Mastering Java Heap Dumps: A Guide to Efficient Analysis
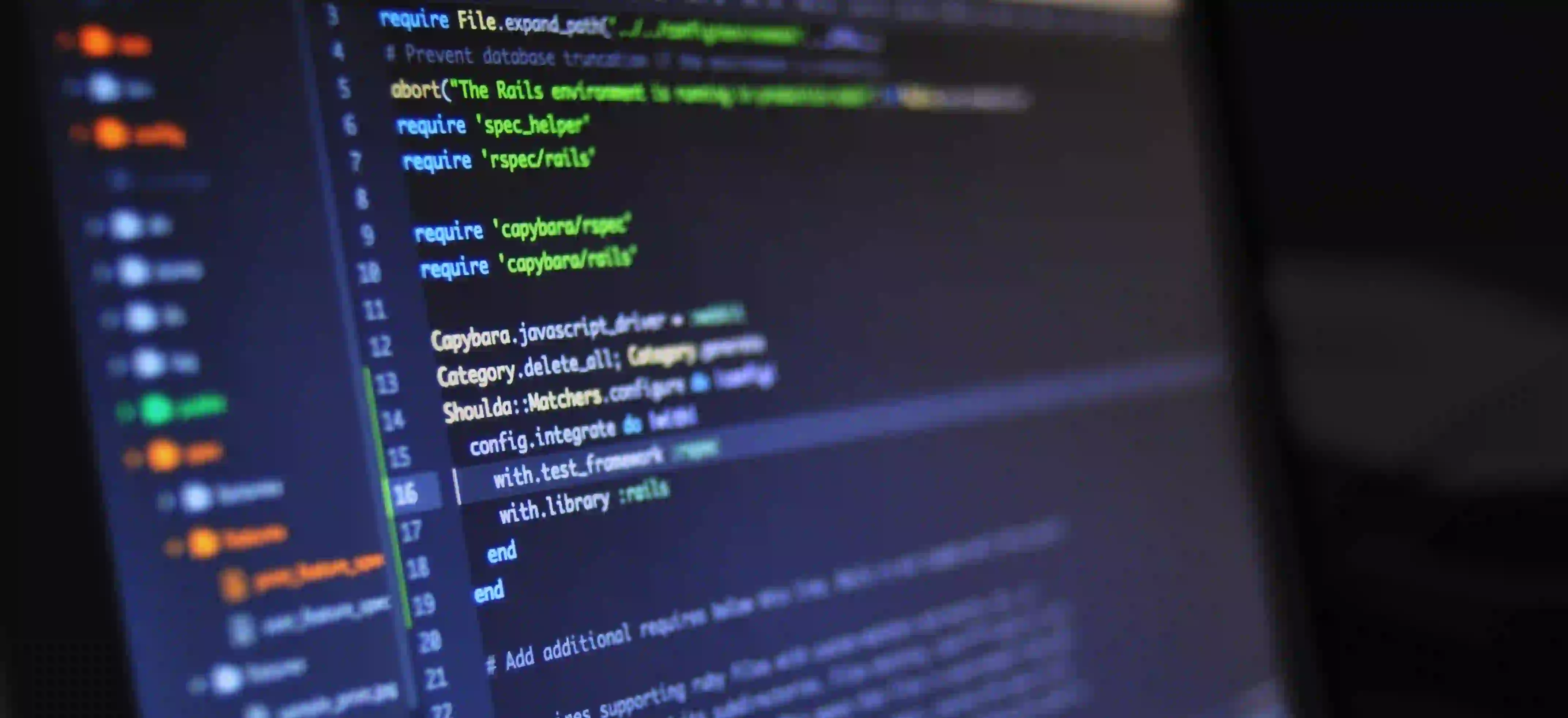
Mastering Java Heap Dumps: A Guide to Efficient Analysis
When dealing with Java applications, developers often encounter performance issues that can be challenging to diagnose. One of the most powerful tools at your disposal for understanding memory usage and performance bottlenecks is the heap dump. This blog post will delve into what heap dumps are, how to generate them, and the methods to analyze them effectively for optimal Java application performance.
What is a Heap Dump?
A heap dump is a snapshot of the Java Virtual Machine (JVM) memory at a specific point in time. It contains information about all the objects that are in memory, including their states, classes, and references. By analyzing heap dumps, developers can identify memory leaks, assess object retention, and ultimately, enhance the performance of their applications.
Why Analyze Heap Dumps?
- Memory Leaks: Prolonged running applications can sometimes retain more objects than necessary, leading to memory leaks. Heap dumps can help identify these objects.
- Garbage Collection Tuning: Understanding which objects are retained in memory can provide insights into how to optimize your garbage collection process.
- Performance Troubleshooting: A heap dump can reveal bottlenecks in application performance, guiding developers to areas requiring optimization.
Generating Heap Dumps
You can generate heap dumps in various ways, depending on your environment and needs. Here are three common methods:
1. Using JVM Options
You can instruct the JVM to generate a heap dump automatically when an OutOfMemoryError occurs:
java -XX:+HeapDumpOnOutOfMemoryError -XX:HeapDumpPath=/path/to/dump hotswap
In this configuration, the HeapDumpPath
specifies where the dump file will be stored. This method is straightforward and ensures you will have a dump to analyze whenever your application experiences critical memory issues.
2. Using jmap
The jmap
tool is part of the JDK and can manually generate a heap dump for a running Java process. Use the following command:
jmap -dump:live,format=b,file=heapdump.hprof <pid>
live
: Indicates whether to include only live objects.format=b
: Output in binary format.file=heapdump.hprof
: The name of the file to which the heap dump will be saved.<pid>
: The process ID of the Java application.
3. Using Java Development Tools (JVisualVM)
JVisualVM provides a graphical interface for monitoring and analyzing Java applications. To generate a heap dump:
- Open JVisualVM.
- Locate your running application under the "Local" node.
- Right-click on the application and select "Heap Dump."
This user-friendly method makes it easy for developers who prefer a GUI over command-line tools.
Analyzing Heap Dumps
Once you've generated a heap dump, the next step is analysis. There are several tools available for analyzing heap dumps effectively.
1. Eclipse Memory Analyzer (MAT)
Eclipse Memory Analyzer (MAT) is an open-source tool used for analyzing Java heap dumps. Here’s how to use it:
- Install MAT: Download it from the Eclipse website.
- Open the Heap Dump: Launch MAT and load your heap dump file.
- Leak Suspects Report: Use this feature to analyze memory usage and identify potential memory leaks.
Here’s a simple example of how you might find memory leaks:
public class MemoryLeakExample {
private static List<String> leakList = new ArrayList<>();
public static void keepAddingStrings() {
while (true) {
leakList.add("Leaking memory: " + new String(new char[1000]));
}
}
}
By examining the heap dump, you can see how leakList
retains references to many string objects, making it ideal for identifying the allocation of unnecessary memory.
2. VisualVM
VisualVM is another tool that provides real-time monitoring, profiling, and analysis of Java applications.
To analyze a heap dump:
- Open VisualVM and select the application.
- Navigate to the “Monitor” tab to view memory usage.
- Click the "Heap Dump" button to capture the dump.
- Analyze the dump similar to MAT.
3. JProfiler
JProfiler is a commercial tool that provides in-depth insights into Java applications. The features include:
- Allocation tracking
- Memory leak detection
- Object reference analysis
The straightforward interface allows quick navigation through object instances and memory usage.
Tips for Effective Heap Dump Analysis
- Investigate Large Objects: Focus on large objects that consume significant amounts of memory.
- Look For High Retention Counts: Objects with high retention counts may indicate memory leaks.
- Use Filters Wisely: Apply filters in tools like MAT to narrow down your analysis.
- Stack Traces: Analyze object stack traces to understand object references better.
- Compare Heap Dumps: Generating heap dumps over time can help compare memory states, making it easier to identify growing problems.
Final Thoughts
Heap dumps are invaluable assets when it comes to diagnosing memory issues within Java applications. Their ability to provide insight into object allocation and reference relationships equips developers with the information needed to tackle performance challenges effectively.
By mastering the generation and analysis of heap dumps, you can enhance your Java applications, reduce memory-related errors, and ultimately deliver a more stable and efficient product. For further reading, consider exploring Oracle's official documentation on JVM options or the comprehensive guide on Eclipse MAT.
Remember, effective memory management is not just about fixing leaks; it's about laying the foundation for robust and scalable Java applications. Happy coding!