Top 5 Missteps Java Developers Make in Career Growth
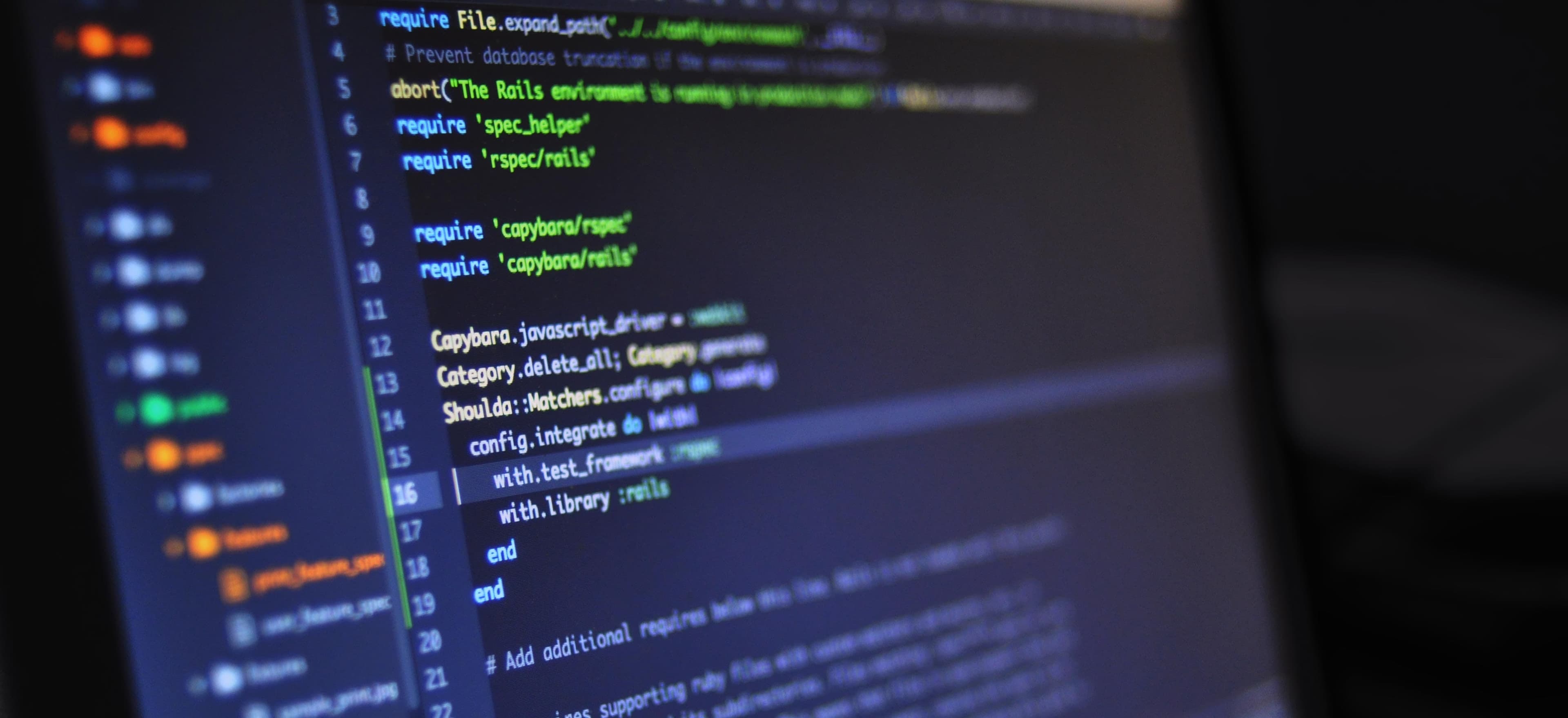
- Published on
Top 5 Missteps Java Developers Make in Career Growth
Java remains one of the most popular programming languages in the world, renowned for its versatility and robustness. However, many Java developers, especially early in their careers, often stumble upon certain missteps that can hinder their professional growth. In this post, we will explore the top five mistakes Java developers make and provide actionable insights on how to avoid them.
1. Neglecting the Fundamentals
Understanding the Basics
Many developers are eager to dive into frameworks and technologies like Spring or Hibernate, overlooking the core principles of Java. A solid understanding of the fundamentals — such as object-oriented programming (OOP), data structures, and algorithms — is crucial.
Why it Matters
- Problem-Solving: A strong grasp of Java fundamentals enhances your problem-solving skills.
- Framework Proficiency: Frameworks build on these principles; without foundational knowledge, you may struggle to use them effectively.
Actionable Tip
Spend time mastering OOP concepts such as inheritance, encapsulation, and polymorphism. Here are a few key points to consider:
// Example of OOP concepts in Java
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
void sound() {
System.out.println("Bark");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound(); // Output: Bark
}
}
In the example above, we see how inheritance allows Dog
to override the sound
method of its superclass, Animal
. Understanding this will make it easier when you leverage Java's rich libraries.
2. Failing to Stay Updated with Technology Trends
Keeping Up with the Change
Java is evolving, with newer versions and features released regularly. Developers often get comfortable with older versions, which can lead to skills stagnation.
Why it Matters
- Competitive Edge: Keeping up-to-date boosts your employability and helps you stand out in the job market.
- Enhanced Performance: New versions can provide performance improvements and cleaner syntax that older versions lack.
Actionable Tip
Regularly read blogs, join online forums, and participate in community discussions. Websites like Baeldung and Java Magazine offer valuable insights and updates.
You can also follow the release notes for Java SE to know what new features are available.
3. Ignoring Code Quality and Best Practices
Importance of Maintainable Code
Many developers prioritize functionality over code quality, resulting in messy codebases. Writing clean, maintainable code using best practices is essential for long-term project success.
Why it Matters
- Team Collaboration: Clean code is easier for your team to manage and build upon.
- Debugging: High-quality code saves time when debugging and testing.
Actionable Tip
Familiarize yourself with best practices such as:
- SOLID Principles: A set of design principles aimed at making code more understandable, flexible, and maintainable.
- Code Reviews: Engage in peer code reviews to get feedback and learn from others' coding styles.
Here’s a simple illustration of the Single Responsibility Principle (SRP):
// Poor design
class User {
void saveToDatabase() {
// Code to save user
}
void sendEmail() {
// Code to send email
}
}
// Improved design
class User {
// User properties and methods
}
class UserRepository {
void save(User user) {
// Code to save user
}
}
class EmailService {
void sendEmail(User user) {
// Code to send email
}
}
In the improved design, we separate concerns, allowing for better maintainability. Each class now has a single responsibility, adhering to the SRP principle.
4. Underestimating Soft Skills
Technical vs. Non-Technical Skills
Depending solely on technical prowess can be a mistake. Soft skills, such as communication, teamwork, and adaptability, are equally important in career growth.
Why it Matters
- Career Advancement: Technical skills might get you hired, but soft skills will get you promoted.
- Project Success: Good communication fosters better collaboration in project teams.
Actionable Tip
Engage in activities that improve your soft skills:
- Public Speaking: Join local Toastmasters or give presentations.
- Networking: Attend industry events and meetups to develop your interpersonal skills.
Online platforms like LinkedIn Learning offer courses to boost your soft skills significantly.
5. Shying Away from Collaboration and Community Engagement
The Power of Networking
Java development, like many tech fields, thrives on community. Some developers find it daunting to contribute to open-source projects or participate in discussions.
Why it Matters
- Learning Opportunities: Engaging with the community exposes you to different coding styles and solutions.
- Job Referrals: Networking can lead to hidden job opportunities and referrals.
Actionable Tip
Start small:
- Contribute to Open Source: Join projects on GitHub or other platforms.
- Join Online Communities: Websites like Stack Overflow and language-specific forums are excellent places to ask questions and learn from peers.
Here's a simple Git command to help you get started with a project:
git clone https://github.com/your-username/your-repo.git
This command clones a repository from GitHub to your local machine, enabling you to contribute easily. Make sure to familiarize yourself with the contribution guidelines of each project.
A Final Look
Neglecting the fundamentals, failing to update skills, ignoring code quality, undervaluing soft skills, and shying away from collaboration are the top mistakes many Java developers make. By being aware of these pitfalls and actively working to avoid them, you can significantly enhance your career trajectory.
Remember, continual learning and community engagement are keys to growth in the rapidly evolving Java landscape. Take proactive steps today, and watch your career flourish.
Additional Resources
For further learning, consider checking out:
- Effective Java by Joshua Bloch
- Java Concurrency in Practice by Brian Goetz
- Java Programming and Software Engineering Fundamentals on Coursera
Happy coding, and may your Java career be both fulfilling and successful!