Navigating the Thin Line: Accidental vs. Essential Complexity
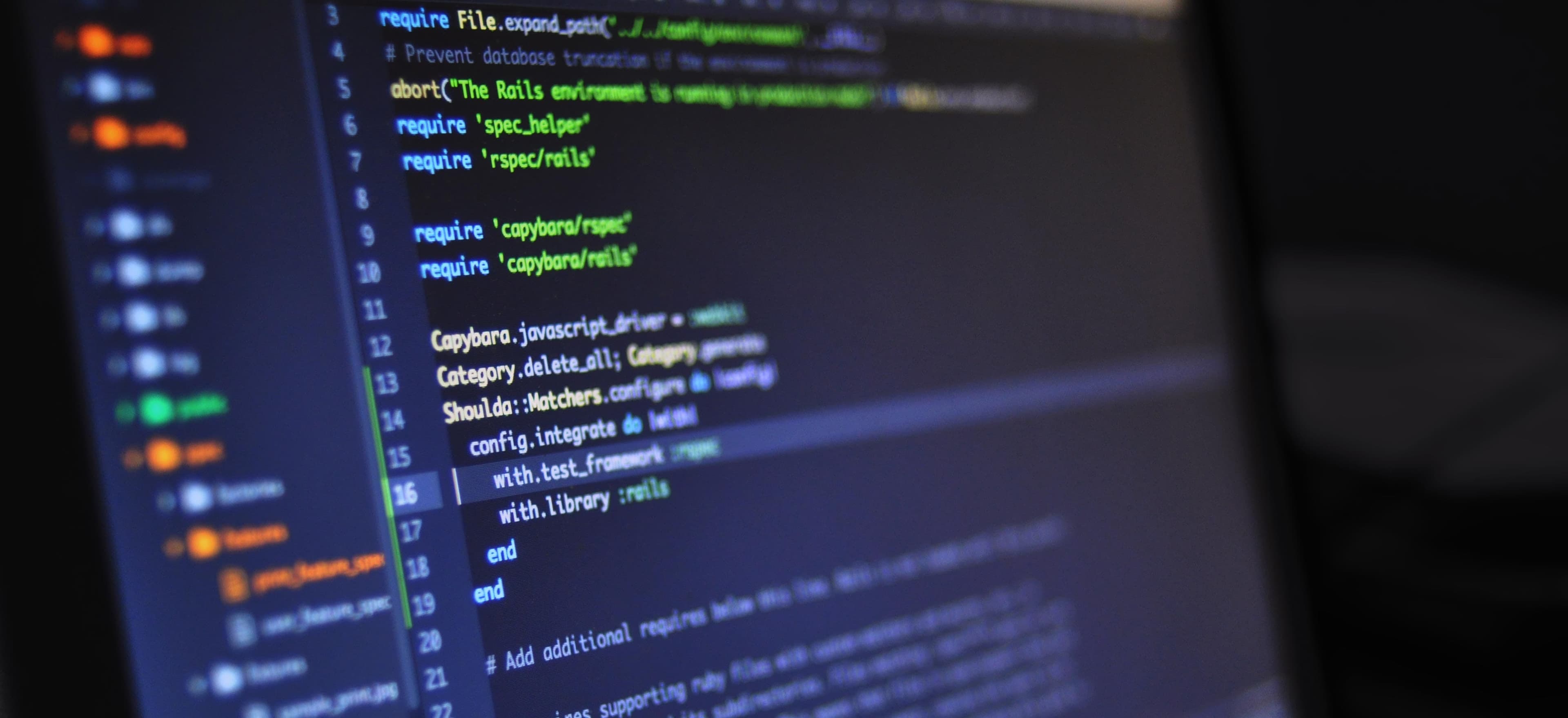
- Published on
Navigating the Thin Line: Accidental vs. Essential Complexity in Software Development
In the realm of software development, one of the most enduring challenges we face is navigating the delicate balance between accidental complexity and essential complexity. Understanding this distinction is crucial for creating efficient, maintainable, and scalable software systems. In this blog post, we will delve into the definitions of both types of complexity, explore real-world examples in Java, and provide insights into how developers can minimize accidental complexity while embracing essential complexity.
What is Complexity in Software Development?
Complexity in software refers to the degree of intricacy involved in a system, which can impact its functionality, performance, and maintainability. Complexity can be categorized into two types:
Essential Complexity
Essential complexity is the complexity inherent to the problem being solved. It arises from the nature of the problem domain itself. For example, creating an algorithm for pathfinding in a maze will have a certain level of complexity simply because the problem demands it. This complexity cannot be avoided; however, it can often be managed through effective design.
Takeaway: Essential complexity is unavoidable and often necessary for solving real-world problems.
Accidental Complexity
Accidental complexity, on the other hand, is the complexity introduced by poor design decisions, inadequate tools, or unnecessary features that don’t contribute to solving the problem at hand. It can arise from an overcomplicated architecture, convoluted code structure, or extensive frameworks that lead to unnecessary overhead.
Takeaway: Accidental complexity can be reduced or eliminated through careful design and better tools.
Understanding the Distinction
Essential Complexity Example
Consider a common problem: sorting data. If you are tasked with sorting a large dataset, sorting algorithms like QuickSort or MergeSort inherently possess essential complexity due to the nature of sorting operations. Here's a basic implementation of QuickSort in Java:
public class QuickSort {
public void sort(int[] array, int low, int high) {
if (low < high) {
int pi = partition(array, low, high);
sort(array, low, pi - 1);
sort(array, pi + 1, high);
}
}
private int partition(int[] array, int low, int high) {
int pivot = array[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (array[j] < pivot) {
i++;
swap(array, i, j);
}
}
swap(array, i + 1, high);
return i + 1;
}
private void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
Here, the QuickSort algorithm has inherent complexity related to the concept of sorting. No matter how well we code it, sorting will always require some level of complexity based on the input data.
Accidental Complexity Example
Conversely, accidental complexity can manifest when we choose an overly complicated solution to a data storage problem, such as integrating multiple libraries and frameworks unnecessarily. Consider the following example of implementing a simple configuration manager in Java:
import java.util.Properties;
import java.io.InputStream;
import java.io.IOException;
public class ConfigManager {
private Properties properties = new Properties();
public ConfigManager(String configFilePath) {
try (InputStream input = getClass().getClassLoader().getResourceAsStream(configFilePath)) {
if (input == null) {
System.out.println("Sorry, unable to find " + configFilePath);
return;
}
properties.load(input);
} catch (IOException ex) {
ex.printStackTrace();
}
}
public String getProperty(String key) {
return properties.getProperty(key);
}
}
While the core functionality here is straightforward, accidental complexity could arise if we choose to use a large, heavyweight framework like Spring for simple property management. The inclusion of a large framework for a simple task can lead to maintenance challenges and a steep learning curve for new developers.
The Role of Design Patterns
Implementing design patterns is an effective strategy for distinguishing between essential and accidental complexities. Patterns such as Singleton, Factory, and Strategy can help to encapsulate complexity and provide clarity to the codebase.
Singleton Example
The Singleton pattern ensures a class has only one instance and provides a global point of access to it.
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
This pattern is essential when you need a single instance (like a configuration manager). However, if used when not necessary, it could introduce accidental complexity.
Reducing Accidental Complexity
To minimize accidental complexity, developers can adopt several best practices:
-
Keep It Simple: Favor simplicity over complexity. Avoid over-engineering solutions.
-
Use Established Libraries: Leverage well-tested libraries instead of building complex solutions in-house.
-
Embrace SOLID Principles: Design your code according to SOLID principles. This encourages a modular architecture that’s easier to understand and maintain.
-
Code Reviews: Regular code reviews help in identifying areas of accidental complexity before they grow.
-
Documentation: Always document complex algorithms and modules. Clear documentation can reduce the mental overhead for team members navigating the codebase.
Wrapping Up
In conclusion, understanding the distinction between accidental and essential complexity is vital for any software developer. While essential complexity is often unavoidable, being aware of and minimizing accidental complexity can lead to a more maintainable and efficient codebase. Java developers, in particular, can use design patterns and best practices to manage complexity effectively.
If you’re interested in more about design patterns and best coding practices, check out the Design Patterns in Java resource for deeper insights.
By continuously striving for simplicity, embracing the necessary complexities, and refining our practices, we can navigate the challenging landscape of software development, yielding robust, effective, and maintainable applications. Happy coding!
Checkout our other articles