Why Java Skill Assessments are Crucial Before Hiring
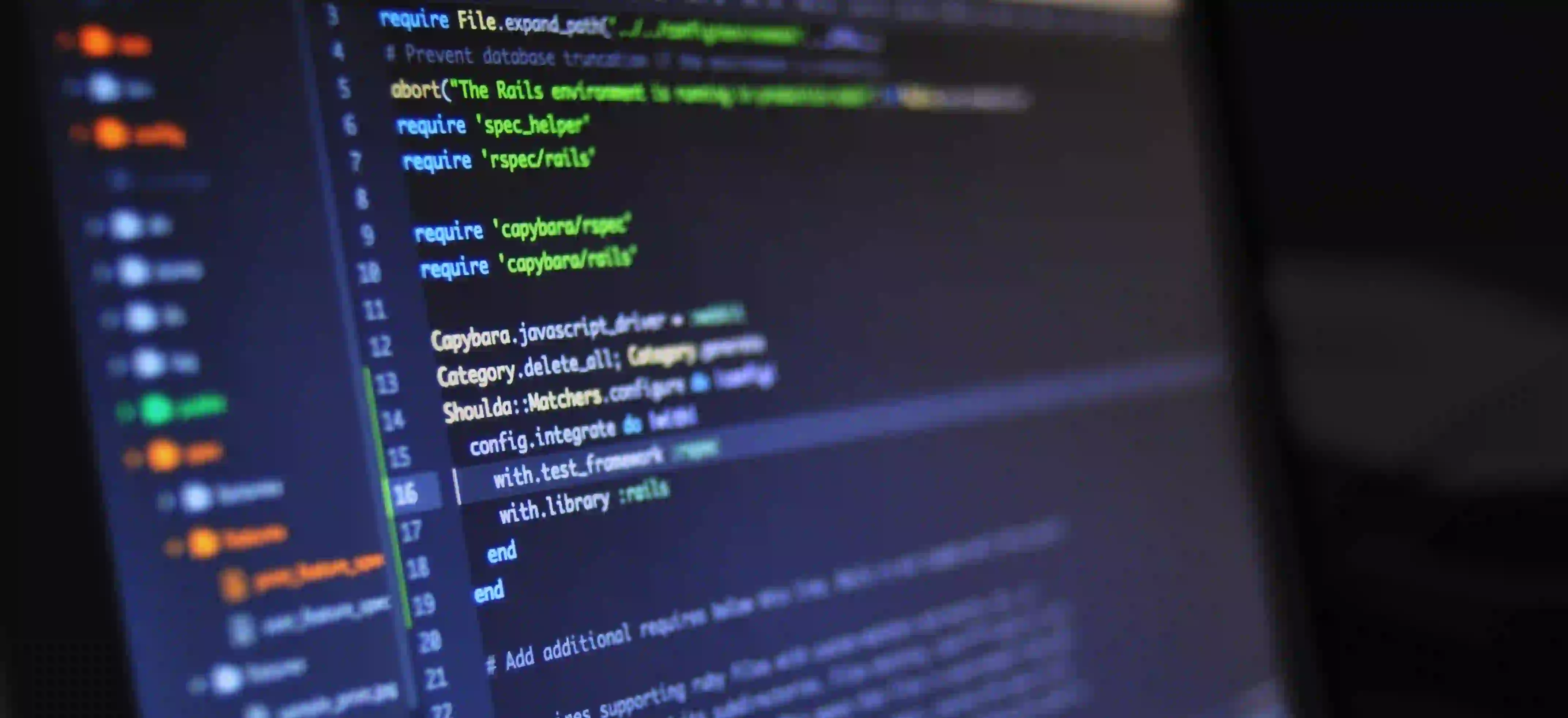
Why Java Skill Assessments are Crucial Before Hiring
In a rapidly evolving tech landscape, hiring the right talent can make or break a project. Java, a language that's been around for decades, continues to remain a cornerstone in software development. But how do you ensure that your next Java developer possesses not only the essential skills but also the adaptability to new technologies? This is where Java skill assessments come into play.
Importance of Java Skill Assessments
Before we delve into the specifics of conducting Java skill assessments, let’s outline why they are paramount in the hiring process.
-
Objective Evaluation: Skill assessments provide an objective measure of a candidate's capabilities. Instead of relying solely on resumes—often filled with buzzwords—skill tests offer a clear and quantifiable insight into what a candidate can actually do.
-
Understanding Project Needs: Every project has unique requirements. By assessing candidates based on those needs, you can better identify who can bridge the gap between theoretical knowledge and real-world problem-solving.
-
Reducing Turnover: A poor hiring choice based on subjective criteria can lead to high turnover rates. Assessments help in identifying candidates who are not just skilled but also a cultural and functional fit for your team.
-
Benchmarking Skill Levels: When conducting assessments across multiple candidates, it allows you to establish a benchmark for the skills you need. This ensures that your hiring practices remain consistent over time.
-
Encourages Continuous Learning: For candidates, going through a skill assessment is a learning opportunity in itself. It can highlight areas for improvement, pushing developers to advance their skills.
Key Areas to Assess in Java Skill Evaluations
When constructing a Java skill assessment, focus on the following areas:
-
Core Java Concepts: Understanding of fundamental concepts like OOP principles, inheritance, encapsulation, and polymorphism.
-
Framework Proficiency: Familiarity with popular frameworks such as Spring and Hibernate, enabling developers to build complex applications efficiently.
-
Problem-Solving Skills: The ability to approach and solve problems effectively. This could be assessed through coding challenges.
-
Data Structures and Algorithms: Proficiency in essential data structures (arrays, linked lists, trees) and algorithms (sorting, searching).
-
API and Libraries: Knowledge of Java APIs and built-in libraries is critical for building and maintaining applications.
Sample Java Skill Assessment
Below is a simple Java coding exercise you might use in an assessment, followed by commentary explaining the rationale behind it:
Task: Write a Java program to reverse a string.
public class StringReversal {
public static void main(String[] args) {
String input = "Java Developer";
String reversed = reverseString(input);
System.out.println("Reversed String: " + reversed);
}
public static String reverseString(String str) {
StringBuilder reversed = new StringBuilder();
// Loop through the string in reverse order
for (int i = str.length() - 1; i >= 0; i--) {
reversed.append(str.charAt(i));
}
return reversed.toString();
}
}
Commentary: The above code demonstrates the use of a class and method, as well as string manipulation techniques. This tests the candidate's understanding of essential components of Java programming.
-
StringBuilder: The use of
StringBuilder
is notable because it is mutable and thus more efficient for string concatenation compared to using the '+' operator in a loop. This indicates to the candidate that efficiency matters, even in seemingly simple tasks. -
Control Structures: This exercise also assesses the understanding of loops and conditions, which are fundamental in coding.
Advanced Assessment Techniques
While simple tasks can be effective, including more complex challenges will give a more rounded insight into a candidate's abilities. Here are some ideas:
-
Real-World Scenarios: Formulate problems based on challenges that your team currently faces. This will not only gauge problem-solving skills but also show how quickly a candidate can adapt.
-
Pair Programming: This technique allows you to see how candidates communicate and collaborate, which is as important as technical proficiency.
-
Code Review: Ask candidates to review a piece of code with deliberate flaws. This tests their attention to detail and knowledge of best practices.
Resources for Conducting Assessments
To conduct skill assessments effectively, consider utilizing online platforms designed for coding challenges. Tools like HackerRank and Codility enable you to create custom assessments tailored for Java developers. These platforms also provide analytics to gauge where candidates may excel or struggle, giving you valuable insights.
Lessons Learned
In today's competitive job market, having the right processes in place for assessing Java developers is essential. Skill assessments are an opportunity for both the company and the candidate to ensure a good fit that aligns with project objectives and team dynamics.
By implementing a robust assessment strategy, you not only improve your chances of hiring top-tier talent but also contribute to the professional growth of prospective candidates. Understanding Java, its frameworks, and the capability to solve problems can hugely impact long-term project success.
In summary, Java skill assessments are not just a checklist item; they are a crucial component of your hiring strategy. By committing to this practice, you are investing in a skilled team equipped to tackle the challenges of today's tech environment.
Further Readings
For additional information on best practices for technical assessments, check out Tech Recruitment: A Practical Guide. To dive deeper into Java frameworks like Spring and Hibernate, consider reading Spring Framework Documentation.
By maintaining a clear focus and structured approach, your skill assessments can establish a solid foundation for finding not just Java developers, but future tech leaders.