Cutting GC Overhead: 5 Essential Coding Hacks
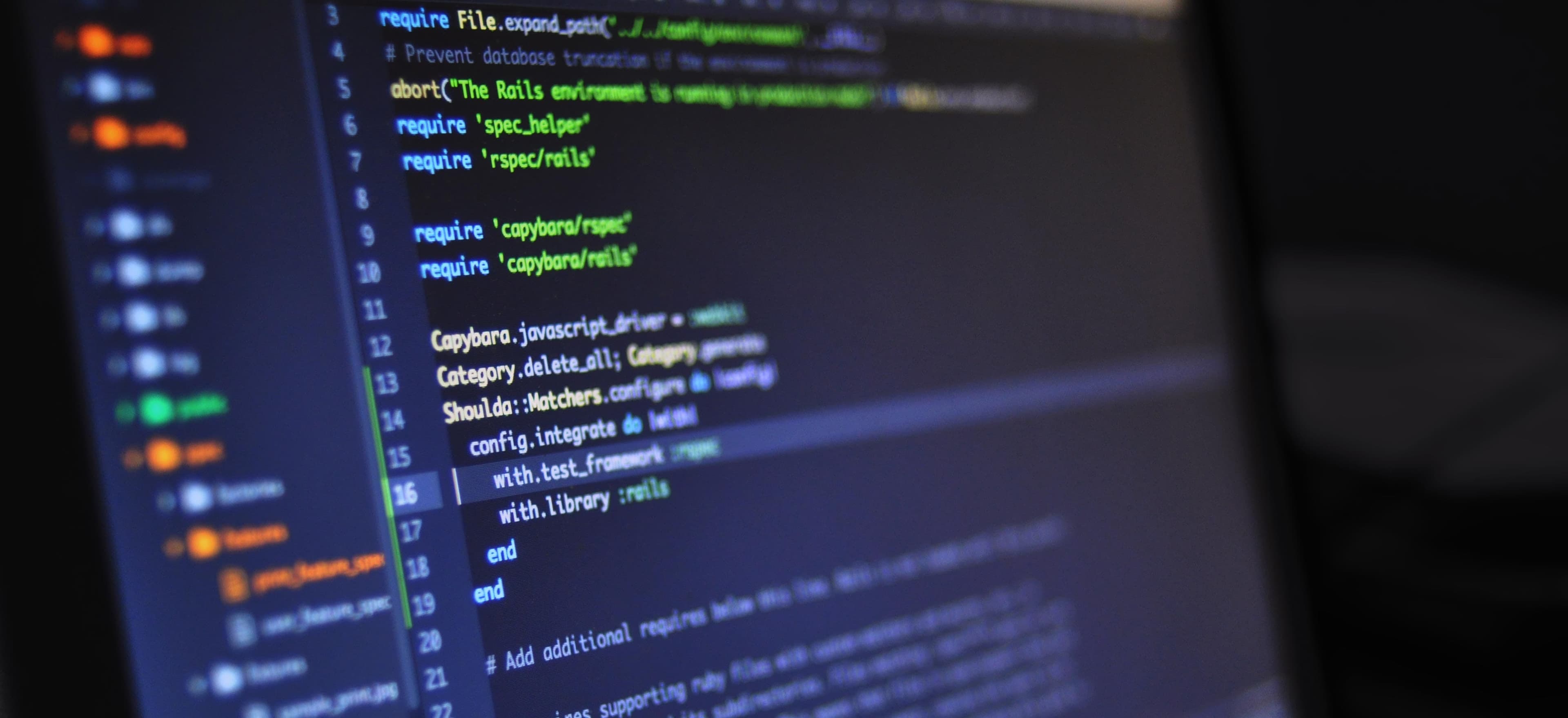
- Published on
Cutting GC Overhead: 5 Essential Coding Hacks
Garbage Collection (GC) is crucial for memory management in Java, but it can also be a source of performance bottlenecks if not managed properly. As Java developers, we often focus on writing clean, efficient code, but the way we handle memory can significantly affect the overall performance of our applications. In this post, we will explore five essential coding hacks to cut GC overhead and improve your Java application's responsiveness and efficiency.
1. Minimize Object Creation
Why It Matters
Every time you create a new object, the Java Virtual Machine (JVM) must allocate memory for it. Excessive object creation can lead to frequent garbage collection cycles, which in turn can degrade application performance.
Coding Hack
To minimize object creation, consider using static factories or object pooling. Here’s an example:
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
class Connection {
// Simulated connection class
}
class ConnectionPool {
private final BlockingQueue<Connection> pool;
public ConnectionPool(int size) {
pool = new ArrayBlockingQueue<>(size);
for (int i = 0; i < size; i++) {
pool.add(new Connection());
}
}
public Connection getConnection() throws InterruptedException {
return pool.take(); // Reuses existing connections
}
public void releaseConnection(Connection conn) {
pool.offer(conn); // Returns connection to the pool
}
}
Explanation
In this example, ConnectionPool
manages a fixed number of Connection
objects. By reusing connections instead of creating new ones, we significantly reduce the frequency and overhead of garbage collection.
Additional Resources
You can read more about Object Pooling to get a deeper understanding of how to implement this concept effectively.
2. Use Primitive Types Instead of Wrapper Classes
Why It Matters
Wrapper classes like Integer
, Double
, etc., introduce unnecessary overhead because they are objects. Using primitive types can significantly reduce memory usage and improve performance.
Coding Hack
Whenever possible, use primitive data types. Here’s an example of when to prefer primitives:
public class SumCalculator {
public int calculateSum(int[] numbers) {
int sum = 0; // Using primitive int
for (int number : numbers) {
sum += number;
}
return sum;
}
}
Explanation
In this example, we use an array of primitive int
values instead of Integer
. This change reduces the memory footprint and eliminates the additional garbage collection burden associated with object wrappers.
3. Utilize StringBuilder for String Concatenation
Why It Matters
In Java, strings are immutable. This means that every time you concatenate two strings using the +
operator, a new string object is created, leading to potential memory overhead.
Coding Hack
Use StringBuilder
for string concatenation instead of the +
operator. Here’s how you can implement it:
public class StringConcatenation {
public String concatenateWords(String[] words) {
StringBuilder result = new StringBuilder();
for (String word : words) {
result.append(word).append(" "); // Efficiently concatenates strings
}
return result.toString().trim(); // Convert to String and trim the final space
}
}
Explanation
In this code, StringBuilder
allows for dynamic string construction without creating excessive intermediate String
objects, reducing GC overhead in operations that involve significant concatenation.
4. Leverage transient
for Non-Serializable Fields
Why It Matters
When an object is serialized, all its fields are by default serialized. If an object has large or unnecessary fields that do not require persistence, marking them as transient
helps avoid serialization overhead.
Coding Hack
Use the transient
keyword for fields that don't need to be serialized. For example:
import java.io.Serializable;
class UserProfile implements Serializable {
private String username;
private transient String password; // Marked transient to skip serialization
public UserProfile(String username, String password) {
this.username = username;
this.password = password;
}
}
Explanation
In this example, marking password
as transient prevents it from being included during serialization. This reduces the memory overhead associated with serialization and deserialization processes.
5. Optimize Collections Usage
Why It Matters
Choosing the right collection type can greatly influence memory usage and performance. Some collections have different memory requirements and operational complexities.
Coding Hack
Select appropriate collection types based on your use case. Here’s a simple example:
import java.util.HashMap;
import java.util.Map;
class UserRegistry {
private Map<String, User> userMap;
public UserRegistry() {
userMap = new HashMap<>(); // O(1) average-time complexity for operations
}
public void addUser(String username, User user) {
userMap.put(username, user);
}
public User getUser(String username) {
return userMap.get(username);
}
}
Explanation
Using a HashMap
for fast lookup reduces the overhead of searching through a list. Always analyze your performance needs; for instance, if you require sorted data, consider a TreeMap
, but bear in mind its O(log n) complexity.
Final Thoughts
In Java development, minimizing garbage collection overhead is essential to building efficient applications. By applying these five coding hacks—minimizing object creation, using primitive types, leveraging StringBuilder
, marking fields as transient
, and optimizing your collections—you can enhance your Java application’s performance while reducing memory consumption.
Final Thoughts
Remember, good performance starts at the code level. Regular profiling, optimization, and understanding the underlying mechanisms of Java's memory management and garbage collection can lead to better application performance.
For more insights into Java performance optimization, check out Java Performance Tuning. Happy coding!
Checkout our other articles