Overcoming Dagger 2 Setup Issues in GWT Applications
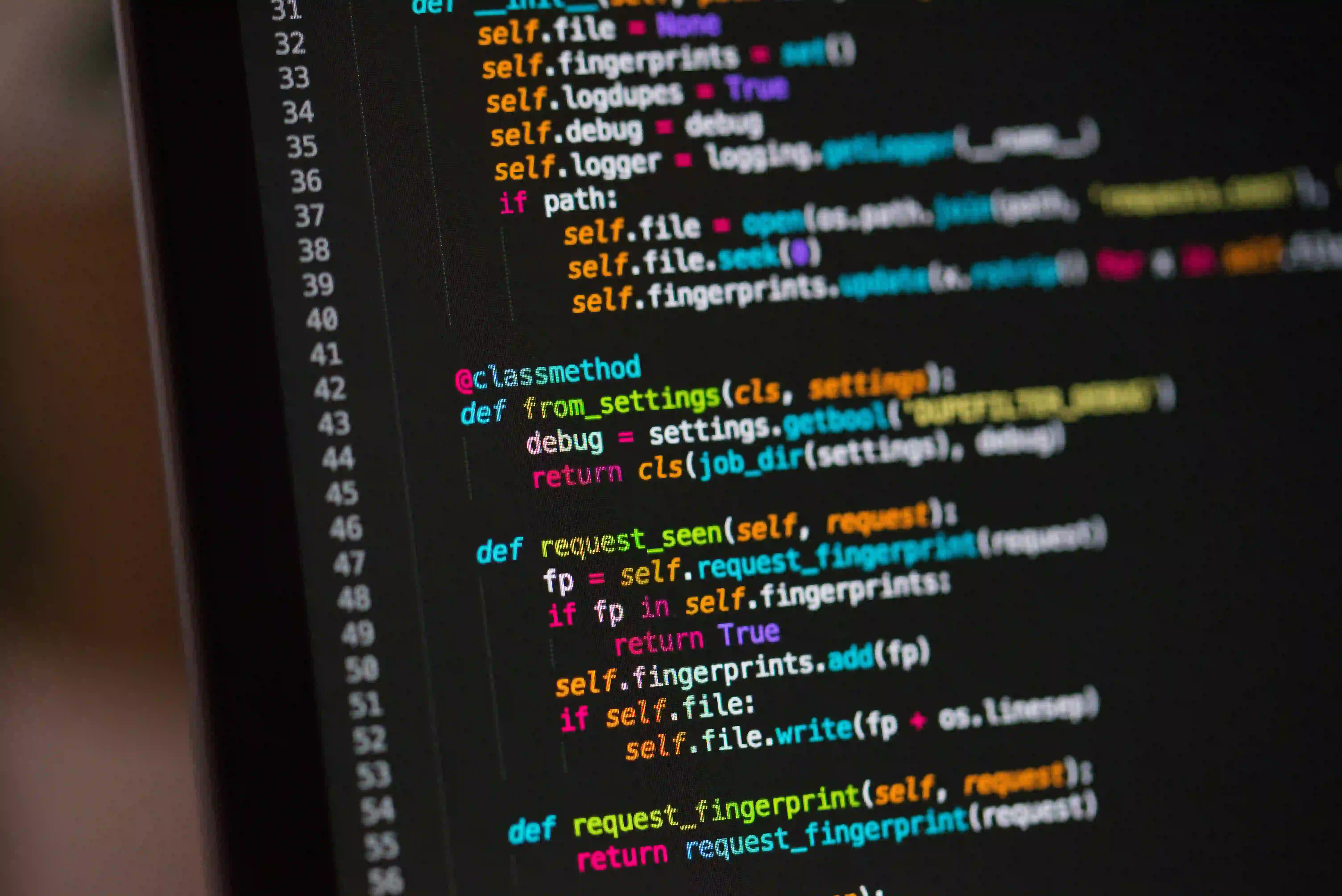
Overcoming Dagger 2 Setup Issues in GWT Applications
Google Web Toolkit (GWT) allows developers to write client-side code in Java and compile it into JavaScript. This powerful tool enables complex web applications to be built with strong type checks and rich, interactive features. However, integrating a dependency injection framework like Dagger 2 into a GWT application can present unique challenges. In this blog post, we will discuss common issues faced during the Dagger 2 setup process in GWT applications and provide practical solutions.
Why Use Dagger 2 with GWT?
Dagger 2 is a compile-time dependency injection framework for Java and Android that simplifies the process of managing dependencies in your applications. By using Dagger 2, you can:
- Improve Code Maintainability: Clear separation of concerns is achieved as Dagger manages object graphs and their lifetimes.
- Enhance Testability: Dependencies can be easily mocked, enabling unit testing without the need to instantiate actual classes.
- Reduce Boilerplate Code: Dagger minimizes the redundant code often required for instantiating dependencies.
With these advantages, you may wonder how to tackle the setup process efficiently in a GWT environment.
Common Issues When Setting Up Dagger 2 in GWT
1. Annotation Processing
Problem: Dagger 2 heavily relies on annotation processing to generate code at compile time. However, the GWT compilation process does not play nicely with annotation processors out of the box.
Solution: Ensure that annotation processing is properly configured in your build system. If you are using Maven, add the following plugin configuration to your pom.xml
:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
<annotationProcessorPaths>
<path>
<groupId>com.google.dagger</groupId>
<artifactId>dagger-compiler</artifactId>
<version>2.x</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
This configuration ensures that Dagger's annotation processor is invoked during the Maven build phase, allowing Dagger to generate the necessary code.
2. GWT's Restricted Class Loading
Problem: GWT uses a specialized class loading mechanism that can lead to ClassNotFoundException
errors when attempting to use Dagger.
Solution: Use @GwtCompatible
annotations to ensure that Dagger components and modules are compiled successfully. For instance:
import com.google.gwt.core.shared.GWT;
@GwtCompatible
public class MyModule extends AbstractModule {
@Override
protected void configure() {
bind(MyService.class).to(MyServiceImpl.class);
}
}
Enable GWT compatibility to ensure that your modules can be correctly recognized during the GWT compilation.
3. ProGuard Configuration
Problem: When using ProGuard for code optimization and obfuscation in release builds, Dagger-generated code may be inadvertently removed.
Solution: Update your ProGuard rules to retain Dagger-related classes. Insert the following lines in your proguard-project.txt
file:
-keep class dagger.** { *; }
-keep class ** implements dagger.Module { *; }
-keep class ** implements dagger.Component { *; }
This setup will ensure that ProGuard retains necessary Dagger classes during the optimization process.
Step-by-Step Guide to Setting Up Dagger 2 with GWT
Now that we have covered common issues, let's take a look at how to set up Dagger 2 in a GWT application step-by-step.
Step 1: Add Dagger 2 Dependencies
Add the Dagger 2 dependencies to your pom.xml
file.
<dependency>
<groupId>com.google.dagger</groupId>
<artifactId>dagger</artifactId>
<version>2.x</version>
</dependency>
<dependency>
<groupId>com.google.dagger</groupId>
<artifactId>dagger-compiler</artifactId>
<version>2.x</version>
<scope>provided</scope>
</dependency>
Step 2: Create Your Module
Create a Dagger module that provides the dependencies your application will use.
import dagger.Module;
import dagger.Provides;
@Module
public class AppModule {
@Provides
MyService provideMyService() {
return new MyServiceImpl();
}
}
Step 3: Create a Component
Define a component to connect your modules with the requesting classes.
import dagger.Component;
@Component(modules = { AppModule.class })
public interface AppComponent {
void inject(MyGwtEntryPoint entryPoint);
}
This component allows injection to happen in the specified classes.
Step 4: Setup Dependency Injection in GWT Entry Point
In your GWT entry point, perform the injection.
public class MyGwtEntryPoint implements EntryPoint {
@Inject
MyService myService;
public void onModuleLoad() {
DaggerAppComponent.create().inject(this);
myService.performAction();
}
}
This example shows how to inject MyService
into the entry point class. The DaggerAppComponent.create().inject(this);
line initializes Dagger's dependency graph.
Step 5: Compile and Test
Compile your GWT application. If everything is set up correctly, your GWT application should work seamlessly with Dagger 2, and you will be able to use your injected services.
Closing Remarks
Integrating Dagger 2 into your GWT applications can yield significant benefits in terms of maintainability, testability, and overall code quality. While the process may involve overcoming some common setup issues, such as annotation processing and ProGuard configuration, the rewards are definitely worth the effort.
For further reading on Dagger 2 and its features, check the Dagger 2 Documentation and the Google Web Toolkit Documentation. These resources can provide more insights and deeper understanding to enhance your applications effectively.
By following the steps outlined in this blog post, you should be well on your way to successfully integrating Dagger 2 into your GWT application. Happy coding!