Overcoming Common Pitfalls in Lightweight Database Microservices
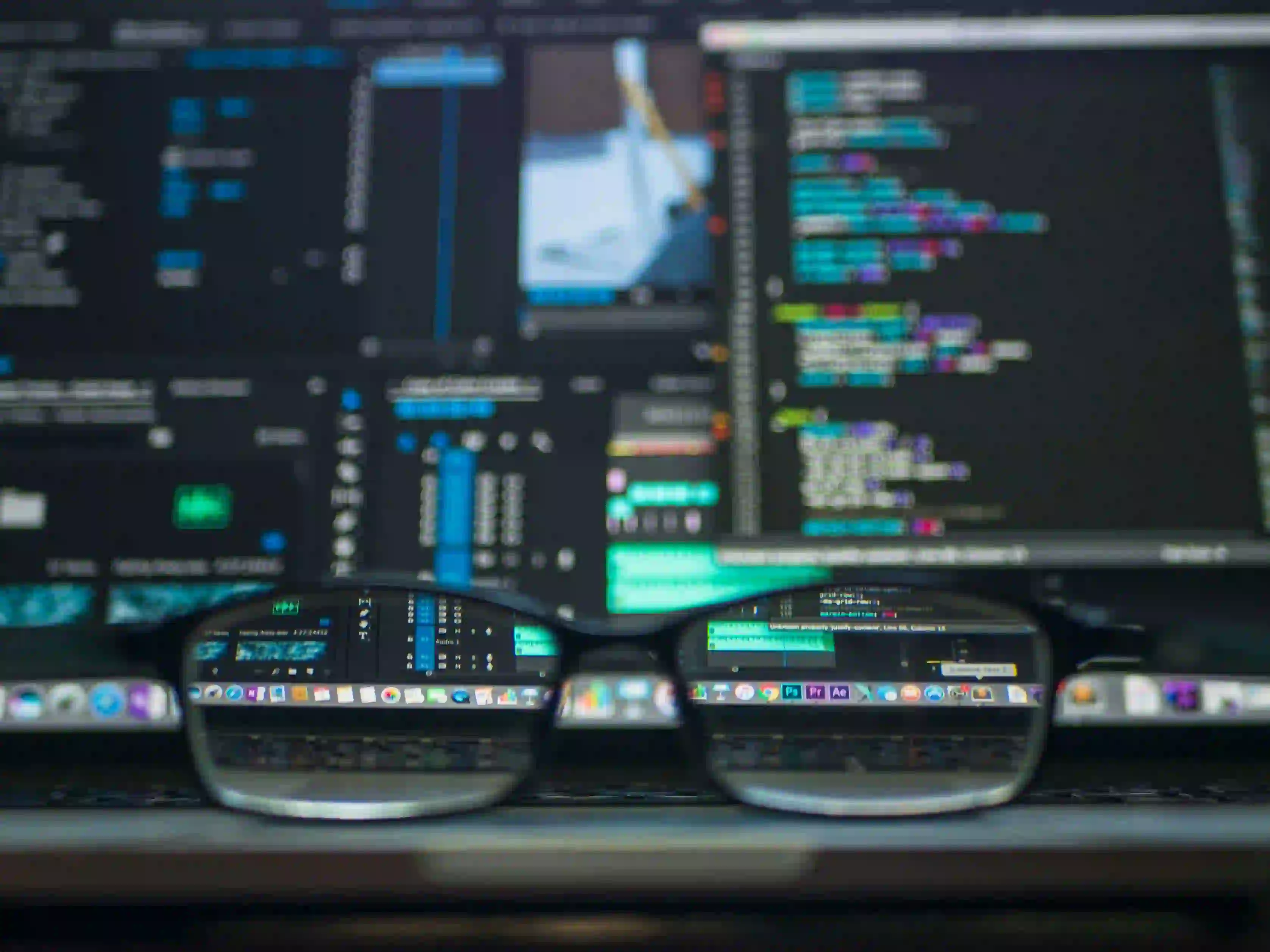
Overcoming Common Pitfalls in Lightweight Database Microservices
The rise of microservices architecture has revolutionized the way we build and deploy applications. One of the critical aspects of this architecture is the interaction with databases. While lightweight databases can enhance performance and scalability, they come with their own set of challenges. In this blog post, we will explore these common pitfalls and provide actionable strategies to overcome them.
Understanding Lightweight Databases
Before diving into the pitfalls, it is essential to clarify what we mean by lightweight databases. These databases typically have smaller footprints, lower resource needs, and can be easier to manage. Some popular lightweight databases include:
- SQLite: An embedded database known for its simplicity.
- H2: A Java SQL database that can run in memory.
- PostgreSQL: Often considered lightweight when configured properly because of its robust features and performance.
Benefits and Challenges
The primary benefits of lightweight databases are their speed, ease of integration, and reduced deployment time. However, they can also lead to challenges such as:
- Limited scalability
- Potential data inconsistency
- Complicated query handling
Common Pitfalls
1. Overestimation of Scalability
One of the most common misconceptions is that lightweight databases are inherently scalable. While they may perform well under low loads, they often struggle under significant demands.
Solution: Benchmarking
Before opting for a lightweight database, conduct thorough benchmarking under expected load conditions. Tools like Apache JMeter can simulate loads and provide insights into how the database performs.
Example Code Snippet:
import org.apache.jmeter.MetronomeJMeter; // Assuming a JMeter setup
public class DatabaseBenchmark {
public static void main(String[] args) {
MetronomeJMeter jmeter = new MetronomeJMeter();
jmeter.startLoadTest("SQLite_Load_Test.jmx");
}
}
// This code is a placeholder for initializing a load test using Apache JMeter.
By measuring the performance, you can determine if the database can handle your specific requirements.
2. Neglecting Data Consistency
Lightweight databases, particularly those designed for local use, may not handle concurrent writes effectively, leading to potential data inconsistencies.
Solution: Implementing Saga Patterns
Using a saga pattern can help manage distributed transactions effectively. This pattern divides a transaction into smaller, manageable steps, ensuring data consistency throughout.
Example Code Snippet:
public class PaymentSaga {
public void startSaga() {
try {
initiatePayment();
updateInventory();
sendConfirmation();
} catch (Exception e) {
compensate(); // Rollback mechanism if any step fails
}
}
private void initiatePayment() {
// Logic for payment initiation
}
private void updateInventory() {
// Logic for inventory update
}
private void sendConfirmation() {
// Logic for sending confirmation
}
private void compensate() {
// Logic to revert changes
}
}
// This code illustrates a basic saga pattern for ensuring data consistency during a transaction.
For more about sagas and their implementation, you can check out this article.
3. Ignoring Database Design
Poor database schema design can hinder performance and complicate queries. Lightweight databases often provide less functionality for schema modification, making it crucial to invest time upfront in the design process.
Solution: Database Normalization
Normalization helps in reducing redundancy and improving data integrity. You can use tools like ER diagrams to visualize the schema before implementation.
Example Code Snippet:
CREATE TABLE Users (
UserID SERIAL PRIMARY KEY,
UserName VARCHAR(50) NOT NULL,
Email VARCHAR(50) UNIQUE NOT NULL
);
CREATE TABLE Orders (
OrderID SERIAL PRIMARY KEY,
OrderDate TIMESTAMP NOT NULL,
UserID INTEGER REFERENCES Users(UserID)
);
// This SQL code defines a basic normalized structure for Users and Orders tables, ensuring clear relationships.
Investing time in refining your database schema saves significant headaches later on.
4. Lack of Monitoring and Metrics
Failing to monitor performance metrics can leave teams unaware of bottlenecks and critical issues until they escalate.
Solution: Implementing Monitoring Tools
Utilizing monitoring tools like Prometheus or Grafana can help capture important metrics such as query times, CPU usage, and memory consumption.
Example Code Snippet:
# Example Prometheus configuration for monitoring a lightweight database
scrape_configs:
- job_name: 'lightweight_db'
static_configs:
- targets: ['localhost:5432'] # Adjust for your database port
Monitoring provides insights into performance, allowing for proactive optimization.
5. Over-Reliance on ORM Libraries
Object-Relational Mapping (ORM) libraries simplify database interactions but can also abstract away important performance issues. Over-reliance can lead to suboptimal queries.
Solution: Custom Queries
While ORMs are beneficial, always be prepared to write custom SQL queries when necessary. Profiling your queries can provide insights into their efficiency.
Example Code Snippet:
public List<User> fetchActiveUsers() {
String sql = "SELECT * FROM Users WHERE isActive = true";
return jdbcTemplate.query(sql, new UserRowMapper());
}
// Here, custom query using JdbcTemplate ensures that the database interaction is optimized for fetching active users.
Implementing custom queries allows for greater control over performance.
The Closing Argument
Building and managing lightweight database microservices is replete with challenges. However, by anticipating pitfalls and employing best practices, you can create a robust and scalable architecture.
From understanding the limitations of lightweight databases to actively monitoring performance, the strategies outlined in this post aim to guide your development process.
To deepen your understanding, explore Microservices Architecture further for principles and patterns that can improve your approach.
In the end, the key takeaway is simplicity in design paired with vigilance in monitoring. Happy coding!