Avoiding Common Pitfalls in State Machine Development
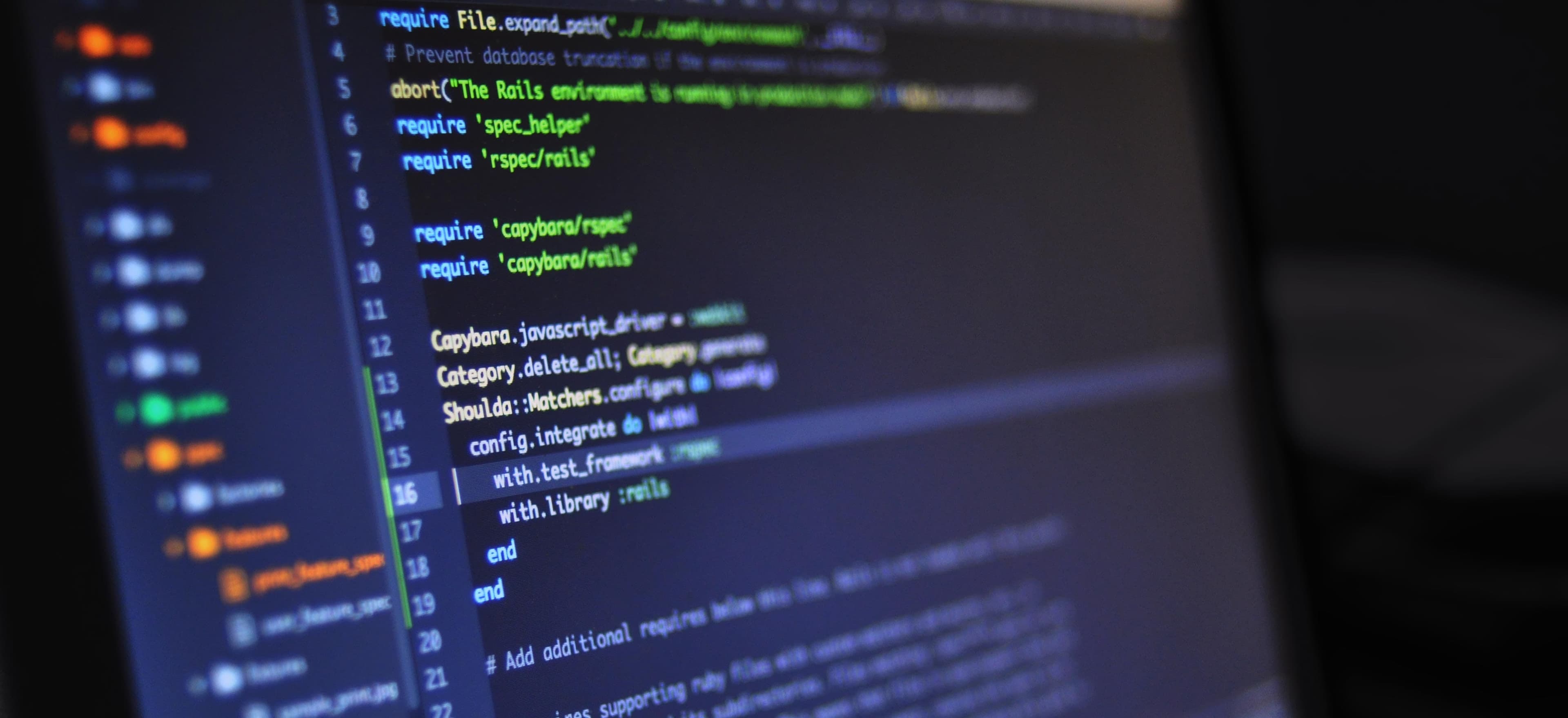
- Published on
Avoiding Common Pitfalls in State Machine Development
State machines are powerful tools used in software engineering that help manage the transitions between different states in a system. They are particularly useful in environments where the behavior of systems can be clearly defined according to their current states. However, developing state machines is not without its challenges. In this post, we will explore some common pitfalls in state machine development and discuss how to avoid them.
Understanding State Machines
Before diving into common pitfalls, let's clarify what a state machine is.
A Finite State Machine (FSM) is defined by:
- A finite number of states.
- A start state.
- A set of input events or triggers.
- A set of transitions between states based on events.
A simple Java implementation of a state machine might look like this:
public enum State {
IDLE,
RUNNING,
PAUSED,
COMPLETED
}
public class SimpleStateMachine {
private State currentState;
public SimpleStateMachine() {
this.currentState = State.IDLE; // Start state
}
public State getCurrentState() {
return currentState;
}
public void start() {
if (currentState == State.IDLE) {
currentState = State.RUNNING;
} else {
throw new IllegalStateException("Cannot start from " + currentState);
}
}
public void pause() {
if (currentState == State.RUNNING) {
currentState = State.PAUSED;
} else {
throw new IllegalStateException("Cannot pause from " + currentState);
}
}
public void resume() {
if (currentState == State.PAUSED) {
currentState = State.RUNNING;
} else {
throw new IllegalStateException("Cannot resume from " + currentState);
}
}
public void complete() {
if (currentState == State.RUNNING || currentState == State.PAUSED) {
currentState = State.COMPLETED;
} else {
throw new IllegalStateException("Cannot complete from " + currentState);
}
}
}
Why Use State Machines?
State machines offer several advantages:
- Clarity: They provide a clear picture of system behavior.
- Structured Control Flow: They can handle complex scenarios through simple state transitions.
- Manage Complexity: They break systems down into manageable pieces.
Now, let's explore some common pitfalls when developing state machines.
Common Pitfalls
1. Overcomplicating the State Machine
One of the most frequent mistakes is making the state machine more complex than necessary. For instance, adding states that don’t contribute meaningful transitions or actions can muddle the design.
Solution: Maintain simplicity. Focus on the core states and events that are essential to your system. Whenever introducing a new state, ask yourself: "Does this state serve a purpose?"
2. Ignoring State Transition Conditions
It's crucial to define the conditions required for transitions between states. Neglecting these conditions can lead to unexpected behaviors.
Consider the following modification of our SimpleStateMachine
:
public void start() {
if (canStart()) {
currentState = State.RUNNING;
} else {
throw new IllegalStateException("Cannot start from " + currentState);
}
}
private boolean canStart() {
return currentState == State.IDLE || currentState == State.PAUSED;
}
Why Define Conditions?
Defining conditions clarifies transitions and helps prevent system errors. Always write down the decision rules governing state changes.
3. Not Handling Unexpected States
State machines should gracefully handle unexpected inputs or states. If a state receives an unexpected event, it shouldn't crash or behave unpredictably.
Solution: Implement error handling for unforeseen states and events. Here's how you could enhance our state machine:
public void receiveEvent(Event event) {
switch (currentState) {
case IDLE:
if (event == Event.START) {
start();
} else {
logUnexpectedEvent(event);
}
break;
case RUNNING:
if (event == Event.PAUSE) {
pause();
} else if (event == Event.COMPLETE) {
complete();
} else {
logUnexpectedEvent(event);
}
break;
// Handle other states...
}
}
private void logUnexpectedEvent(Event event) {
System.err.println("Unexpected event: " + event + " in state: " + currentState);
}
Why Handle Unexpected States?
Anticipating unexpected inputs allows systems to remain robust. It also provides insights into areas that might require reconsideration.
4. Lack of Testing and Verification
Assuming the state machine logic is fool-proof without thorough testing is a common misstep. Bugs in state handling can lead to severe runtime issues.
Solution: Employ unit tests. Use libraries such as JUnit to create scenarios for each state and event interaction. Here’s a basic example:
@Test
public void testInitialState() {
SimpleStateMachine sm = new SimpleStateMachine();
assertEquals(State.IDLE, sm.getCurrentState());
}
@Test
public void testStartTransition() {
SimpleStateMachine sm = new SimpleStateMachine();
sm.start();
assertEquals(State.RUNNING, sm.getCurrentState());
}
Why Is Testing Critical?
Testing verifies that your state machine behaves as expected. It reduces risks of future changes breaking the existing logic.
5. Poor Documentation
Finally, neglecting to document your state machine can lead to misunderstanding and misuse. Proper documentation should clarify the purpose of each state, transitions, and events.
Solution: Write clear documentation alongside your state machine. Use diagrams if necessary. State machine diagrams can visually represent the flow and logic.
Why Document?
Documentation serves as a guide for future development and for other team members. It also aids in maintaining the codebase as the system evolves.
The Closing Argument
Developing a state machine can significantly simplify a program's control flow. However, avoiding common pitfalls is crucial to ensure its effectiveness and reliability. Always keep your design simple, define clear transitions, handle unexpected states, rigorously test your logic, and ensure proper documentation.
By adhering to these best practices, you can create a state machine that is robust, maintainable, and easy to understand. Happy coding!
For further reading, consider exploring articles on state machine design patterns or the use of state machines in game development.
Checkout our other articles