Mastering Syntax: Common Errors and How to Fix Them
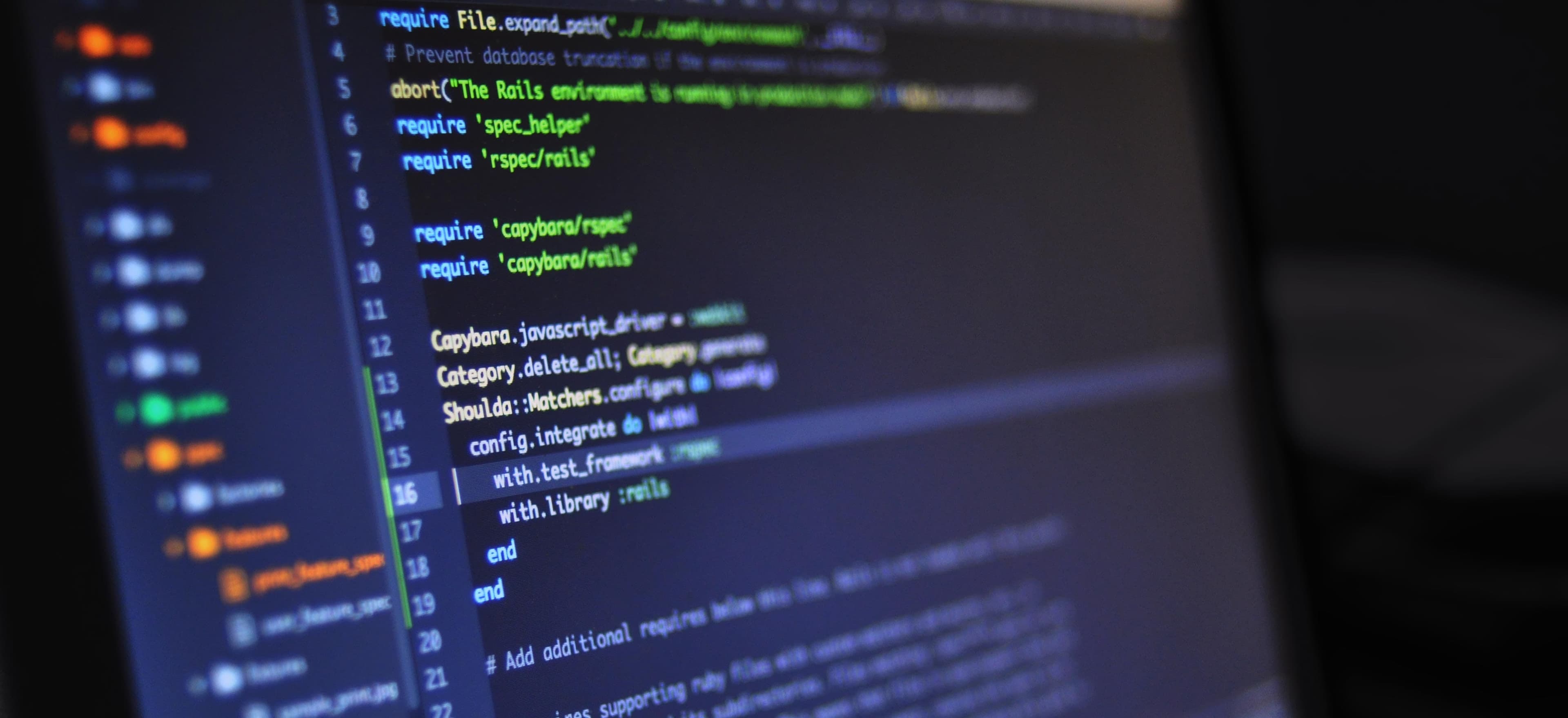
- Published on
Mastering Syntax: Common Java Errors and How to Fix Them
Java is a powerful and widely-used programming language known for its versatility and impact on software development. However, even seasoned developers can run into syntax errors that disrupt workflow. This blog post explores common Java syntax errors, how to identify them, and effective strategies for fixing them.
What is Syntax in Java?
In the context of programming, syntax refers to the set of rules that defines the combinations of symbols that are considered to be correctly structured programs in a given language. Java's syntax is heavily influenced by C and C++.
Understanding syntax is essential because even minor typographical errors can lead to significant issues during code execution. Java is case-sensitive and has a strict structure that must be followed.
Common Java Syntax Errors
1. Missing Semicolons
One of the most frequent syntax errors in Java is forgetting to terminate statements with a semicolon.
public class Example {
public static void main(String[] args) {
int number = 5 // Error: Missing semicolon
System.out.println(number);
}
}
How to Fix: Always remember to place a semicolon at the end of each statement.
int number = 5; // Corrected
System.out.println(number);
2. Mismatched Parentheses, Brackets, and Braces
Not closing parentheses, brackets, or braces can cause syntax errors that are often difficult to trace in larger code blocks.
public class Example {
public static void main(String[] args) {
if (number > 0 { // Error: Mismatched parentheses
System.out.println("Positive");
}
}
}
How to Fix: Always ensure that each opening parenthesis, bracket, or brace has a corresponding closing counterpart.
if (number > 0) { // Corrected
System.out.println("Positive");
}
3. Incorrect Variable Declarations
Java has strict rules regarding data types and variable declarations. Declaring a variable without specifying its type or trying to assign an incompatible value will lead to errors.
public class Example {
public static void main(String[] args) {
number = 5; // Error: Type declaration missing
int number; // Correct order would be to declare it first
}
}
How to Fix: Always declare the variable with its data type before using it.
int number; // Correct declaration
number = 5; // Assignment after declaration
4. Using Reserved Keywords
Java has a list of reserved keywords, and using them as variable names leads to syntax errors.
public class Example {
public static void main(String[] args) {
int class = 10; // Error: 'class' is a reserved keyword
}
}
How to Fix: Avoid using reserved keywords as variable names. Consider prefixes or suffixes to name your variables better.
int myClass = 10; // Corrected variable name
5. Incorrect Use of Comments
While comments do not affect execution, incorrect comment syntax can lead to unexpected behavior in your program.
public class Example {
public static void main(String[] args) {
int number = 5; // This is a comment
/* Error occurs here if the closing tag is missing
System.out.println(number);
}
}
How to Fix: Make sure that block comments are correctly opened and closed, and avoid nesting them.
/* This is a properly formatted comment */
System.out.println(number); // This will execute correctly
How to Diagnose Syntax Errors
1. Compile Your Code Regularly
Frequent compilation helps identify errors sooner rather than later. Each time you compile, the compiler provides feedback on errors and their locations.
2. Read Compiler Error Messages
Compiler messages often indicate the type of error and the line number where it occurred. Pay close attention to these messages as they guide you toward the source of the problem.
3. Use an IDE with Syntax Highlighting
Integrated Development Environments (IDEs) like IntelliJ IDEA, Eclipse, or NetBeans can help highlight syntax errors as you type. They provide real-time feedback, making it easier to catch errors early.
4. Break Down Your Code
If you encounter a syntax error that is difficult to locate, break your code into smaller, manageable parts. This strategy allows you to isolate sections and find the error more easily.
Best Practices to Avoid Syntax Errors
-
Consistent Code Formatting: Maintaining consistent formatting, including indentation and spacing, significantly reduces the chances of missing elements like semicolons or braces.
-
Engage in Code Reviews: Having others review your code can catch errors you might overlook. Collaborative code reviews promote best practices and improve code quality.
-
Write Modular Code: Breaking code into smaller classes or methods helps isolate functionality and makes it easier to locate syntax errors.
-
Familiarize with Java Syntax: Regularly revisiting Java's syntax rules ensures better retention and reduces the chances of basic mistakes.
Final Thoughts
In Java programming, syntax errors can be minor hiccups or major roadblocks. Understanding common errors and employing strategic fixes ensures smoother programming experiences. By following best practices and using tools to catch errors early, developers can significantly reduce syntactical pitfalls.
For further information on Java programming principles and best practices, consider checking out resources like Oracle’s Java Documentation or GeeksforGeeks Java Programming. With patience and persistence, mastering syntax becomes second nature and leads to more efficient and effective coding. Happy coding!
Additional Resources
By addressing and understanding common syntax errors in Java, you will become more proficient in your programming endeavors and ultimately enhance your software development skills.
Checkout our other articles