // Construct the route locator
}
Why: Good documentation not only aids in team collaboration but also simplifies future maintenance. A well-documented project is more likely to survive as team members change over time.
Closing the Chapter
While setting up Spring Cloud may seem straightforward at first glance, there are numerous pitfalls that can arise. By understanding and avoiding these common pitfalls, you can set up a robust microservices architecture that is flexible, secure, and easy to manage.
For further reading, you might find these resources helpful:
By mastering the key components of Spring Cloud and being aware of potential setup challenges, you're one step closer to building efficient and resilient microservices applications. Happy coding!