Mastering Java's New Local Variable Type Inference Challenges
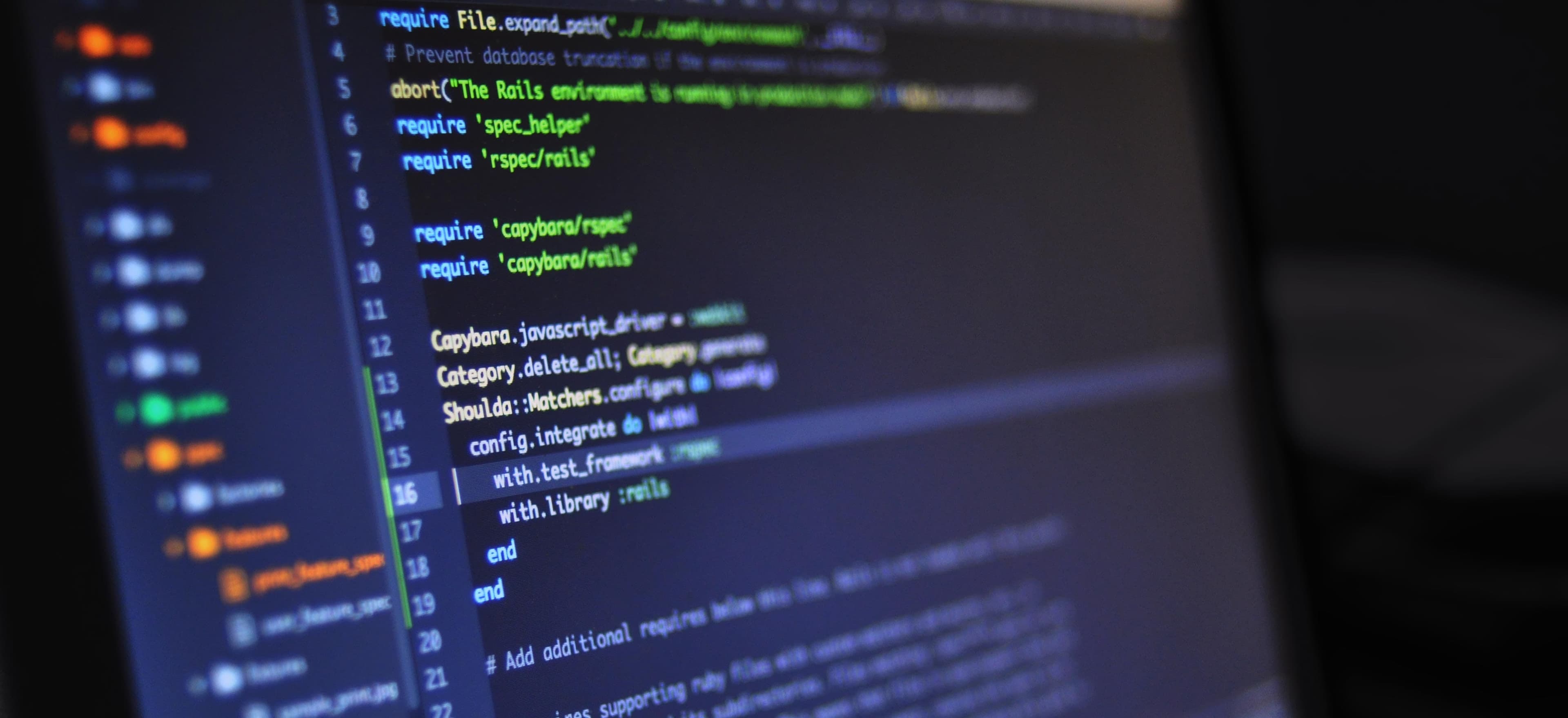
- Published on
Mastering Java's New Local Variable Type Inference Challenges
Java has evolved significantly over the years, and with the introduction of Java 10, local variable type inference (also known as var keyword) made its grand debut. This feature highlights Java's adaptability while also raising questions about readability and type safety. In this blog post, we'll explore what local variable type inference is, its benefits and challenges, and share practical examples to illustrate its usage.
What is Local Variable Type Inference?
Local variable type inference allows developers to declare local variables without explicitly specifying their type. Instead of writing the type, you can use the var
keyword. For instance, instead of:
String greeting = "Hello, World!";
You can simply write:
var greeting = "Hello, World!";
Java infers the type of the variable based on the assigned value. Thus, the inferred type for greeting
remains String
.
Benefits of Using Local Variable Type Inference
- Conciseness: By reducing boilerplate code, it can make your code less verbose and easier to read.
- Readability: In cases where the type is obvious from the context,
var
can enhance readability. - Flexibility: For complex generic types,
var
can simplify declarations, improving clarity.
For example, consider this complex generic type:
Map<String, List<Integer>> map = new HashMap<>();
Using type inference, it becomes:
var map = new HashMap<String, List<Integer>>();
Challenges with Local Variable Type Inference
While using var
has its advantages, it also comes with challenges that developers need to be cautious about.
1. Reduced Readability
While var
can make code concise, it risks obscuring the meaning of the code in scenarios where the type isn't immediately clear. Consider:
var stream = new ArrayList<>().stream();
What type does stream
refer to? At a glance, it is not obvious.
2. Loss of Type Safety
In some cases, using var
can lead to type-related mistakes that would be caught at compile-time if explicit types were used. For instance:
var number = 10; // inferred as int
number = "text"; // Should raise a compile error!
The ambiguity brought in by type inference can lead to logical errors that compromise the integrity of your code.
3. Misuse in API Consistency
Using var
with method return types can be misleading when the end user of the API cannot directly see the type being returned. This could lead to confusion when consorting with external libraries.
Practical Examples and Commentary
To better understand the challenges and benefits of local variable type inference, let’s break down some practical examples.
Example 1: Simple Variable Declaration
Using var
for clear assignments can enhance your code with better readability:
var age = 25; // clearly an int
However, caution should be exercised:
var value = someMethod(); // What is someMethod() returning?
Here, without knowledge of someMethod
, it’s effectively unclear what value
is.
Example 2: Using with Collections
Java developers often deal with Collections, which can lead to verbose declarations. Using var
simplifies this:
List<String> names = new ArrayList<>();
becomes:
var names = new ArrayList<String>();
It is clear and concise, enhancing the readability of a programmer familiar with the code.
Example 3: Complex Generics
As discussed earlier, using var
can brilliantly simplify generic types:
Map<String, List<Integer>> numberMap = new HashMap<>();
Switching to:
var numberMap = new HashMap<String, List<Integer>>();
This enhances understandability and reduces visual clutter.
Example 4: API Return Types
Let’s say you have a method returning a complex type:
public List<String> getNames() {
return Arrays.asList("Alice", "Bob", "Charlie");
}
Using var
in this context would obscure the understanding of list
:
var list = getNames(); // What did we get?
In such cases, it might be better to define the variable explicitly.
Best Practices for Using var
- Use When Types are Clear: Use
var
in cases where it is immediately evident what type you are working with. - Avoid for Public APIs: If the variable is part of an API, stay explicit to enhance clarity.
- Work with IDE Annotations: Use Integrated Development Environment (IDE) features that help you understand the types being inferred.
- Team Standards: Establish coding standards in your team to define when to use
var
.
In Conclusion, Here is What Matters
Java's local variable type inference feature undeniably positions Java among modern languages by allowing developers to write cleaner, less verbose code. But as encapsulated in the above challenges and discussions, it also necessitates careful consideration of readability, type safety, and clarity—especially in a collaborative environment.
In mastering Java's local variable type inference, developers must balance the use of var
with the fundamental principles of coding: clarity and maintainability. By adhering to best practices and understanding the implications, developers can leverage this feature effectively while minimizing its pitfalls.
For more in-depth reading on Java features and updates, you can explore the official Java Language Specification or the comprehensive Java Tutorials by Oracle.
By keeping these concepts in mind, you can master Java's local variable type inference challenges while safeguarding your code's integrity and readability. Happy coding!