Troubleshooting HTTP Status Code Issues with HttpClient 4
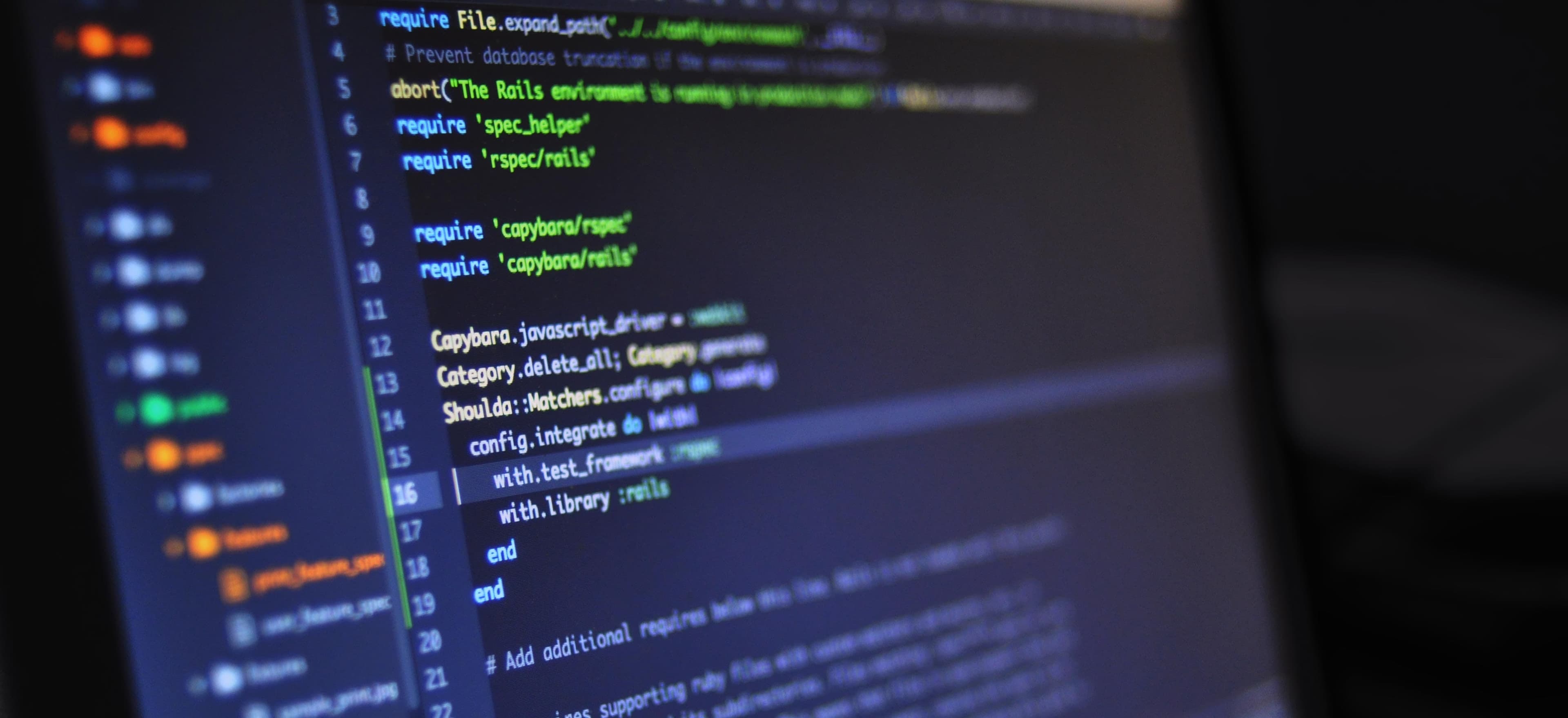
- Published on
Troubleshooting HTTP Status Code Issues with HttpClient 4
In the world of web development, HTTP status codes play a crucial role in client-server communication. As a Java developer, you may often find yourself working with HTTP requests and responses, relying heavily on the capabilities of libraries like Apache HttpClient 4. However, when things go awry, diagnosing the situation can be challenging. This blog post will guide you through the process of troubleshooting HTTP status code issues when using HttpClient 4.
Understanding HTTP Status Codes
HTTP status codes are issued by a server in response to a client's request. They indicate whether the request was completed successfully and provide information about the outcome. Below are some common HTTP status codes you might encounter:
- 200 OK: The request was successful.
- 404 Not Found: The requested resource was not found.
- 500 Internal Server Error: The server encountered an unexpected condition.
In practice, understanding these status codes is essential for effective debugging and resolving issues quickly.
Setting Up Apache HttpClient 4
Before diving deeper into troubleshooting, ensure that you have Apache HttpClient 4 set up in your Java project. You can include it as a dependency in your Maven pom.xml
:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version> <!-- You can check for the latest version -->
</dependency>
Making a Simple GET Request
Here’s a simple Java example demonstrating how to make a GET request using HttpClient 4:
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
public class HttpClientExample {
public static void main(String[] args) {
try (CloseableHttpClient client = HttpClients.createDefault()) {
// Create a GET request
HttpGet request = new HttpGet("https://httpbin.org/get");
// Execute the request
HttpResponse response = client.execute(request);
// Handle the response
System.out.println("Response Code: " + response.getStatusLine().getStatusCode());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Code Works
- HttpClients.createDefault() initializes the client.
- HttpGet is used to construct a GET request to a specified URL.
- client.execute() sends the request and captures the response.
Common HTTP Status Code Issues
1. Handling 404 Not Found
A 404 Not Found
status code typically indicates that the requested resource does not exist on the server. This could be due to an incorrect URL. Here's how to troubleshoot it:
- Check the URL: Confirm that the URL is correct and the server is running.
- API Documentation: If you are accessing an API, make sure to reference its documentation.
To demonstrate, you can adjust the previous GET request as follows:
HttpGet request = new HttpGet("https://httpbin.org/status/404");
// The above URL will intentionally trigger a 404 error
Quick Check
To verify the status code programmatically, use the following:
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == 404) {
System.out.println("Error 404: Resource not found!");
}
2. Handling 500 Internal Server Error
A 500 Internal Server Error
suggests something went wrong on the server-side. When you encounter this, it’s essential to approach it methodically.
- Server Logs: If you have access to server logs, check them for any error messages.
- Contact Support: If it’s an external API, reach out for details on downtime or issues.
Here’s how to capture this status code in the code:
if (statusCode == 500) {
System.out.println("Error 500: Internal server error!");
}
Implementing Retries for Resiliency
When dealing with HTTP responses, particularly with transient issues (like 500 errors), implementing a retry mechanism can be beneficial. Here’s an example of a basic retry mechanism:
public static void makeRequestWithRetry(String url, int maxRetries) {
int attempts = 0;
CloseableHttpClient client = HttpClients.createDefault();
while (attempts < maxRetries) {
try {
HttpGet request = new HttpGet(url);
HttpResponse response = client.execute(request);
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == 200) {
System.out.println("Success on attempt #" + (attempts + 1));
break;
} else {
attempts++;
System.out.println("Attempt #" + attempts + " failed with status: " + statusCode);
}
} catch (Exception e) {
attempts++;
System.out.println("Attempt #" + attempts + " failed with exception: " + e.getMessage());
}
}
}
Explanation of Retry Logic
- maxRetries controls how many attempts should be made.
- while loop continues until the request is successful or the maximum attempts are reached.
- Print messages provide real-time feedback about the attempt counts and status codes.
Logging HTTP Interactions
Logging is vital for diagnosing issues. Apache HttpClient provides a built-in logger which can be configured via Log4j or Java Util Logging. Here’s an example using Log4j:
log4j.properties
log4j.logger.org.apache.http=DEBUG
log4j.logger.org.apache.http.wire=DEBUG
By enabling logging, you'll gain deeper insights into the HTTP requests and responses, helping streamline your troubleshooting process.
The Bottom Line
Troubleshooting HTTP status code issues using HttpClient 4 can be simplified by understanding the status codes and implementing systematic approaches such as retries and logging. By adhering to best practices and referencing API documentation, you can significantly reduce debugging time and enhance application stability.
If you want to learn more about HTTP status codes, you can check MDN Web Docs on HTTP Response Status Codes. For more insights into using Apache HttpClient, consider visiting the official Apache HttpClient Documentation.
By applying the techniques mentioned in this blog post, you’ll be well-equipped to handle HTTP-related challenges in your Java applications effectively.