Understanding the Limitations of Java's Record Feature
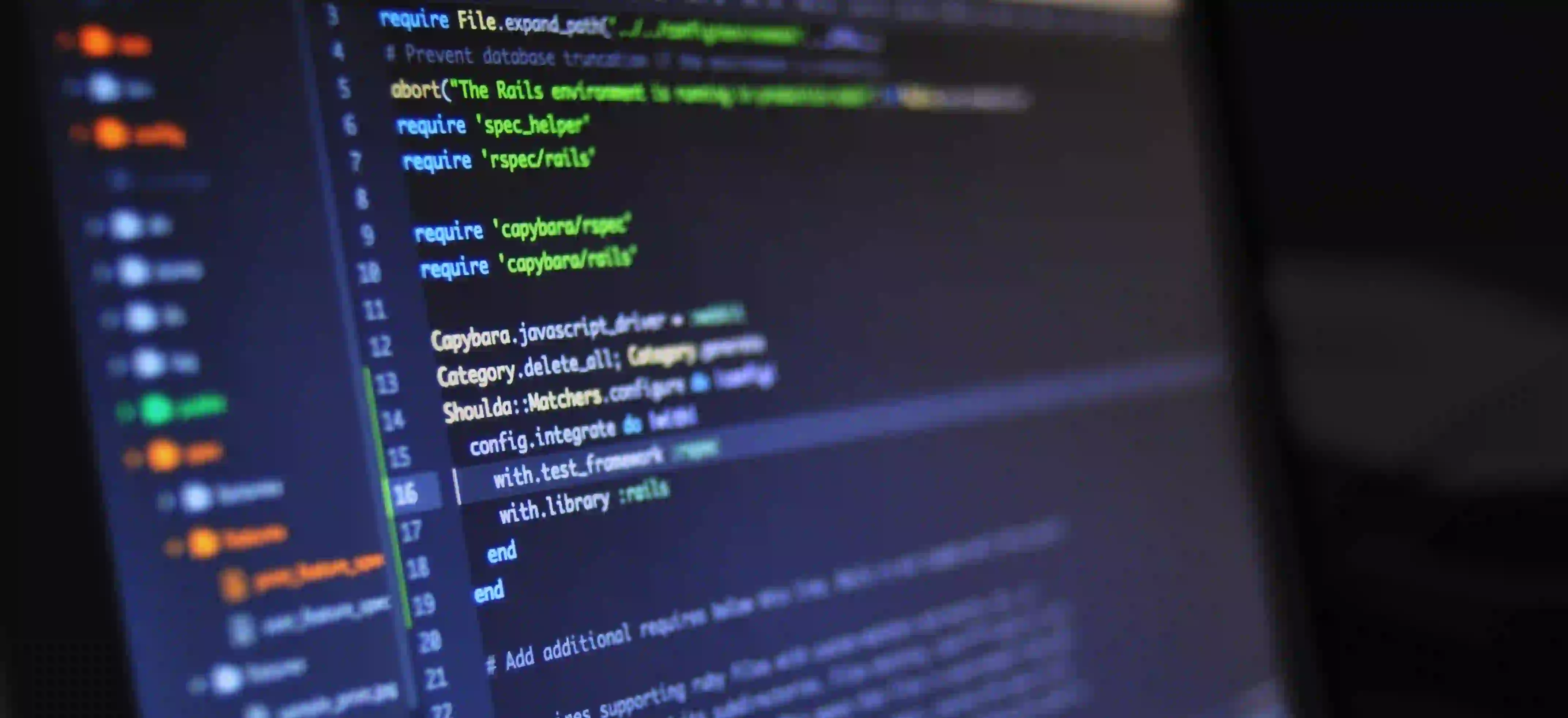
Understanding the Limitations of Java's Record Feature
Java has seen numerous enhancements since its inception, aimed at making the language more efficient and easier to use. One of the most significant additions in Java 14 was the introduction of records. Records provide a concise way of creating data carrier classes without the boilerplate code typical in traditional Java classes. However, despite their usefulness, records come with certain limitations that developers must understand.
In this blog post, we'll explore the characteristics of Java's record feature, its benefits, and its limitations. Together, we will pinpoint scenarios where records can be helpful and where their limitations could pose challenges.
What Are Java Records?
At its core, a record is a special kind of class in Java. Records provide a quick and efficient way to define classes primarily used to carry data. When you declare a record, the compiler automatically generates essential methods such as toString()
, equals()
, hashCode()
, and accessor methods for the fields, reducing boilerplate code significantly.
Syntax of Records
Here's an example of a simple Java record:
public record Person(String name, int age) {
}
In this example, the Person
record consists of two fields: name
and age
. The compiler generates the following methods automatically:
public String name()
public int age()
public String toString()
public boolean equals(Object obj)
public int hashCode()
This automatic generation allows developers to focus on business logic rather than boilerplate code, streamlining the development process.
Benefits of Using Records
1. Less Boilerplate Code
Developers often find themselves writing multiple lines of code just to create getter methods and other simple class structures. Records drastically reduce the boilerplate associated with data-carrying classes.
2. Immutability
Records are immutable by default. Once a record instance is created, its fields cannot be changed. This immutability leads to safer code, as the risk of unintended side effects is minimized, particularly in multi-threaded environments.
3. Easier to Read and Maintain
The concise syntax and built-in methods not only make records easy to write but also improve readability and maintenance. When you see a record, you can intuitively understand its purpose without delving into multiple methods.
Despite these benefits, there are important limitations to consider.
Limitations of Java Records
1. Records are Final
One significant limitation is that records are implicitly final. This means you cannot extend a record. While this limitation aligns with the principle of immutability, it can be a hindrance when you need to create subclasses to introduce variations of a data type.
Example:
public record Vehicle(String type, String brand) {
}
// This will cause a compilation error
public class Car extends Vehicle {
}
2. No Instance Fields
Records cannot have instance fields apart from the ones declared in their header. This means you cannot introduce any additional field to a record that is not part of its declared components. For instance:
public record Order(int orderId, String product) {
// This will cause a compilation error
private String status;
}
3. No Customizations of Method Implementations
While records automatically generate helpful methods like toString()
, equals()
, and hashCode()
, there are restrictions on customization. For example, if you try to override the toString()
method:
public record Point(int x, int y) {
@Override
public String toString() { // This will cause a compilation error
return "Point at (" + x + ", " + y + ")";
}
}
Only the implementation in the record declaration is allowed, limiting flexibility on how data representation is handled.
4. Limited Control Over Serialization
When it comes to serialization, records require specific conditions to be met. They cannot implement Serializable
directly due to the restrictions placed on serialization of default fields. While it is possible to customize serialization, the added complexity may lead to difficulties.
5. Disallowed Nullability for Components
While records handle typical Java objects well, they do not allow for nullability on their components. This can lead to NullPointerExceptions
if an instance is inadvertently created with null values.
Example:
public record User(String username, String email) {
public User {
if (username == null || email == null) {
throw new IllegalArgumentException("Fields cannot be null");
}
}
}
Scenarios Where Records Make Sense
Given their limitations, records shine in specific scenarios:
-
Data Transfer Objects (DTOs): For transferring data between layers in an application, records make an excellent choice.
-
Configuration Classes: Simple configurations or settings can be encapsulated within records without added complexity.
-
Immutable Data Structures: When building functional-style, immutable data structures, records allow easy instantiation while maintaining immutability.
Lessons Learned
Java's record feature is undoubtedly a powerful tool that simplifies the creation of data-carrying classes, minimizes boilerplate code, and promotes immutability. However, understanding its limitations is equally important. While records can enhance efficiency in many scenarios, they are not suitable for every use case.
As a developer, making informed decisions based on the specific requirements of your applications will ensure you leverage the strengths of both records and conventional classes effectively.
For further reading on Java records and their capabilities, consider exploring the Java documentation here or the Java Language Specification here.
By understanding both the features and limitations of records, you can improve your Java applications and maintain both code quality and performance effectively.