Mastering Stream-Powered Collections: Common Pitfalls to Avoid
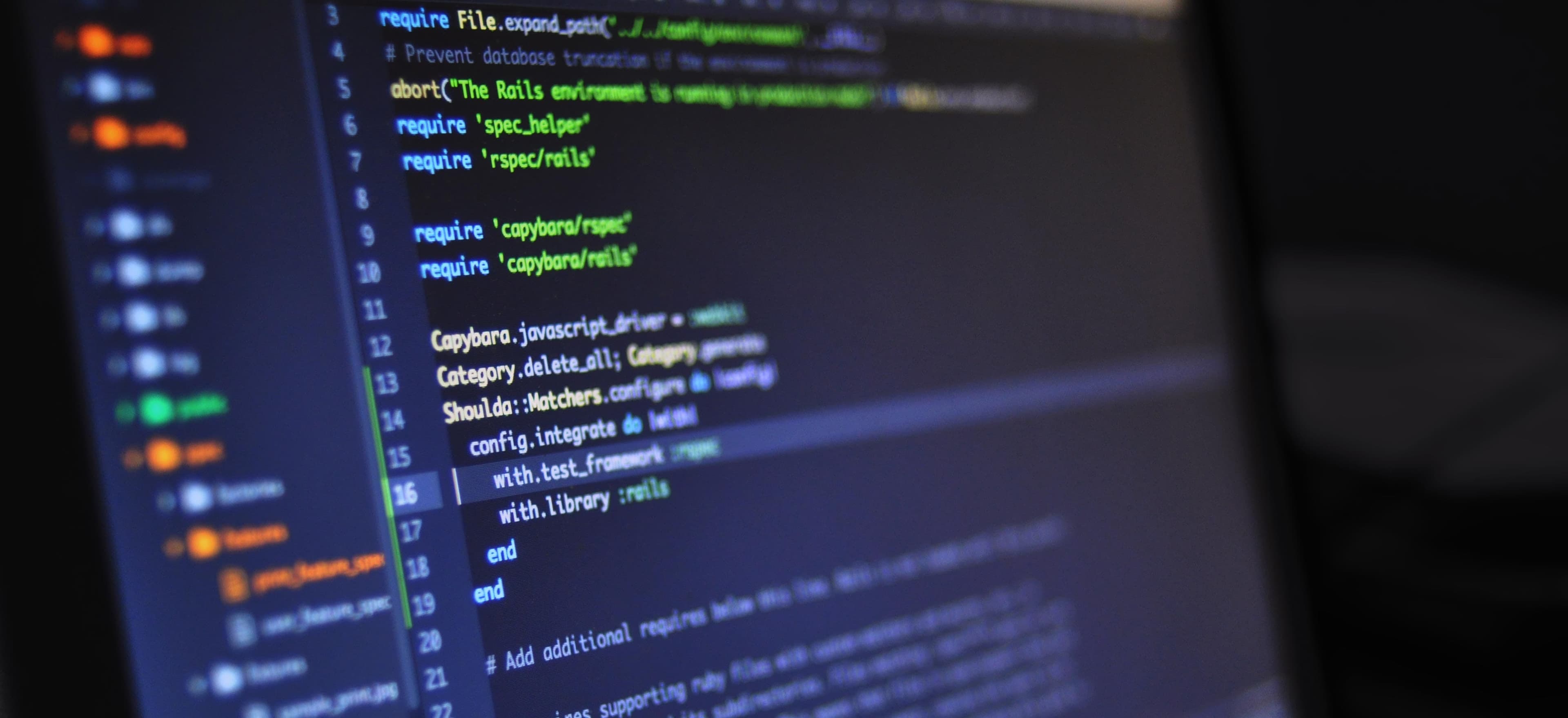
- Published on
Mastering Stream-Powered Collections in Java: Common Pitfalls to Avoid
Java Streams have revolutionized the way we interact with collections. Introduced in Java 8, the Stream API enables developers to process data in a functional style—cleaner and more succinct than traditional for-loops. However, as with any powerful tool, it’s crucial to understand potential pitfalls. In this blog post, we will explore common errors developers encounter when using Streams in Java, alongside best practices for mastering this powerful feature.
Table of Contents
Understanding Java Streams
Before diving into the pitfalls, let’s briefly recap what Java Streams are. A Stream
represents a sequence of elements supporting sequential and parallel aggregate operations. Unlike collections, Streams do not store data; they only provide a view of the data present in collections.
Example of Creating a Stream
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
Stream<String> nameStream = names.stream();
Here, we create a stream from a list of names. You can perform various operations on this stream, such as filtering, mapping, and reducing.
Common Pitfalls
While Streams offer a concise and expressive way to handle collections, several common pitfalls can adversely affect performance and readability.
1. Not Using Streams for the Right Use Cases
Streams shine in situations where you want to perform bulk operations and transformations. However, for simple tasks like iterating over a collection and performing actions, traditional loops can be more appropriate.
// Using a loop for simple iteration
for (String name : names) {
System.out.println(name);
}
While you could use a stream here, it might add unnecessary complexity. Streams should be used when your task involves transformations or data processing.
2. Failing to Limit Intermediate Operations
Intermediate operations like map()
, filter()
, or sorted()
are lazy; they do not execute until a terminal operation is invoked. However, applying too many intermediate operations can lead to poor performance.
List<String> result = names.stream()
.filter(name -> name.startsWith("A"))
.map(String::toUpperCase)
.distinct()
.sorted()
.collect(Collectors.toList());
In the example above, numerous operations are applied before the end collection occurs. Always try to minimize the number of intermediate operations and combine them whenever possible.
3. Forgetting to Collect Results
One of the frequent issues is forgetting to collect results after applying operations to a stream. Since streams are generally used in a pipeline fashion, a terminal operation is mandatory to produce a final result.
// Correct usage
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
In this example, we are filtering names and collecting them into a List
. Without the collect()
, no data will be returned, and you’ll just work with an empty stream.
4. Not Handling Exceptions Properly
Java Streams process elements in a functional style, which can make error handling tricky. It’s not advisable to throw checked exceptions from lambda expressions since it may lead to runtime exceptions.
// Wrong approach: Exception handling inside the stream
List<Integer> lengths = names.stream()
.map(name -> {
try {
return name.length(); // If there's a possible exception
} catch (Exception e) {
return 0; // Fallback value
}
})
.collect(Collectors.toList());
A better approach would be separating the logic that can throw exceptions and handle it beforehand.
5. Parallel Streams Pitfalls
When working with larger datasets, you may consider using parallel streams for performance. However, misuse can lead to unpredictable behavior and reduced performance.
// Using parallel stream
List<Integer> lengths = names.parallelStream()
.map(String::length)
.collect(Collectors.toList());
Parallel streams can improve performance but be cautious of mutable shared states, race conditions, and the cost of splitting tasks into parallel threads. Before choosing parallel processing, measure performance impacts and understand your data's nature.
Best Practices
To harness the full power of Java Streams while avoiding common pitfalls, keep these best practices in mind:
- Use Streams Judiciously: Apply streams when necessary, especially for bulk operations. Simple tasks may not need the overhead of stream processing.
- Minimize Intermediate Operations: Keep your operations concise and combine them when applicable.
- Collect Results Promptly: Always ensure that you use a terminal operation to gather results.
- Handle Exceptions Externally: Avoid handling exceptions within stream pipelines—do it outside the stream context.
- Understand When to Use Parallel Streams: Only use parallel streams when performance profiling indicates the benefits outweigh the complexity.
My Closing Thoughts on the Matter
Java Streams are a powerful feature that can enhance productivity and lead to cleaner code when used correctly. However, awareness of potential pitfalls is essential. By understanding these common errors and adhering to best practices, you can unlock the full potential of Stream-powered collections and elevate your Java programming skills.
For further context, consider checking Oracle's official Java documentation or refer to a comprehensive guide on functional programming in Java.
With this foundational knowledge, dive into your Java projects with confidence. Whether you're processing lists of items or transforming data streams, you now have the insights to avoid common traps and use Java Streams efficiently. Happy coding!