How to Retrieve Insert ID in JDBC with Ease
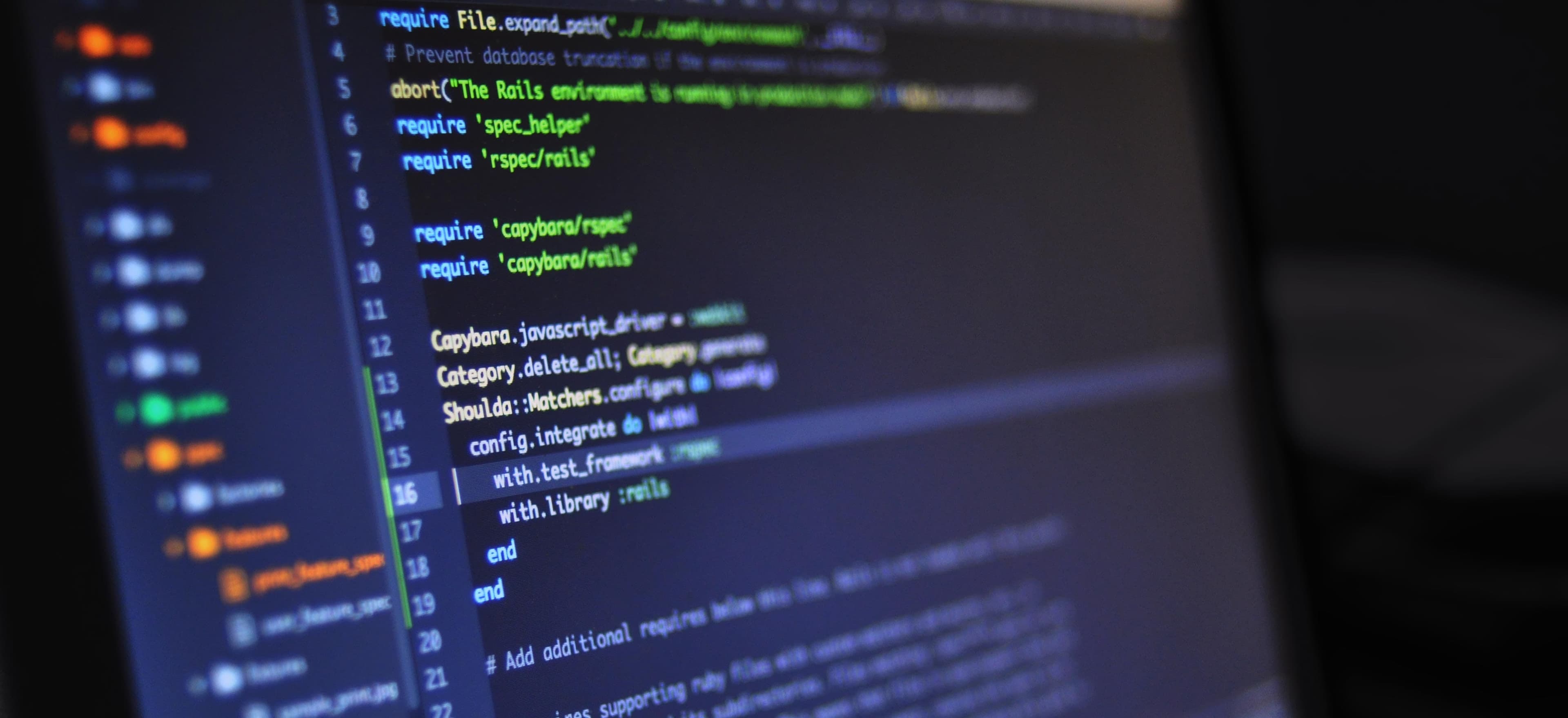
- Published on
How to Retrieve Insert ID in JDBC with Ease
Java Database Connectivity (JDBC) is a powerful technology in Java that enables you to connect to databases and execute SQL statements. One common requirement in database operations is to retrieve the ID of a newly inserted record. This is particularly useful in cases where IDs are auto-generated, such as with primary keys in SQL databases.
In this blog post, we will explore how to retrieve the insert ID in JDBC with ease. We will cover different approaches, provide code snippets, and discuss the underlying concepts so that you can implement this feature in your Java applications effectively.
Understanding the Basics of JDBC
JDBC is an API that allows Java applications to interact with relational databases. It offers a standard set of interfaces to provide a mechanism to connect to a database, execute SQL statements, and retrieve results.
Before we dive into retrieving the insert ID, let's quickly review the steps to set up a basic JDBC connection:
- Load the JDBC Driver: This step is necessary to register the driver with the DriverManager.
- Establish a Connection: Use the
DriverManager.getConnection()
method. - Create a Statement: This is used to send SQL statements to the database.
- Execute Queries: Utilize
Statement
orPreparedStatement
to execute your SQL commands. - Process Results: Handle the results returned from the database.
- Close Connections: Always close the resources to prevent memory leaks.
Retrieving Generated Keys
To retrieve the auto-generated keys from an insert operation, we will primarily use the PreparedStatement
interface in JDBC. The method getGeneratedKeys()
returns a ResultSet
object that contains the generated keys.
Setting Up the Database
For demonstration purposes, let’s assume you have a simple database table named users
:
CREATE TABLE users (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(100)
);
Code Example: Retrieving Insert ID
Here’s how to insert a new user and retrieve the auto-generated ID:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class JDBCInsertExample {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static void main(String[] args) {
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet generatedKeys = null;
try {
// Establish connection
connection = DriverManager.getConnection(URL, USER, PASSWORD);
// SQL insert statement
String sql = "INSERT INTO users (name) VALUES (?)";
preparedStatement = connection.prepareStatement(sql, PreparedStatement.RETURN_GENERATED_KEYS);
// Setting the parameter
preparedStatement.setString(1, "John Doe");
// Execute the insert statement
preparedStatement.executeUpdate();
// Retrieve generated keys
generatedKeys = preparedStatement.getGeneratedKeys();
if (generatedKeys.next()) {
long insertedId = generatedKeys.getLong(1);
System.out.println("Inserted User ID: " + insertedId);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// Close resources
try {
if (generatedKeys != null) generatedKeys.close();
if (preparedStatement != null) preparedStatement.close();
if (connection != null) connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
Explanation of the Code
- Connection Setup: We establish a connection to the database using
DriverManager.getConnection()
. - Preparing the Statement: The SQL INSERT statement is constructed, and we specified
PreparedStatement.RETURN_GENERATED_KEYS
to instruct JDBC to return the generated keys. - Setting the Parameter: We set the user's name before executing the statement.
- Executing the Statement: The
executeUpdate()
method is called to perform the insertion. - Retrieving the Generated Key: We call
getGeneratedKeys()
on thePreparedStatement
to retrieve the generated key(s) and check if the operation was successful.
By using the generated keys feature, we can easily access the ID of the newly inserted record.
Using Other Data Types for Generated Keys
In some cases, you might not be using a numeric type for your primary key. The getGeneratedKeys()
method will still work; you just need to make sure you retrieve the key using the corresponding data type. For example, if you're using a UUID as your primary key, you can retrieve it as follows:
UUID insertedId = (UUID) generatedKeys.getObject(1);
Handling Exceptions
Exception handling is crucial in JDBC operations. Always make sure to catch SQLException
and provide an informative message. It is also a good practice to log these exceptions using a logging framework for better debugging.
To Wrap Things Up
Retrieving the insert ID in JDBC is a straightforward process that can significantly enhance your database interaction in Java applications. By using the PreparedStatement
interface and invoking getGeneratedKeys()
, you can effortlessly obtain the autogenerated primary keys right after an insert operation.
For more in-depth knowledge about JDBC and its capabilities, consider checking out the official Java JDBC documentation or other useful resources like Baeldung’s guide on JDBC.
With the knowledge and examples provided in this post, you should now be well-equipped to implement ID retrieval in your own JDBC projects. Happy coding!
Checkout our other articles