Overcoming Memory Issues When Parsing Large XML Files in Java
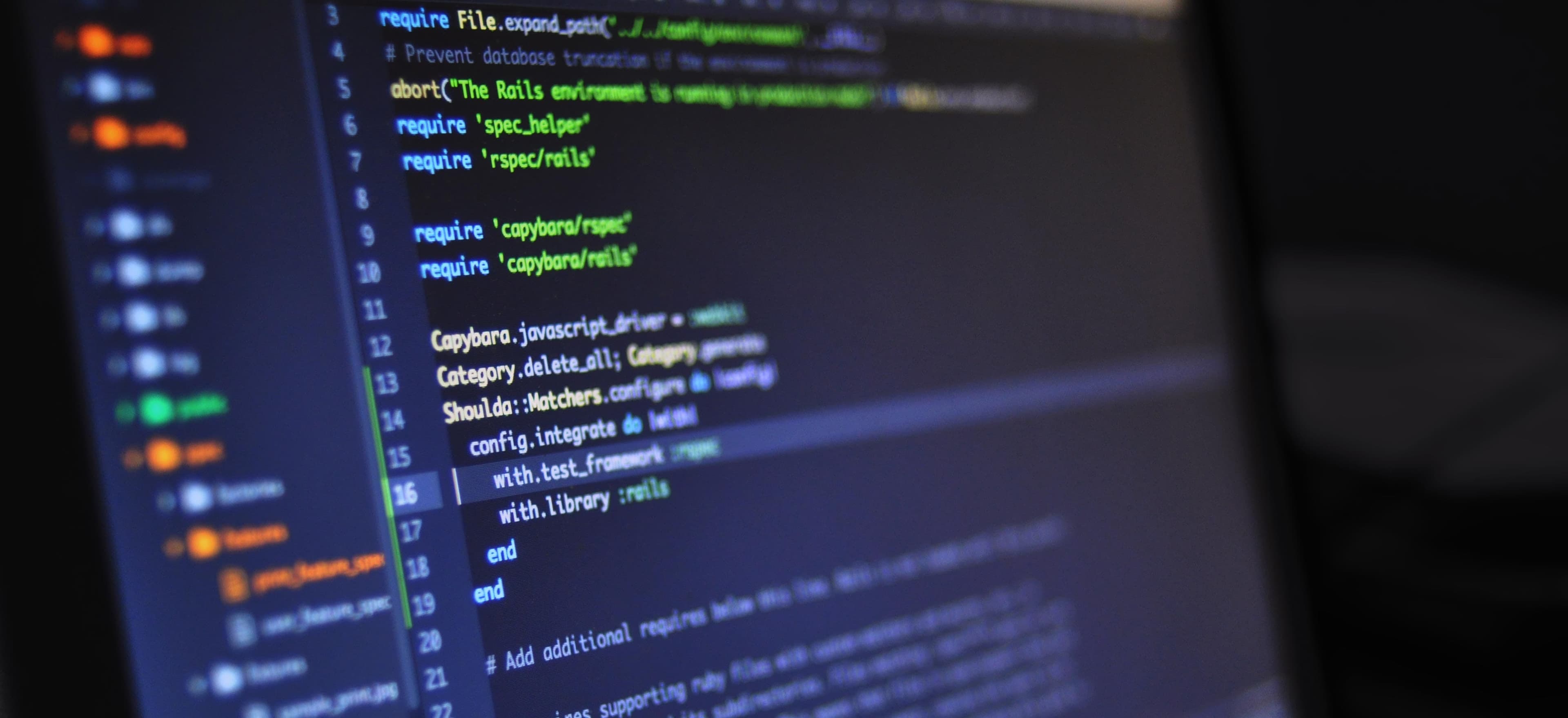
- Published on
Overcoming Memory Issues When Parsing Large XML Files in Java
Parsing large XML files in Java can be a challenging task, especially when memory issues arise. XML is a widely used format for storing and transporting data, but its hierarchical structure can lead to significant memory consumption during parsing. In this post, we will explore various strategies to overcome memory issues when working with large XML files in Java. We'll discuss event-driven parsing, streaming APIs, and provide practical code snippets to illustrate these approaches.
Understanding XML Parsing in Java
Java provides several methods for parsing XML. The two primary approaches are:
-
DOM (Document Object Model): The DOM parser reads the entire XML file into memory and builds a tree structure. While this allows for easy manipulation of the XML data, it is highly memory-intensive and not suitable for large files.
-
SAX (Simple API for XML): The SAX parser reads the XML file sequentially and triggers events based on XML elements. This approach is more memory-efficient as it does not load the entire document into memory.
For large XML files, using the SAX method or the StAX (Streaming API for XML) method is recommended to mitigate memory consumption.
Why Not Use DOM for Large XML Files?
Using the DOM parser for large XML files can quickly lead to OutOfMemoryError
, as it attempts to load the full XML structure into JVM memory. For instance, parsing a 100 MB XML file could potentially consume hundreds of megabytes of heap space, making it impractical for large data sets where the structure may contain millions of nodes.
Here's a simple example of Dom parsing:
import org.w3c.dom.*;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
public class DomParserExample {
public static void main(String[] args) {
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse("largefile.xml");
// Processing the document...
NodeList nodeList = document.getElementsByTagName("item");
for (int i = 0; i < nodeList.getLength(); i++) {
Element element = (Element) nodeList.item(i);
System.out.println("Item: " + element.getTextContent());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
This code illustrates the potential inefficiencies of parsing a large XML file, as it holds all data in memory.
Utilizing SAX for Efficient XML Parsing
SAX provides a more efficient approach. It allows for a lower memory footprint by processing XML data incrementally rather than in one go. Below is an example of how to implement a SAX parser.
SAX Parser Example
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
public class SaxParserExample {
public static void main(String[] args) {
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
DefaultHandler handler = new DefaultHandler() {
boolean isItem = false;
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
if (qName.equalsIgnoreCase("item")) {
isItem = true;
}
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException {
if (isItem) {
System.out.println("Item: " + new String(ch, start, length));
isItem = false;
}
}
};
saxParser.parse("largefile.xml", handler);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on SAX Example
In the SAX parser example above, the DefaultHandler
class allows us to handle events related to the XML parsing. The startElement
method captures opening tags, and characters
handles the text between tags. This method consumes minimal memory since it processes data in chunks, making it suitable for large files.
Introducing StAX for Streaming XML Processing
The StAX (Streaming API for XML) parser is another excellent option for handling large XML files. It provides a pull-parsing approach, allowing developers to control the parsing process. This means that you can read through the file as needed, which further helps in managing memory.
StAX Parser Example
import javax.xml.stream.XMLEventReader;
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.events.XMLEvent;
import java.io.FileInputStream;
public class StaxParserExample {
public static void main(String[] args) {
try {
XMLInputFactory factory = XMLInputFactory.newInstance();
FileInputStream fis = new FileInputStream("largefile.xml");
XMLEventReader eventReader = factory.createXMLEventReader(fis);
while (eventReader.hasNext()) {
XMLEvent event = eventReader.nextEvent();
if (event.isStartElement() && event.asStartElement().getName().getLocalPart().equals("item")) {
event = eventReader.nextEvent();
System.out.println("Item: " + event.asCharacters().getData());
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on StAX Example
The StAX parser reads events from the XML file as a stream. It allows you to pull data as needed without loading the entire document, which is particularly useful for large files. In the above example, we utilize XMLEventReader
to process elements, reading only what we need and optimizing memory use.
Optimize JVM Memory Settings
In addition to using efficient parsing methods, you can fine-tune JVM options to manage memory better. Setting appropriate heap sizes can help control memory allocation for larger files.
Common JVM options include:
-Xms256m
: Sets the initial memory allocation.-Xmx1024m
: Sets the maximum memory allocation.
For example:
java -Xms256m -Xmx1024m -cp yourapplication.jar com.example.Main
My Closing Thoughts on the Matter
Parsing large XML files doesn't have to be a memory-consuming ordeal. By using SAX or StAX, developers can efficiently handle XML data without the risk of running into memory issues. Each approach has its own merits, so consider your specific use case when choosing between them.
When dealing with large datasets, remember:
- Avoid DOM parsing for massive XML files.
- Use SAX for lower memory consumption.
- Consider StAX for a more controlled, pull-based approach.
- Optimize JVM memory settings to support larger files.
For further reading, you may refer to Oracle's XML Parsing in Java or XML Processing in Java for comprehensive guides on the subject.
By implementing these strategies, you can work efficiently with large XML files and maintain your application's performance.
Checkout our other articles