Mastering Stateful Testing with Hoverfly and Java
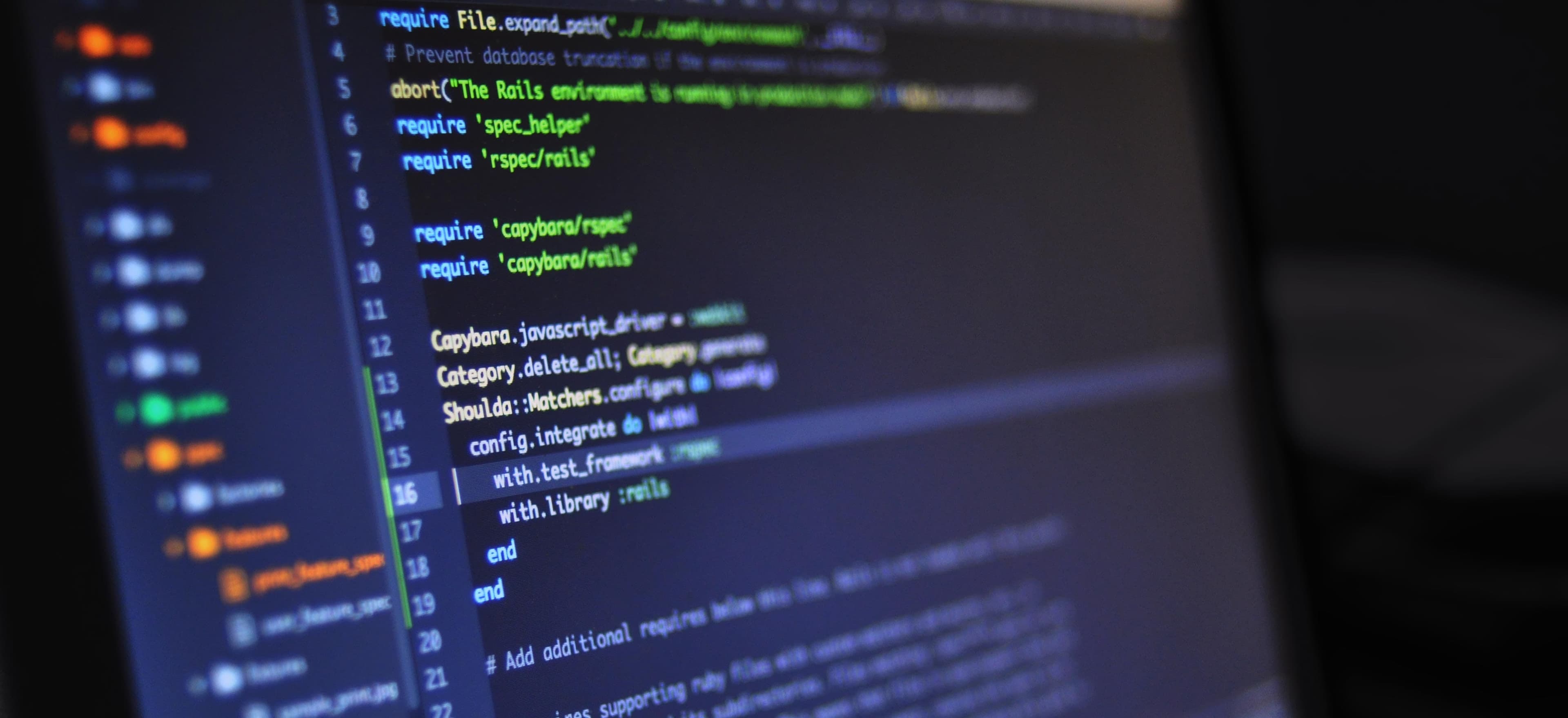
- Published on
Mastering Stateful Testing with Hoverfly and Java
Application testing is a critical aspect of the development lifecycle, especially as applications become increasingly complex. Among the various testing methodologies, stateful testing holds particular importance for applications that interact with external APIs or have complex data flows. In this blog post, we will delve into stateful testing using Hoverfly with Java, providing you with insights, code snippets, and best practices.
What is Stateful Testing?
Stateful testing involves testing an application’s behavior in response to different sequences of events or states. This approach is essential for applications that maintain state between requests, such as those that utilize a database or hold session data.
For instance, consider a shopping application where a user can add items to their cart, view the cart, and proceed to checkout. Each of these actions alters the application's state, and testing these transitions correctly is pivotal to ensure a reliable user experience.
Why Choose Hoverfly for Stateful Testing?
Hoverfly is an open-source API simulation tool designed to record, simulate, and analyze HTTP(S) traffic. It allows developers to easily mock external services and create automated stateful tests. Here are some reasons why Hoverfly is a good choice:
- Simplicity: Hoverfly works out-of-the-box and requires minimal configuration, allowing developers to focus on writing tests rather than setting up complex mock environments.
- Speed: By simulating only the necessary parts of the API, tests run faster, and you can be confident that your application logic processes correctly without relying on vulnerable external services.
- Flexibility: Hoverfly can be integrated with CI/CD pipelines, making it suitable for both development and production testing environments.
Getting Started with Hoverfly and Java
Before we dive into code, let’s set up Hoverfly in a Java project.
Step 1: Add Hoverfly Dependency
If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>io.specto.hoverfly</groupId>
<artifactId>hoverfly-java</artifactId>
<version>0.14.0</version>
<scope>test</scope>
</dependency>
This dependency allows us to use Hoverfly in our tests without affecting our production code.
Step 2: Creating a Stateful Test
Now let’s create a simple stateful test for a mock shopping cart API. For simplicity, we will mock the endpoints for adding items to the cart and checking out.
Sample Java Code:
import io.specto.hoverfly.junit.HoverflyRule;
import io.specto.hoverfly.junit.HoverflyMode;
import org.junit.Rule;
import org.junit.Test;
import static io.restassured.RestAssured.given;
import static org.hamcrest.Matchers.equalTo;
public class CartServiceTest {
@Rule
public HoverflyRule hoverflyRule = HoverflyRule.inCaptureOrSimulationMode("cart-service.json");
@Test
public void shouldAddItemToCart() {
// Simulate adding item to cart
given()
.contentType("application/json")
.body("{\"itemId\": 123, \"quantity\": 1}")
.when()
.post("http://localhost:8080/cart/add")
.then()
.statusCode(200)
.body("message", equalTo("Item added successfully"));
}
@Test
public void shouldCheckout() {
// Simulate checkout behavior
given()
.contentType("application/json")
.when()
.post("http://localhost:8080/cart/checkout")
.then()
.statusCode(200)
.body("message", equalTo("Checkout completed successfully"));
}
}
Explanation of the Code
-
Import Statements: The necessary Hoverfly and REST-assured libraries are imported to facilitate the mocking and testing process.
-
HoverflyRule: Using
HoverflyRule
, we set up our testing environment. TheinCaptureOrSimulationMode()
method indicates that Hoverfly should start capturing traffic or simulate responses based on the providedcart-service.json
file. -
Test Methods: Each test method uses
given()
,when()
, andthen()
constructs provided by REST-assured for writing fluent tests.-
The
shouldAddItemToCart()
test simulates adding an item to the cart. After sending the request, it verifies the status code and response message. -
The
shouldCheckout()
test validates the checkout process similarly.
-
Step 3: Creating Mock Responses
Capture the traffic when interacting with your actual API to create the cart-service.json
file, which contains the mock responses Hoverfly will use during testing.
To start capturing, run Hoverfly in capture mode. Here’s a command you can use:
hoverctl -web listen
During this time, interact with your API as you normally would. Once done, use the command below to export the captured data:
hoverctl export -f cart-service.json
Step 4: Running Your Tests
Once your testing code is set up and your responses are captured, you can run your JUnit tests as you would normally. Your tests will now execute against the mocked Hoverfly API, maintaining a stateful interaction for efficient testing.
Best Practices for Stateful Testing with Hoverfly
-
Isolate Tests: Ensure that your tests do not affect each other's state. Each test should ideally start with a clean state.
-
Use Verbose Logging: Enable logging in Hoverfly to understand better what requests were made and what simulated responses were returned.
-
Keep Tests Small: Each test should focus on a single functionality or state transition, making it easier to debug failures when they occur.
-
Regularly Update Captured Responses: As your application's API evolves, so should your mock responses. Regularly update your
cart-service.json
file to reflect any API changes. -
Combine with Other Testing Strategies: Stateful tests should complement unit and integration tests for a well-rounded testing strategy.
Lessons Learned
Stateful testing is vital to ensuring that your application functions correctly across various interaction patterns. By utilizing Hoverfly with Java, you can effectively simulate API responses, making your tests faster and more reliable.
As you implement these strategies in your projects, you'll find that handling state in your tests becomes manageable, which ultimately leads to a more robust application.
Explore more about Hoverfly in its official documentation and take your testing practices to the next level!
Happy testing!
Checkout our other articles