Navigating Common Pitfalls in Spring Security Bean Calls
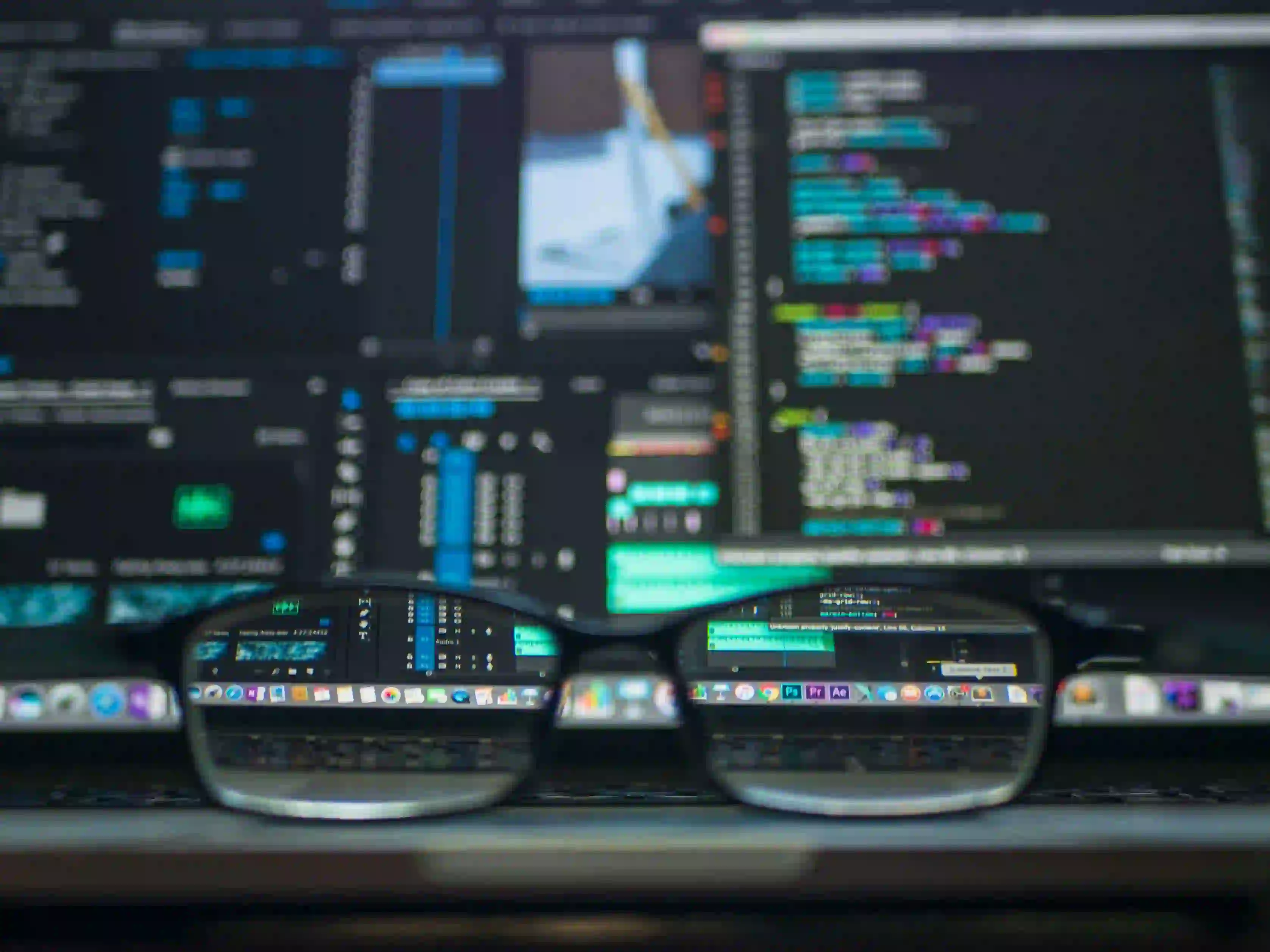
Navigating Common Pitfalls in Spring Security Bean Calls
When working with Spring Security, it is essential to understand how to effectively manage bean calls. Proper configuration ensures that your application remains secure and functions smoothly. In this post, we will dive into some common pitfalls associated with Spring Security bean calls and ways to avoid them. By the end, you’ll have a clearer roadmap for implementing Spring Security in your applications.
Understanding Spring Security Beans
Spring Security is a powerful framework that allows you to secure your applications effortlessly. At its core, it relies heavily on the concept of beans. These are Spring-managed objects that are instantiated, assembled, and managed by the Spring IoC container.
Spring Security offers various beans, including:
- SecurityFilterChain: Manages security filters for HTTP requests.
- AuthenticationManager: Manages authentication processes.
- UserDetailsService: Loads user-specific data.
Key Spring Security Components
Before we dive into common pitfalls, let's briefly discuss key components relevant to security in a Spring application.
-
WebSecurityConfigurerAdapter: This is a key class where you can configure security settings. It allows the overriding of methods to customize security definitions.
-
AuthenticationProvider: It handles the process of authenticating users.
-
PasswordEncoder: This component manages password encryption.
Each of these components relies on proper bean management, making their configuration critical.
Common Pitfalls in Spring Security Bean Calls
1. Lack of Proper Bean Definition
One of the most common pitfalls is failing to define the beans correctly. This can lead to a variety of errors, including NoSuchBeanDefinitionException
.
Example: Defining a Custom Authentication Provider
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
// Configuring In-memory user store
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}admin").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/", "/home").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
}
Comments:
- In this example, the
configure
methods define how authentication and authorization operations will function. - Here, an in-memory authentication manager is defined, which is suitable for testing and development.
2. Bean Scope Misunderstanding
Another frequent pitfall lies in misunderstanding the scope of Spring beans. For instance, if you do not specify the correct scope, your bean may not behave as intended. By default, Spring beans are singletons, meaning there will only be one instance of the bean per Spring context.
@Bean
@Scope("prototype")
public MyCustomService myCustomService() {
return new MyCustomService();
}
Comments:
- In this snippet, a prototype scope is defined. Every time the bean is requested, a new instance will be created. Use cases for prototype beans include scenarios needing state that should not be shared.
3. Circular Dependencies
Circular dependencies are another common issue that can cause application startup failures. This arises when two or more beans reference each other in their configuration.
Example of a Circular Dependency:
@Component
public class ServiceA {
@Autowired
private ServiceB serviceB;
}
@Component
public class ServiceB {
@Autowired
private ServiceA serviceA;
}
Comments:
- To resolve circular dependencies, consider refactoring your code to separate the concerns or using constructor injection instead of field injection.
- Circular dependencies could lead to
BeanCurrentlyInCreationException
.
4. Missing Password Encoding
A key aspect of securing your application is password storage. Failing to encode passwords can expose user credentials. Always use PasswordEncoder
for encoding passwords.
Example of Password Encoding:
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
// Later used in UserDetailsService
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
String encodedPassword = passwordEncoder().encode(user.getPassword());
// Use the encoded password for authentication
}
Comments:
- In this example,
BCryptPasswordEncoder
is used, which is one of the most secure ways to encrypt passwords. This prevents potential data breaches.
5. Ignoring Security Context
Failing to check the Security Context can lead to unauthorized access. Always ensure you verify user roles and permissions before granting access to sensitive resources.
Example of Checking Security Context:
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
public void secureMethod() {
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (auth != null && auth.isAuthenticated()) {
// Perform secured task
} else {
throw new SecurityException("User is not authenticated.");
}
}
Comments:
- This method ensures that only authenticated users can access protected functionality.
Closing Remarks
Securing your Spring application can be straightforward if you pay attention to common pitfalls. Understanding how to effectively handle Spring Security beans is key to maintaining a secure application environment.
Here’s a quick recap of what we discussed:
- Ensure proper bean definitions to avoid
NoSuchBeanDefinitionException
. - Correctly understand bean scopes.
- Prevent circular dependencies by refactoring your code.
- Always encode passwords to protect user data.
- Validate authentication and authorization via the Security Context.
Implementing these best practices will help you navigate the complexities of Spring Security with greater ease and ensure your application's security integrity.
For more information on Spring Security configurations, you can check out the official Spring Security documentation. Happy coding!
This post aimed to provide insights into the complexities of managing Spring Security beans with a focus on potential pitfalls and best practices. By consistently applying these principles, you can enhance the security posture of your Spring applications.