Understanding Sealed Types: Common Pitfalls for Candidates
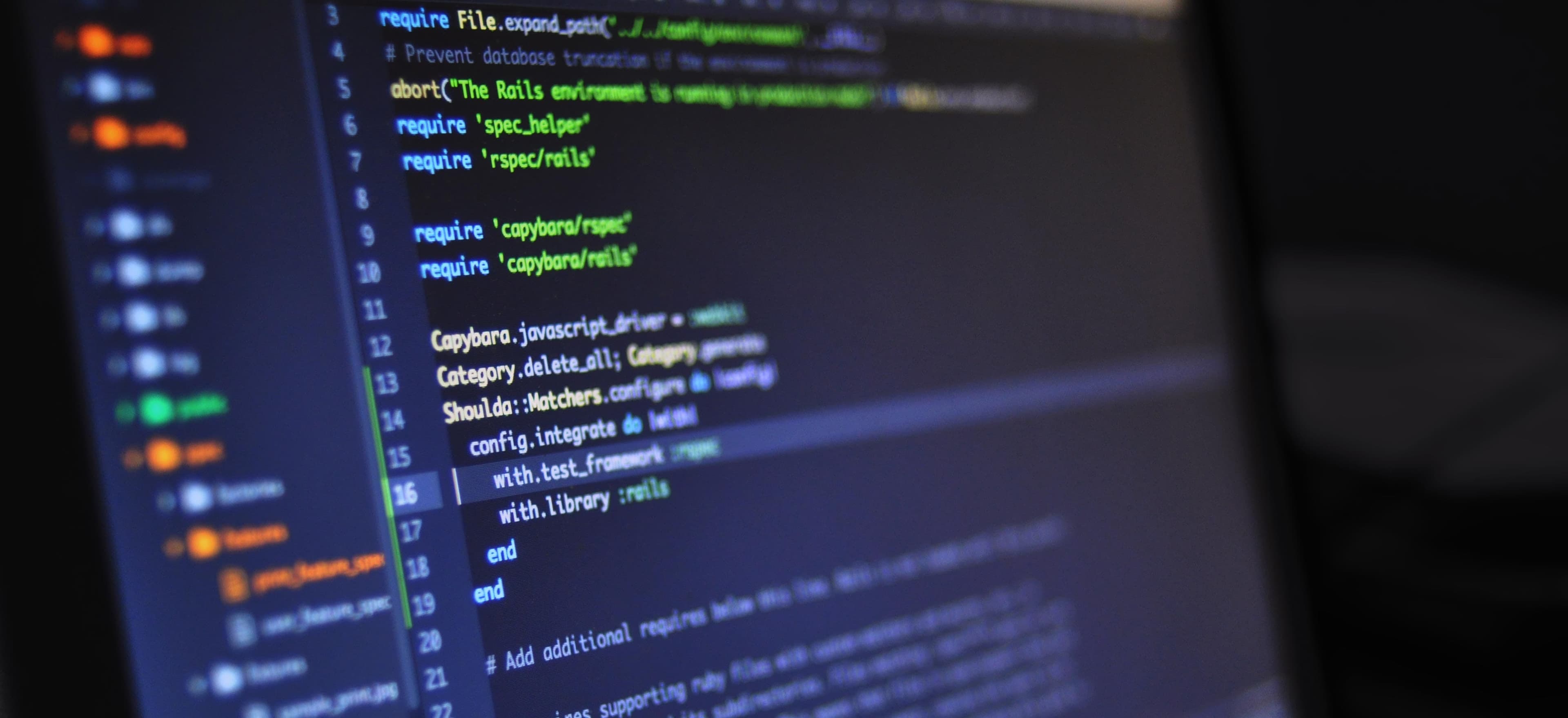
- Published on
Understanding Sealed Types: Common Pitfalls for Candidates
In recent years, Java has seen significant enhancements designed to make coding more efficient and expressive. One of the most notable additions is sealed types, introduced in Java 15 as a preview feature and fully incorporated in Java 17. Sealed types provide a way to restrict which classes can extend a particular class, granting developers more control and ensuring a more predictable and maintainable codebase.
During interviews or coding assessments, candidates often find themselves misinterpreting or mishandling sealed types. This blog post will delve into sealed types, highlighting some common pitfalls candidates encounter while also offering clear definitions, examples, and strategic measures to avoid these pitfalls.
What Are Sealed Types?
Sealed types allow you to control which classes can inherit from a given class or implement an interface. By doing so, you can define a more controlled environment for inheritance. This feature promotes a more robust design, allowing developers to define a limited scope of implementations.
Here's a simple example:
// Defining a sealed class
public sealed class Shape permits Circle, Rectangle {
}
// Allowed subclasses
public final class Circle extends Shape {
}
public final class Rectangle extends Shape {
}
Why Use Sealed Types?
- Controlled Inheritance: You define which classes can extend or implement your sealed classes or interfaces.
- Pattern Matching: Improved pattern matching capabilities in switch statements, which can leverage the closed set of subclasses.
- Explicit Design: A clearer design of your code, making it easier for others to understand how components interact.
Common Pitfalls
As we discuss sealed types, let's highlight some common pitfalls candidates face, elucidating why they occur and how to avoid them.
1. Forgetting the permits
Keyword
Pitfall: Candidates often define a sealed class but forget to include the permits
clause, leading to compilation errors.
Example:
// Incorrect: Missing permits clause
public sealed class Vehicle {
}
// Compilation error occurs here since no subclasses are permitted.
Solution: Always remember that a sealed class must declare which classes are allowed to extend it using the permits
keyword.
2. Using Abstract Subclasses Improperly
Pitfall: Some candidates attempt to define an abstract subclass for a sealed class. While this is possible, neglecting that the concrete classes must still terminate the subclass hierarchy can lead to confusion.
Example:
public sealed class Animal permits Dog, Cat {
}
// Attempting to create an abstract subclass
public abstract class Mammal extends Animal {
}
// Incorrect since Mammal is not in the permits clause
Solution: Ensure all subclasses derived from a sealed class are listed in the permits
clause to ensure proper hierarchy.
3. Misunderstanding Sealed Interfaces
Pitfall: Candidates might not realize that sealed interfaces operate similarly to sealed classes but are often less understood and frequently lead to confusion.
Example:
public sealed interface Vehicle permits Car, Truck { }
public final class Car implements Vehicle { }
public final class Truck implements Vehicle { }
// Incorrect: Attempt to extend sealed interface
public class Motorcycle implements Vehicle { } // Compilation error
Solution: Familiarize yourself with how sealed interfaces work and the permits
clause. Make sure you distinguish between class inheritance and interface implementation.
4. Ignoring Final Classes
Pitfall: A common misunderstanding is regarding the concept of final classes in relation to sealed types. Final classes cannot be extended, which can cause issues when integrating them into a sealed hierarchy.
Example:
public sealed class Shape permits Circle, Rectangle { }
public final class Circle extends Shape {
}
// Incorrect: Cannot create another subclass of Circle
public class SmallCircle extends Circle { } // Compilation error
Solution: Understand that using a final
class in your sealed hierarchy will restrict further inheritance, which is by design. Use this feature judiciously.
5. Overusing Sealed Types
Pitfall: Some candidates may overuse sealed types for every class or interface, leading to unnecessary complexity.
Example:
// Overused: A simple class now sealed
public sealed class Example permits AnotherExample {
// Not requiring sealed for this is more coherent
}
Solution: Use sealed types when it benefits your design clarity and maintainability. Otherwise, stick to standard inheritance.
Implementation Strategies
When implementing sealed types in your Java programs, consider these strategies:
-
Plan Your Hierarchy: Before coding, plan out which classes should be sealed and use the
permits
clause to clarify your design. -
Evaluate Necessity: Ask yourself if using a sealed class is necessary. Utilize sealed types selectively, aiming for clarity.
-
Test Extensively: When designing with sealed types, write unit tests to ensure all permitted subclasses function as intended, especially in pattern matching scenarios.
-
Document Your Design: Provide clear documentation for your sealed classes and interfaces, explaining the purpose of sealing and the rationale behind chosen subclasses.
To Wrap Things Up
Sealed types present an excellent opportunity to create more controlled, reliable, and maintainable Java code. However, it's essential to understand their nuances and properly implement them to avoid common pitfalls. By keeping in mind the mentioned pitfalls and solutions, you’ll not only enhance your understanding of sealed types but also your coding proficiency in Java.
For further exploration, consider reading the official Java Documentation on Sealed Types and the Java Language Specification for deeper insights.
Keep honing your skills, and remember to embrace the power of sealed types for a more robust Java programming experience!