Overcoming Performance Bottlenecks in Apache Arrow Streaming
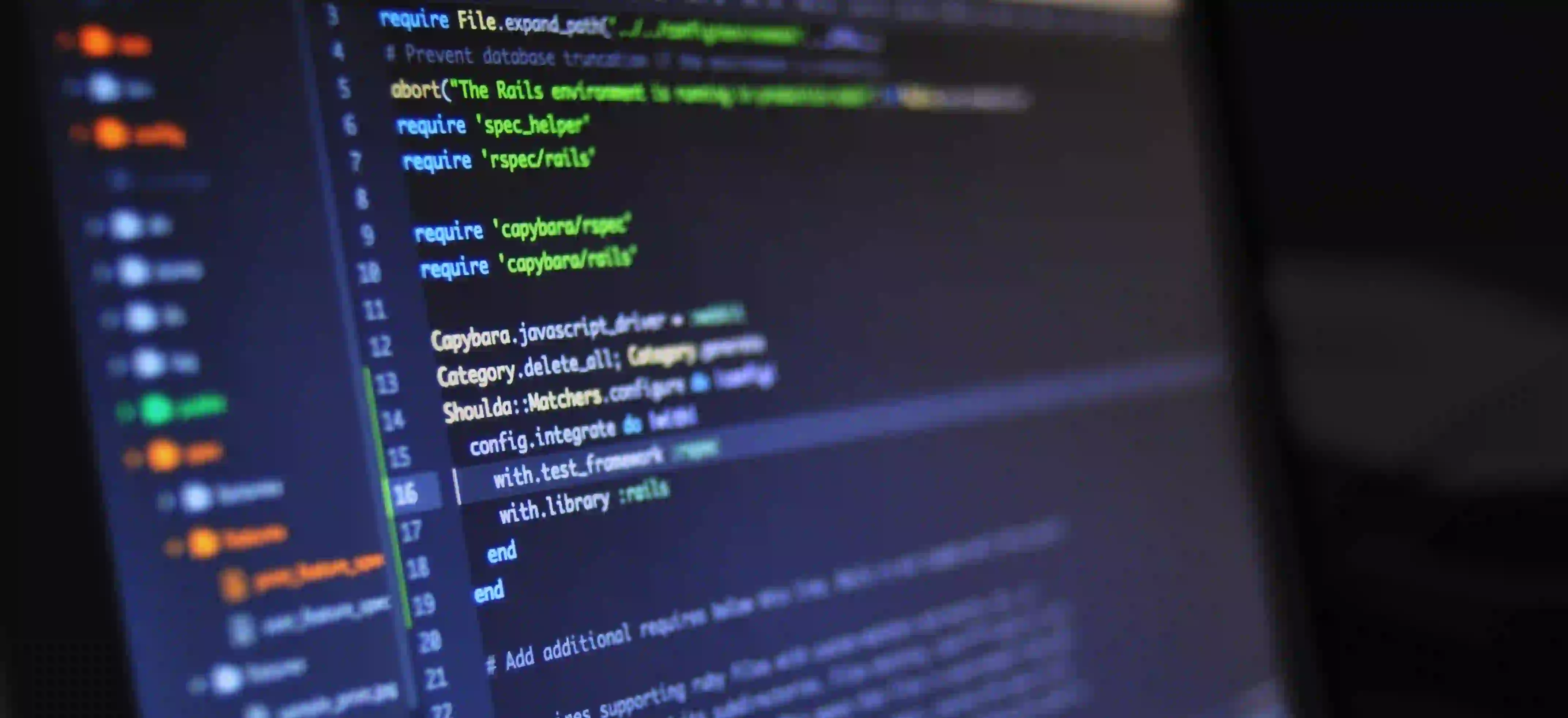
Overcoming Performance Bottlenecks in Apache Arrow Streaming
Apache Arrow has rapidly gained traction in the big data ecosystem due to its high-performance capabilities for in-memory analytics and efficient data interchange between processes. However, as with any technology, performance bottlenecks can arise in Arrow streaming applications. In this blog post, we will explore common challenges encountered when streaming data with Apache Arrow and provide solutions to overcome these bottlenecks.
Understanding Apache Arrow Streaming
Apache Arrow provides a columnar memory format which allows for seamless and fast data processing. The efficient serialization of data enables Arrow to support high-throughput data streaming. However, complications can occur when dealing with large datasets, network latency, or resource limitations.
Why use Apache Arrow for streaming?
- Speed: The columnar format is optimal for analytics tasks.
- Interoperability: Arrow allows different programming languages to share data interchangeably.
- Memory Efficiency: The format minimized overhead, allowing for better utilization of RAM.
Key Concepts to Understand
Before diving into performance tuning, it’s essential to understand some key concepts in Apache Arrow:
- Columnar Format: Data is organized into columns, which improves cache locality and minimizes the amount of data that must be read from memory at one time.
- Memory Mapping: Arrow employs zero-copy reads, which means data doesn’t need to be serialized and deserialized, improving throughput.
- Stream Table: A construct to manage the flow of data, allowing for efficient processing.
Identifying Performance Bottlenecks
Common Bottlenecks
- Network Latency: High latency can occur when streaming data between nodes in a distributed system.
- Serialization Overhead: Although Apache Arrow is designed to minimize serialization overhead, if large datasets require transformation, serialization can become a bottleneck.
- Concurrency Issues: Improper handling of multiple concurrent streams can lead to performance degradation.
- Memory Management: Inadequately managed or insufficient memory can create significant slowdowns.
Measuring Performance
Before tackling these issues, always quantify the performance bottleneck. Use profiling tools specific to the programming environment or libraries you are working with. Integrating Apache JMeter or Perf can allow you to visualize streaming performance.
Solutions to Overcome Bottlenecks
Optimize Data Serialization
Data serialization plays a critical role in stream performance. By utilizing Arrow’s native data types effectively, you can minimize conversion time. Here is an example of optimal serialization using both Arrow's RecordBatch and Stream structures:
import org.apache.arrow.memory.RootAllocator;
import org.apache.arrow.vector.IntVector;
import org.apache.arrow.vector.types.pojo.Schema;
import org.apache.arrow.vector.BatchSchema;
import org.apache.arrow.vector.VectorSchemaRoot;
...
try (RootAllocator allocator = new RootAllocator()) {
// Creating a schema for your data
Schema schema = new Schema(...); // Define your schema here
VectorSchemaRoot root = VectorSchemaRoot.create(schema, allocator);
IntVector intVector = root.getVector("yourIntVectorName");
// Bind your data
intVector.setSafe(0, 1);
intVector.setSafe(1, 2);
// Ensure memory managing is efficient
root.setRowCount(2);
// Process data further for streaming
}
Why this works: By sticking to Arrow's native structures and carefully managing the lifecycle of allocated memory, you mitigate serialization costs and optimize performance.
Utilize a Message Broker
In high-latency environments, employing a message broker like Apache Kafka can help decouple data producers and consumers. Arrow's capabilities can be directly integrated with Kafka Streams.
Adjust Network Settings
When working in a distributed environment, network settings can greatly impact performance. Here are some tips:
- Increase the TCP Window Size for larger throughput.
- Utilize efficient compression algorithms like Snappy or LZ4 during transmission.
- Ensure that streaming clients are configured with optimal buffer sizes.
Efficiently Handle Concurrency
Concurrency issues are common when managing multiple streams. To mitigate these challenges, consider using asynchronous programming techniques or worker pools to manage thread usage more effectively.
Some examples are:
import java.util.concurrent.*;
...
ExecutorService executor = Executors.newFixedThreadPool(10);
Callable<Void> task = () -> {
// Perform stream processing here
return null;
};
List<Future<Void>> futures = new ArrayList<>();
for (int i = 0; i < 10; i++) {
futures.add(executor.submit(task));
}
// Collect results if necessary
Why use ExecutorService: This abstraction helps in managing concurrent streams without exhausting system resources, improving throughput.
Monitor and Adjust Memory Allocations
Apache Arrow operates best when memory management is optimized. Properly allocating and deallocating memory resources can significantly enhance performance. Use Heap Memory Management judiciously, and analyze memory consumption over time with the following Java memory monitoring command:
jstat -gc <PID>
This command helps you monitor garbage collection in real-time and adjust your Arrow application resources accordingly.
Final Thoughts
Overcoming performance bottlenecks in Apache Arrow streaming requires a multi-faceted approach. With careful attention to serialization, network efficiency, concurrency, and memory management, you can significantly enhance the performance of your data streaming applications.
Remember that effective profiling and monitoring are essential components of this optimization process. Every application is unique, so tailor these strategies to fit your specific context.
For further information on Apache Arrow optimizations, consider checking out the Apache Arrow documentation.
By continuously questioning your performance metrics and refining your application’s architecture, you can unlock the full potential of Apache Arrow for real-time data processing and analytics.