Transforming Ant Builds to Gradle: Common Pitfalls to Avoid
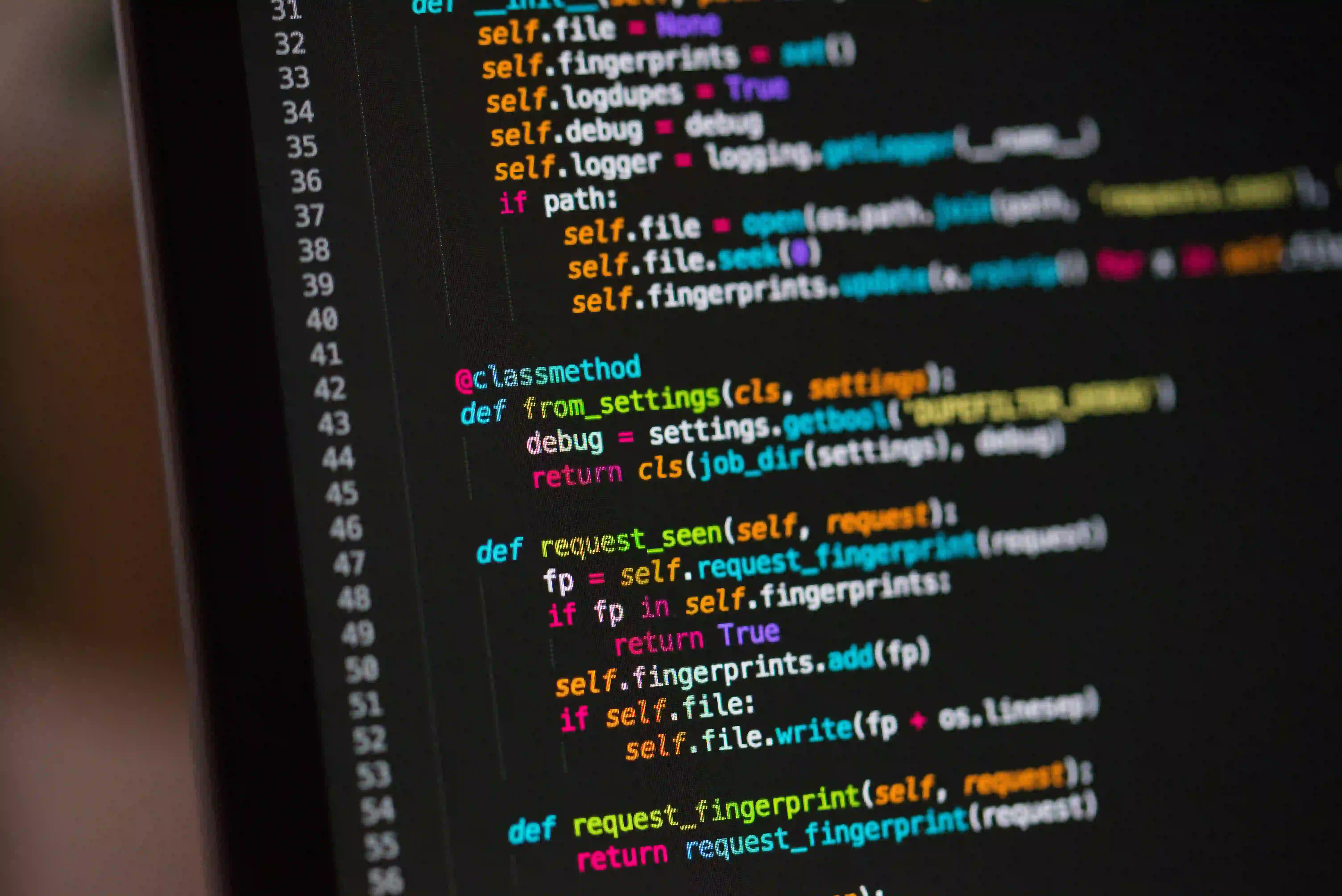
Transforming Ant Builds to Gradle: Common Pitfalls to Avoid
As software builds evolve, developers increasingly shift from Apache Ant to Gradle for its modern features, powerful dependency management, and incremental builds. However, this transition can be fraught with challenges. In this blog post, we will detail common pitfalls to watch out for during the transformation process, ensuring a smoother migration for your team.
Why Move from Ant to Gradle?
Gradle offers a plethora of advantages over Ant:
- Declarative Build Scripts: Unlike the imperative style of Ant, Gradle uses a Groovy/Kotlin DSL, making build scripts concise and readable.
- Dependency Management: Gradle simplifies dependency management and supports Maven and Ivy repositories out of the box.
- Performance: Thanks to incremental builds, Gradle can drastically speed up your builds.
- Extensive Plugin Ecosystem: With plugins for various languages and frameworks, it integrates well into diverse development environments.
While the benefits are significant, let's examine some common pitfalls developers encounter when making the transition.
1. Ignoring Build File Structure
The Ant Way
Ant build files typically consist of a build.xml
that defines various targets. Here’s a simple example:
<project name="SampleProject" default="compile">
<target name="compile">
<javac srcdir="src" destdir="bin" />
</target>
</project>
The Gradle Way
In Gradle, the structure is different. You define tasks in a build.gradle
file. It’s imperative to understand the Gradle lifecycle and build phases. Here’s how you might translate the above Ant target into Gradle:
plugins {
id 'java'
}
sourceSets {
main {
java {
srcDirs = ['src']
}
}
}
tasks.register('compile') {
doLast {
println 'Compiling Java sources...'
}
}
Why Avoid Ignoring Structure?
Gradle files are executed in a specific order based on the lifecycle and task dependencies. Not respecting this order can lead to builds that do not behave as expected.
2. Overcomplicating Dependencies
Ant Handling Dependencies
Ant typically requires several manual steps to manage dependencies, often involving directly downloading JAR files.
<path id="project.classpath">
<fileset dir="lib">
<include name="*.jar"/>
</fileset>
</path>
Gradle Dependency Management
In contrast, Gradle has robust dependency management. Utilizing Gradle, you can declare dependencies clearly in your build.gradle
file:
dependencies {
implementation 'org.apache.commons:commons-lang3:3.12.0'
}
Why Keep It Simple?
Simplifying dependency management reduces complexity and minimizes errors that could arise from manual handling. Gradle's rich DSL enables you to specify dependencies at various scopes (implementation, testImplementation, etc.) cleanly.
3. Neglecting Plugin System
Underestimating Plugins in Gradle
Ant does not have a built-in extensible plugin system, so adding functionalities usually requires manual scripting.
Full Utilization of Gradle Plugins
Gradle's plugin system allows you to leverage community and enterprise plugins easily. For instance, to apply the java
plugin:
plugins {
id 'java'
}
And to apply the application
plugin:
apply plugin: 'application'
mainClassName = 'com.example.Main'
Why Embrace the Plugin System?
By leveraging existing plugins, you save time and reduce the likelihood of introducing bugs. This modularity is a hallmark of modern build tools, facilitating easier maintenance and upgrades.
4. Overlooking Gradle Caching
Ant's Build Process
In Ant, the build process runs each task every time, leading to slower builds as the project grows.
Gradle's Incremental Build Mechanism
Gradle employs a caching mechanism using up-to-date checks. Here's how you can set it up for a task:
tasks.register('processResources') {
inputs.file 'src/main/resources/config.xml'
outputs.file 'build/resources/config.xml'
doLast {
copy {
from 'src/main/resources'
into 'build/resources'
}
}
}
Why Utilize Caching?
Skipping unnecessary task executions drastically reduces build time. Understanding how Gradle manages task inputs and outputs is crucial to leveraging this feature effectively.
5. Skipping Gradle Wrapper
Ant's Static Builds
Ant does not have a standardized way to define the project’s JDK version or Ant version easily.
Gradle Wrapper
Gradle provides a robust solution known as the Gradle Wrapper. By including the wrapper in your project, you ensure that everyone on the team uses the same Gradle version:
gradle wrapper --gradle-version 7.5
Why Use the Gradle Wrapper?
Including the wrapper allows consistent builds across different environments, as it eliminates discrepancies in Gradle versions. This is essential for a stable build environment.
6. Lack of Visibility into Build Execution
Limited Logging in Ant
Ant’s logging capabilities are relatively basic, which can make it difficult to debug build issues.
Gradle's Enhanced Logging
Gradle offers rich logging features. For example:
gradle build --info
Why Monitor Build Execution?
Enhanced logging and the ability to run builds in different modes (quiet, info, debug) help identify issues quickly and effectively. This can lead to a better understanding of the overall build process.
Final Considerations
Transitioning from Ant to Gradle presents a range of benefits, but it also requires careful attention to detail. By avoiding the common pitfalls discussed here—like ignoring build structure, overcomplicating dependencies, and neglecting the usage of plugins—you can streamline your migration process.
With Gradle's powerful features, you not only modernize your build system but also enhance collaboration among your development team. Start small, leverage existing knowledge, and take advantage of Gradle's rich ecosystem to pave the way for successful builds.
For further reading on Gradle, check out the official documentation. Embrace the power of Gradle, and watch your builds transform for the better!