Common Pitfalls When Deploying JAX-WS on Tomcat
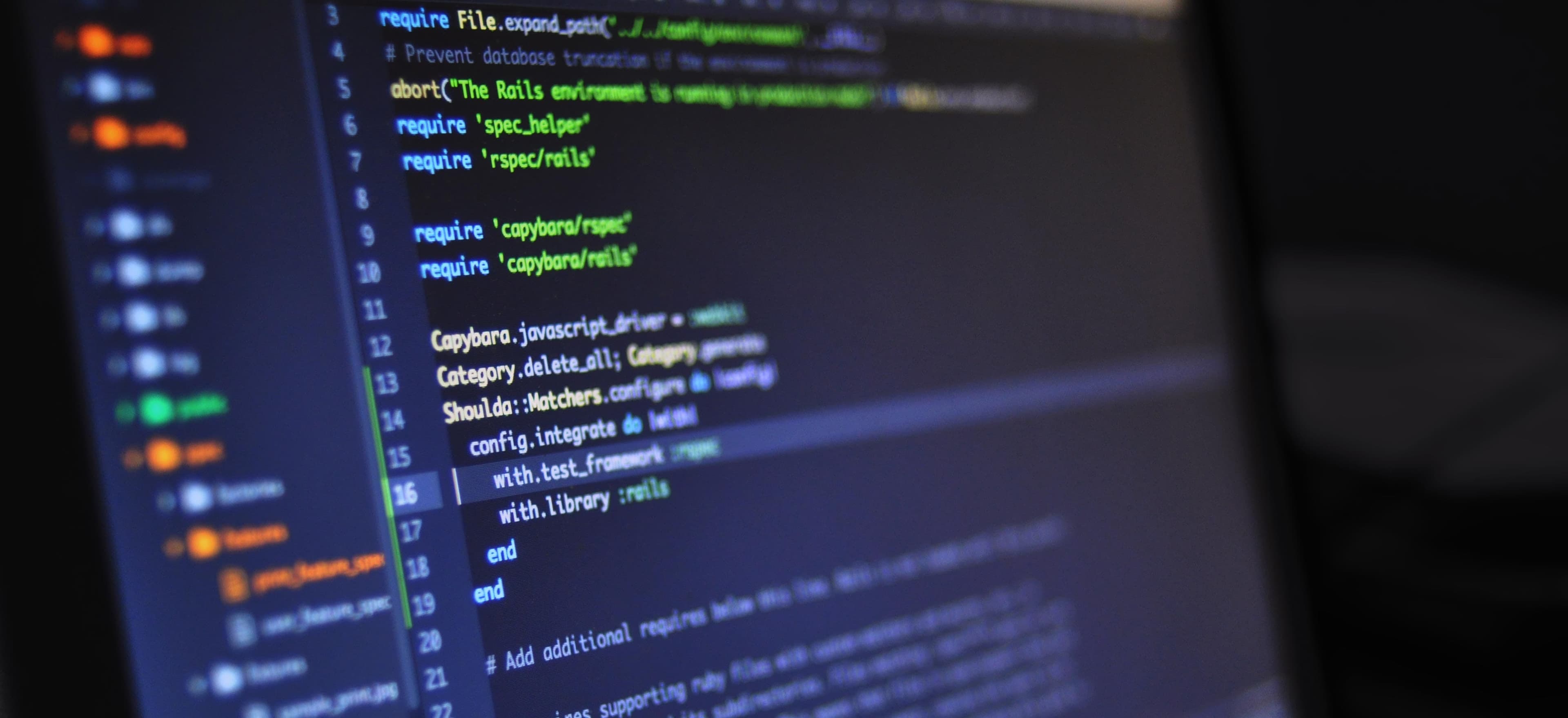
- Published on
Common Pitfalls When Deploying JAX-WS on Tomcat
Java API for XML Web Services (JAX-WS) is a powerful framework for creating web services in Java. Understanding and deploying a JAX-WS application on Apache Tomcat can greatly enhance your application’s capabilities. However, many developers stumble upon common pitfalls that can lead to frustrating debugging sessions and failed deployments. In this blog post, we'll outline these pitfalls and explain how to avoid them, providing you with sample code snippets for clarity.
What is JAX-WS?
JAX-WS is a Java standard that allows developers to create web services and consume web services using XML. It is built upon the SOAP (Simple Object Access Protocol), which allows for function calls over HTTP.
Tomcat is a popular open-source web server and servlet container that is widely used for running Java applications. When JAX-WS is deployed on Tomcat, developers unlock the potential of Java web services, but it is crucial to be aware of the common pitfalls associated with this process.
Common Pitfalls
1. Incorrect Configuration of Web Services
Problem:
A very frequent mistake involves incorrect configuration in the web.xml
file. This file is essential when deploying JAX-WS on Tomcat, as it defines servlets, filters, and other Java EE components.
Solution:
Ensure your web.xml
contains the proper configuration for the JAX-WS endpoint. Below is a sample configuration:
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<servlet>
<servlet-name>MyWebService</servlet-name>
<servlet-class>com.example.MyWebService</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>MyWebService</servlet-name>
<url-pattern>/MyWebService</url-pattern>
</servlet-mapping>
</web-app>
2. Missing Required Annotations
Problem:
Another common issue arises when developers overlook necessary JAX-WS annotations, such as @WebService
and @WebMethod
. These annotations are critical because they define the class as a web service and its methods as web service operations.
Solution:
An example of a properly annotated web service class is shown below:
import javax.jws.WebMethod;
import javax.jws.WebService;
@WebService
public class CalculatorService {
@WebMethod
public int add(int a, int b) {
return a + b;
}
}
Why This Matters: Without these annotations, your JAX-WS service will not be recognized or accessible, leading to a frustrating "service not found" error when you make requests.
3. Class Loading Issues
Problem:
Encoding issues, missing libraries, or incorrect classpaths can lead to class loading problems that prevent the JAX-WS service from running correctly.
Solution:
Ensure all required libraries such as jaxws-api.jar
, jaxws-impl.jar
, and any other dependent libraries are included in your WEB-INF/lib
directory. If you are using Maven, ensure your pom.xml
file includes the dependencies:
<dependency>
<groupId>com.sun.xml.ws</groupId>
<artifactId>jaxws-rt</artifactId>
<version>2.3.0</version>
</dependency>
4. Not Handling SOAP Faults Properly
Problem:
Inadequate handling of SOAP faults can lead to vague error messages for API consumers. This makes the application less user-friendly and more difficult to debug.
Solution:
Use @WebFault
to handle exceptions gracefully in your web service. Here’s how you can define a custom exception:
import javax.jws.WebService;
import javax.xml.ws.WebFault;
@WebService
public class CalculatorService {
@WebMethod
public int divide(int a, int b) throws MathException {
if (b == 0) {
throw new MathException("Division by zero", new Throwable());
}
return a / b;
}
}
@WebFault(name = "MathFault")
class MathException extends Exception {
private String faultInfo;
public MathException(String message, Throwable cause) {
super(message, cause);
this.faultInfo = message;
}
public String getFaultInfo() {
return faultInfo;
}
}
5. Apache Tomcat Version Compatibility
Problem:
Another pitfall is deploying on an unsupported version of Tomcat. Not all versions of Tomcat support JAX-WS out-of-the-box.
Solution:
Ensure that your version of Tomcat is compatible with JDK and JAX-WS. Versions greater than 7 should work fine, but testing compatibility is always recommended.
6. Firewall and Network Configuration Issues
Problem:
After deploying, you may be unable to access your web service due to firewall or network configuration issues.
Solution:
Check your server and local firewall settings and ensure that they allow traffic on the port you are using (default: 8080). Always validate if the endpoint is accessible by attempting to access it via your browser or a REST client like Postman.
7. Not Testing Your Service
Problem:
Neglecting to perform thorough testing can lead to failing services in production.
Solution:
Use tools like SOAP UI or Postman to test your JAX-WS services before deployment. Testing allows for identifying issues early, ensuring more stable software delivery.
8. Ignoring Maven Build Resources
Problem:
If you are using Maven for dependency management, failing to correctly configure the build resources can lead to runtime issues.
Solution:
Ensure that your Maven configuration file (pom.xml
) includes the necessary plugins to package your JAX-WS services along with dependencies:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>3.3.1</version>
<configuration>
<failOnMissingWebXml>false</failOnMissingWebXml>
</configuration>
</plugin>
</plugins>
</build>
Subscribe to updates at Maven's official site here to keep up with best practices.
Closing the Chapter
Deploying JAX-WS on Tomcat can provide immense benefits to your Java application, but understanding the common pitfalls is critical for a smooth deployment process. Incorrect configurations, missing annotations, handling SOAP faults, and class loading issues can derail your project if not adequately addressed. Always test your services and keep Tomcat version compatibility in mind.
By being proactive and checking these areas during the development lifecycle, you can take significant strides towards reliable and robust web services. The next time you prepare to deploy a JAX-WS application on Tomcat, you will be equipped with the right mindset to avoid these common pitfalls. Always remember that a thorough understanding of the underlying architecture is the first step towards effective deployment!
By applying these guidelines, your deployment process can transform from a trial-and-error maze into a streamlined, efficient operation. Happy coding!
For more advanced JAX-WS concepts, explore the Java JAX-WS documentation.