Resolving Maven Cargo Plugin Failures in Integration Tests
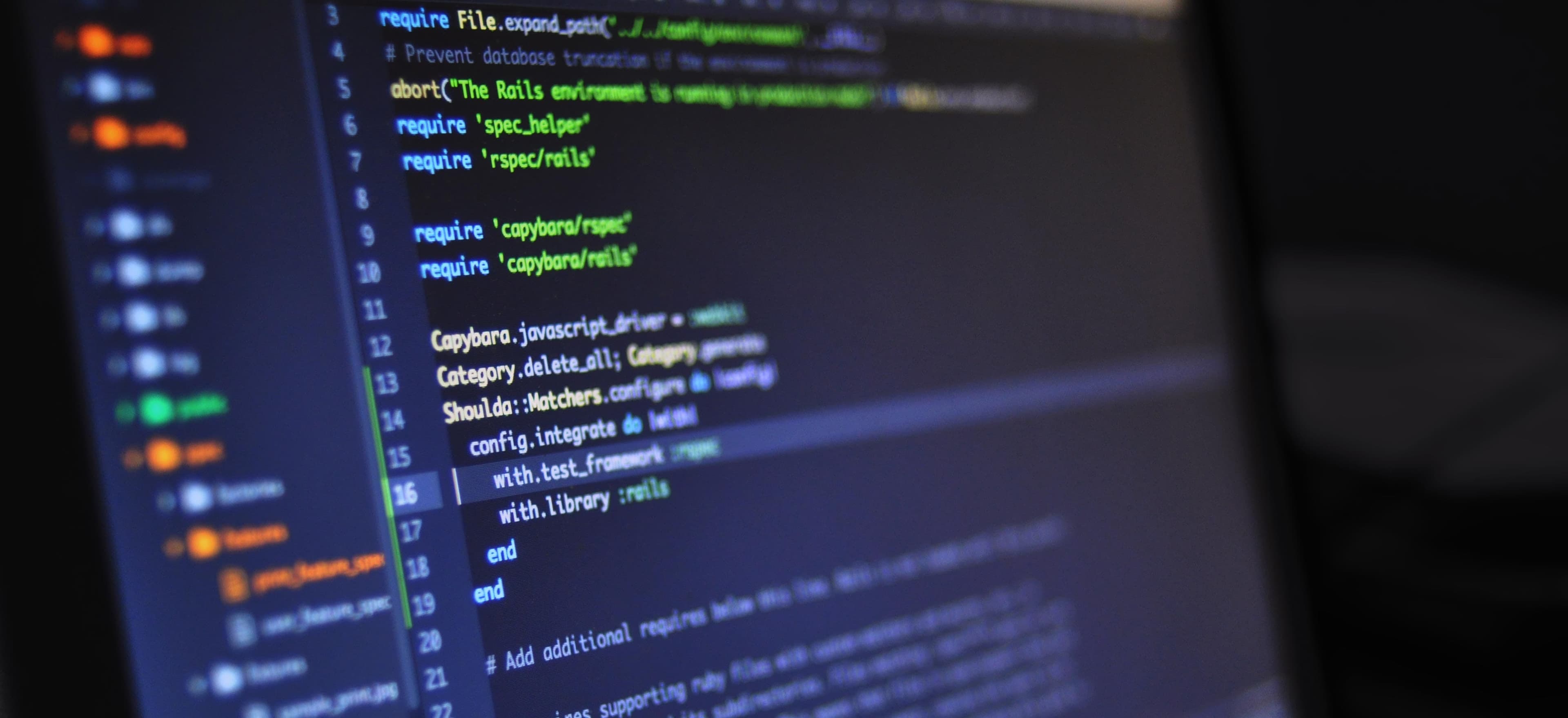
- Published on
Resolving Maven Cargo Plugin Failures in Integration Tests
In the world of Java development, integration testing is a crucial component of a smooth deployment pipeline. When working with integration tests, the Apache Maven Cargo Plugin is a popular choice. However, like many things in software development, it can come with its own set of challenges. This blog post aims to elucidate common Maven Cargo Plugin failures during integration testing and provide practical solutions along with code snippets to make your debugging process simpler.
What is the Maven Cargo Plugin?
The Maven Cargo Plugin allows developers to deploy and manage their applications in various container environments. It seamlessly integrates with Maven to simplify the process of executing integration tests on a running server instance. While it is a robust tool, failures and unexpected behavior may occur.
Why Use Maven Cargo?
Using the Maven Cargo Plugin during integration testing facilitates:
- Automated Deployment: Automates the deployment of applications, eliminating manual errors.
- Multiple Container Support: Provides compatibility with various containers like Tomcat, JBoss, and Glassfish.
- Remote and Local Deployment: Facilitates deploying both local and remote configurations, allowing for flexibility.
For detailed documentation, refer to the Maven Cargo Plugin documentation.
Common Issues and Their Solutions
Before digging deeper into solutions, let's explore some common failures that developers might face when using the Maven Cargo Plugin.
1. Container Not Starting
Symptoms: Integration tests fail to run because the configured application server is not starting.
Why it Occurs:
- Incorrect configurations in
pom.xml
. - Port conflicts where the desired port is already in use.
Solution:
Ensure the configuration details in the pom.xml
are accurate. Here is an example configuration:
<plugin>
<groupId>org.apache.cargo</groupId>
<artifactId>cargo-maven2-plugin</artifactId>
<version>1.10.2</version>
<configuration>
<deployables>
<deployable>
<groupId>your.app</groupId>
<artifactId>your-artifact</artifactId>
<type>war</type>
</deployable>
</deployables>
<container>
<containerType>jetty</containerType>
</container>
<configuration>
<type>standalone</type>
<properties>
<property>
<name>cargo.port</name>
<value>8080</value>
</property>
</properties>
</configuration>
</configuration>
</plugin>
Key Points:
- Ensure the
type
andcontainerType
correspond to your target deployment server. - Change the
cargo.port
if it clashes with another application running on your machine.
2. Timeouts During Execution
Symptoms: Maven hangs during the execution phase and eventually times out.
Why it Occurs: This usually happens if the application takes too long to start, possibly due to heavy configuration or resource limitations.
Solution:
Increase the timeout settings in the pom.xml
file:
<configuration>
...
<timeout>600</timeout> <!-- Increase timeout to 600000 milliseconds -->
...
</configuration>
3. Incorrect Server URL
Symptoms: Integration tests fail due to connectivity issues when attempting to access the application.
Why it Occurs: The endpoint URL specified in the integration tests might not align with the deployment settings or contain typographical errors.
Solution: Ensure that your integration tests are configured to hit the correct URL. Here’s a small snippet for HTTP requests in tests:
@Test
public void testApplicationEndpoint() throws Exception {
URL url = new URL("http://localhost:8080/your-context-path/api/resource");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
assertEquals(200, conn.getResponseCode());
}
4. Dependency Issues
Symptoms: ClassNotFoundExceptions occur when running integration tests.
Why it Occurs: The application may depend on certain libraries that are not included in the deployment.
Solution:
Add the necessary dependencies in your pom.xml
. Example:
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>example-library</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
Also, consider packaging the WAR file with all dependencies to ensure they are available during runtime.
5. Failed Deployment
Symptoms: The application fails to deploy despite providing correct configuration.
Why it Occurs: Possible causes could be outdated deployment artifacts or issues with the server itself.
Solution: Before running the tests, perform a clean build:
mvn clean package
mvn cargo:deploy
This ensures that you are deploying the latest version of your application.
Running Integration Tests
Once you've resolved the above issues, it's time to run your integration tests with Maven Cargo. Here’s the command to do that:
mvn verify -P cargo
Make sure the profile with Cargo configurations is correctly specified.
My Closing Thoughts on the Matter
Improving integration testing with the Maven Cargo Plugin can significantly enhance your development workflow. By understanding the common failures and optimally utilizing the plugin, you can ensure smoother deployments and a more resilient testing strategy.
For further reading on dealing with deployment issues, check out this comprehensive guide on the Maven Cargo Plugin from Baeldung.
With persistence and the right strategies, Maven Cargo Plugin failures can be effectively managed, bringing you one step closer to having a robust deployment and testing pipeline.
Remember, the key to resolving these issues lies in systematic troubleshooting and the continuous evolution of your testing practices. Happy coding!
Checkout our other articles