Common Pitfalls When Implementing Secure Encryption in Java
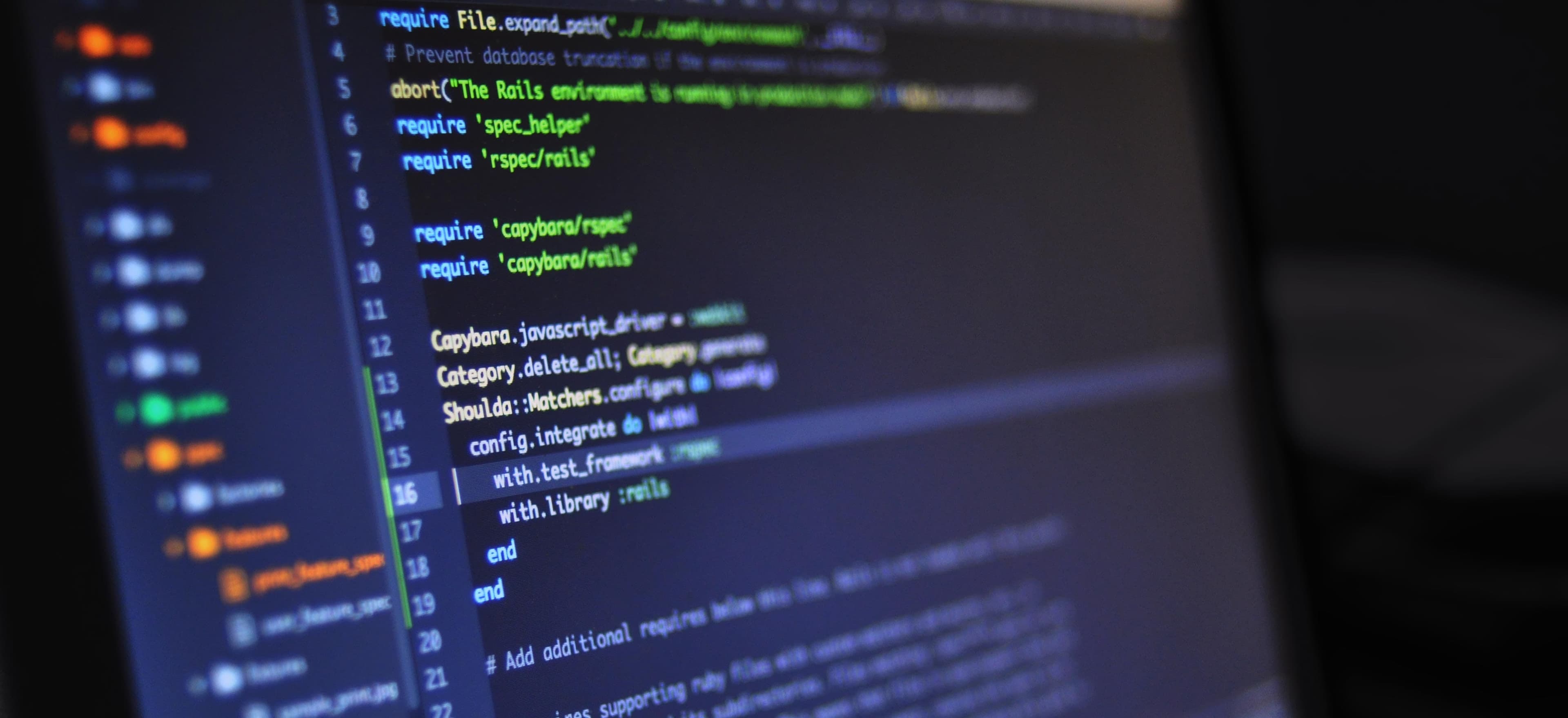
- Published on
Common Pitfalls When Implementing Secure Encryption in Java
In the digital era, ensuring data security is paramount, and encryption represents a fundamental tool in safeguarding sensitive information. However, while Java offers robust frameworks and libraries for encryption, developers can easily fall into common pitfalls that compromise security. This blog post aims to outline these pitfalls and provide best practices for implementing secure encryption in Java.
Understanding Encryption Fundamentals
Before diving into the pitfalls, let's briefly explore what encryption is. Encryption is the transformation of data to prevent unauthorized access. In Java, the Java Cryptography Architecture (JCA) allows developers to implement encryption and decryption mechanisms using various algorithms such as AES (Advanced Encryption Standard), RSA (Rivest–Shamir–Adleman), and more.
What Are Common Encryption Modes?
Encryption modes define how your encryption algorithms operate. Common modes include:
- ** ECB (Electronic Codebook)**: Simplest but not secure. Same plaintext will yield the same ciphertext.
- ** CBC (Cipher Block Chaining)**: More secure as it combines each block of plaintext with the previous ciphertext.
- ** GCM (Galois/Counter Mode)**: Authenticated encryption providing both confidentiality and integrity.
It's important to choose the right mode to ensure your data confidentiality and integrity.
Pitfall #1: Using Weak Algorithms
In Java, it’s tempting to use older or weaker algorithms such as DES (Data Encryption Standard) or RC4, thinking they’ll suffice. The reality is that these algorithms have known vulnerabilities.
Code Example
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class WeakAlgorithmExample {
public static void main(String[] args) throws Exception {
// Using DES - discouraged
KeyGenerator keyGenerator = KeyGenerator.getInstance("DES");
SecretKey secretKey = keyGenerator.generateKey();
Cipher cipher = Cipher.getInstance("DES/");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
// Encryption logic...
}
}
Why This Is Problematic: DES has a key size of only 56 bits, making it vulnerable to brute-force attacks. Using recognized strong algorithms like AES with a minimum key size of 128 bits is strongly encouraged.
Pitfall #2: Hardcoding Keys
Another frequent mistake is hardcoding encryption keys directly in the source code. This can lead to exposure if the code is ever decompiled or leaked.
Code Example
public class HardcodedKeyExample {
private static final String SECRET_KEY = "mysecretkey123"; // Weak key
public void encryptData(String data) {
// Encryption logic using hardcoded key...
}
}
Why This Is Problematic: Hardcoded keys are exposed to anyone who gains access to the code. Instead, consider using environment variables or secure vaults like HashiCorp Vault for storing secrets securely.
Pitfall #3: Ignoring Key Management
Even if keys are curated properly, poor key management practices can jeopardize security. Failure to rotate keys, store them securely, and implement access controls can lead to breaches.
Best Practices for Key Management
- Key Rotation: Regularly update keys to minimize risks if a key is compromised.
- Access Controls: Limit access to keys; only authorized applications should retrieve them.
- Secure Storage: Use secure storage solutions to manage and store cryptographic keys.
Pitfall #4: Not Using Salts and IVs Properly
Initialization vectors (IVs) and salts must be used correctly to ensure powerful security. A common mistake is using predictable IVs or static salts, which can expose your encryption to attacks.
Code Example
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.security.SecureRandom;
public class ProperIVExample {
public static void main(String[] args) throws Exception {
byte[] iv = new byte[16]; // Size should match block size
SecureRandom random = new SecureRandom();
random.nextBytes(iv);
SecretKeySpec keySpec = new SecretKeySpec("your-128-bit-key".getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, keySpec, new IvParameterSpec(iv));
// Encryption logic...
}
}
Why This Is Important: Using secure random values as IVs ensures that encrypting the same plaintext multiple times yields different ciphertext, thereby enhancing security.
Pitfall #5: Failing to Validate Input
Improper validation of input before encryption can lead to data leaks or corruption. Consider what happens if chucking unverified data into an encryption method.
Code Example
public class InputValidationExample {
public void encryptData(String data) {
// No validation - can lead to XSS, injection, etc.
// Encryption logic...
}
}
Why This Is Problematic: Failing to validate input allows attackers to manipulate the data flow and potentially carry out attacks such as injecting malicious content.
Pitfall #6: Ignoring Exceptions
Exception handling in encryption and decryption processes is critical. Ignoring exceptions could lead to security holes, making it easier for attackers to bypass security measures.
Code Example
try {
// Encryption logic...
} catch (Exception e) {
// Ignored - could compromise security
}
Why This Is Problematic: If exceptions are undocumented or hidden, it may lead to vulnerabilities being exploited without detection. Always log exceptions and handle them appropriately.
Summary
Implementing secure encryption in Java is not only a matter of using the right libraries but also understanding best practices in security. Ensuring that you do not succumb to common pitfalls—such as using weak algorithms, hardcoding keys, ignoring IVs and salts, failing to validate input, and improper exception handling—is vital for safeguarding sensitive data.
Further Reading
To bolster your encryption knowledge, you may find the Java Cryptography Documentation helpful. Additionally, for an understanding of common encryption patterns, explore more about the Secure Randomness in Java.
By being cautious and informed, you can implement encryption that protects your data effectively and efficiently in your Java applications.