Debugging Challenges with EE JSP Servlet Integration
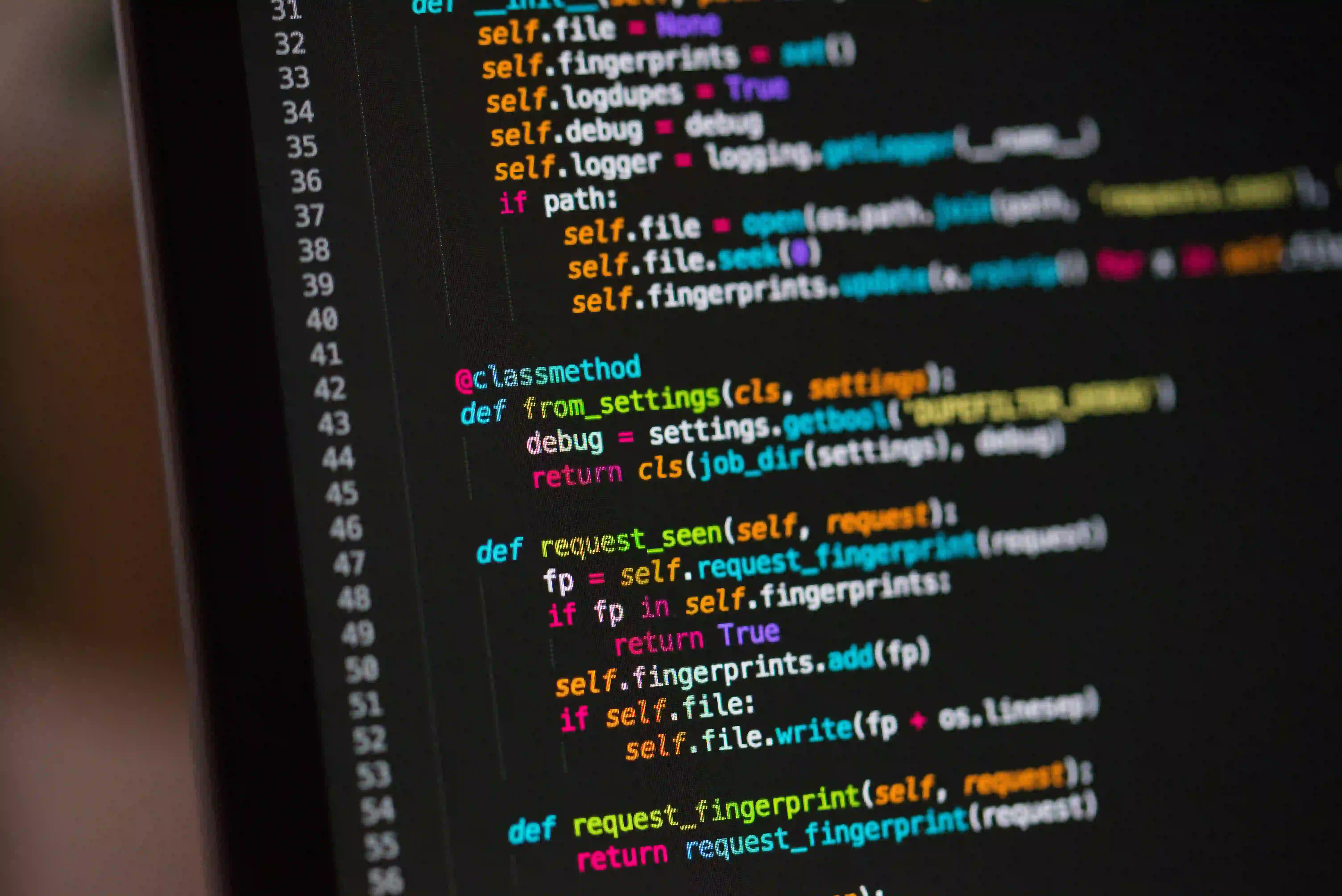
Debugging Challenges with EE JSP Servlet Integration
In the world of Java web application development, understanding how to effectively debug your code can greatly enhance productivity and reduce downtimes. One of the most common configurations in Java EE is the integration of JavaServer Pages (JSP) and Servlets. This combination allows developers to build dynamic web applications with ease, but it also introduces specific challenges that can complicate the debugging process. In this blog post, we'll explore some of these challenges and provide actionable strategies to tackle them.
Understanding the JSP and Servlet Lifecycle
Before we dive into debugging challenges, it is essential to grasp the lifecycle of JSPs and Servlets, as understanding this foundation can simplify the debugging process.
JSP Lifecycle Overview
- Translation: When a JSP is requested, the server converts it into a Servlet.
- Compilation: The server compiles the Servlet code into bytecode.
- Initialization: The server creates an instance of the Servlet and calls its
init()
method. - Request Handling: The server will call the
service()
method for every request, which then differentiates HTTP methods. - Destroy: Finally, the
destroy()
method is called before the Servlet is removed from memory.
Servlet Lifecycle Overview
Serves follow a lifecycle similar to JSPs, but with a more manual approach in terms of coding.
- Initialization: The container creates a Servlet instance and initializes it.
- Request Processing: Each incoming request is handled in a
doGet()
ordoPost()
method. - Destruction: When no longer needed, the Servlet is destroyed.
Understanding these processes informs debugging because we can anticipate where issues might arise.
Common Debugging Challenges in JSP and Servlet Integration
1. Error Tracking in JSP Compilation
Problem: Errors seem to occur mysteriously when JSP pages are compiled into Servlets.
Solution: Pay close attention to server logs. When a JSP fails to compile, the logs typically provide stack traces that are invaluable for identifying syntax or compilation errors.
Example:
<%
String name = request.getParameter("name");
out.println("Hello, " + name);
%>
Suppose the above code snippet throws an error because name
is not in request. Carefully check the logs for NullPointerException
, which often indicates missing request parameters.
2. Session Management Issues
Problem: Keeping track of user sessions can become convoluted, especially in a complex web application.
Solution: Use debugging tools to inspect session attributes. Logging session creation, modification, and destruction helps isolate session-related bugs.
Example of logging session attributes:
HttpSession session = request.getSession();
session.setAttribute("username", "JohnDoe");
System.out.println("Session username: " + session.getAttribute("username"));
3. Forwarding and Redirecting Challenges
Problem: Understanding the difference between RequestDispatcher.forward()
and response.sendRedirect()
is crucial.
Solution: Misusing these can lead to unexpected behavior. Use forward()
if you wish to maintain the URL without changing it, and sendRedirect()
to change the URL for a new request.
Example:
// Correct way to forward
RequestDispatcher dispatcher = request.getRequestDispatcher("nextPage.jsp");
dispatcher.forward(request, response);
Note: If the page is not rendering as expected, ensure you're using the correct path relative to the web application context.
4. Debugging with Logging
Problem: Insufficient logging can make identifying the root cause of errors time-consuming.
Solution: Employ a robust logging framework such as SLF4J alongside Logback or Log4J2 to provide comprehensive logging.
Example:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyServlet extends HttpServlet {
private static final Logger logger = LoggerFactory.getLogger(MyServlet.class);
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
logger.info("Handling GET request");
// more code...
}
}
5. Concurrency Issues
Problem: Servlets are often shared across multiple threads, which can lead to race conditions.
Solution: Avoid instantiating mutable objects directly in the Servlet. Instead, store them in thread-safe data structures or utilize synchronized
blocks.
The Last Word: Strategies for Effective Debugging
- Thorough Logging: Ensure debugging logs are clear and actionable. Always include timestamps and request identifiers.
- Regular Unit Testing: Use JUnit and Mockito to perform unit tests on individual components to catch issues early.
- Integrated Development Environments (IDEs): Use IDEs like IntelliJ IDEA or Eclipse, which come equipped with debugging tools that simplify tracking state and isolating bugs.
- Remote Debugging: Use remote debugging capabilities to attach to your Java server and inspect variables and control flows in real-time.
Additional Resources
- Java EE Documentation
- Servlets and JavaServer Pages (JSP)
- Logback Documentation
By understanding the nuances of JSP and Servlet integration and embracing practical debugging strategies, you not only enhance your coding skills but also contribute to building more robust Java web applications. Happy coding!